There are two main types of functions in Python:
- Built-in functions: they are built-in in Python and hence they are available immediately in your Python installation. Some examples of Python built-in functions are
input()
,isinstance()
,len()
,list()
,print()
,sum()
,zip()
. - User-defined functions: they are custom functions that users create to solve specific problems that are not solved by one of the many built-in functions.
In this tutorial, we will focus on Python built-in functions.
How Many Python Built-in Functions Are Available?
Python 3.12 has 71 built-in functions. This number might change in future versions of Python.
Here are common built-in functions and examples of code that show how you can use them.
Execute the following examples using your Python shell to practice writing code in Python.
abs(): calculate the absolute value of a number
The abs()
function accepts a single argument, which can be an integer, a floating-point number, or a complex number, and returns the absolute value in the same data type for integers and floats, and the magnitude for complex numbers.
>>> abs(-10)
10
>>> abs(-4.3)
4.3
>>> abs(3 + 4j)
5.0
Understanding how to use the abs()
function is useful in calculations that require handling positive and negative numbers in the same way.
dict(): create an empty dictionary
The dict()
function in Python allows you to create dictionaries, which are data structures made of key-value pairs.
>>> values = dict()
>>> values
{}
>>> type(values)
<class 'dict'>
float(): convert a value into a floating point number
The float()
function in Python converts integer and string representations of numbers into floating-point numbers.
>>> float(10)
10.0
>>> float("10")
10.0
This function helps in scenarios where precision and decimal points are necessary, such as in scientific calculations and data analysis.
input(): take an input from the user
The input()
function in Python is used for interactive programming. It allows a program to pause and wait for user input, which can then be used dynamically within the program.
>>> number = input("Insert a number: ")
Insert a number: 25
>>> number
'25'
>>> type(number)
<class 'str'>
With this function, you can create user-friendly applications where user interaction is required, such as in simple command-line tools, basic text games, or input-driven scripts.
I have created a specific tutorial to help you learn how to use the input function in Python.
int(): convert a string into an integer
The int()
function in Python converts strings into integers. When applying int()
to strings, it’s important to ensure that the string represents a valid integer.
>>> number = int("23")
>>> number
23
>>> type(number)
<class 'int'>
Attempting to convert a non-numeric string with the int() function results in a ValueError
:
>>> int("not_a_number")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: 'not_a_number'
isinstance(): check if an object is of a specific type
The isinstance()
function in Python is a tool for type-checking. It allows developers to verify if an object (or in simpler terms a variable) belongs to a specific class.
>>> number = 23
>>> isinstance(number, int)
True
>>> isinstance(number, str)
False
>>> isinstance(number, float)
False
The isinstance()
function returns a boolean value. The boolean returned can be either True or False based on the fact that an object belongs to a specific class or not.
len(): calculate the size of data types
The len()
function in Python is a tool for determining the size or length of various data types, including strings, lists, tuples, sets, and dictionaries.
The code below shows you examples applied to those data types:
>>> animal = 'tiger'
>>> type(animal)
<class 'str'>
>>> len(animal)
5
>>> animals = ['tiger', 'lion', 'giraffe']
>>> type(animals)
<class 'list'>
>>> len(animals)
3
>>> animals = ('tiger', 'lion', 'giraffe')
>>> type(animals)
<class 'tuple'>
>>> len(animals)
3
>>> animals = {'tiger', 'lion', 'giraffe'}
>>> type(animals)
<class 'set'>
>>> len(animals)
3
>>> animal = {'name':'tiger', 'age':12}
>>> type(animal)
<class 'dict'>
>>> len(animal)
2
list(): use it to return a list
The list()
function is used for creating new lists and converting other iterable objects into lists (including tuples, strings, dictionaries, and sets).
For example, you can use list()
to convert a set into a list.
>>> numbers = {1, 2, 3, 4}
>>> list(numbers)
[1, 2, 3, 4]
>>> type(list(numbers))
<class 'list'>
max(): calculate the maximum value in a list
You can use the max()
function to find the highest value in an iterable, such as a list, a tuple, or a dictionary (for which it returns the maximum key).
>>> numbers = [1, 2, 3, 4]
>>> max(numbers)
4
>>> numbers = (1, 2, 3, 4)
>>> max(numbers)
4
>>> points = {34: 'John', 25: 'Kate', 70: 'Jane'}
>>> max(points)
70
set(): convert a list into a set
The set()
function converts lists into sets, a process that removes duplicate elements due to the nature of the set data type in Python.
>>> animals = ['tiger', 'tiger', 'lion', 'giraffe', 'lion']
>>> set(animals)
{'tiger', 'lion', 'giraffe'}
Notice that the original list contains five elements while the set contains three elements because a set cannot contain duplicates.
tuple(): convert a list into a tuple
The tuple()
function allows you to transform a list into a tuple. This can be useful when you need to create an immutable collection of elements from an existing list. Immutable means that the data structure cannot be modified.
>>> animals = ['tiger', 'lion', 'giraffe', 'lion']
>>> tuple(animals)
('tiger', 'lion', 'giraffe', 'lion')
A separate CodeFatherTech tutorial explains what is a tuple in Python.
zip(): merge two tuples
With the zip()
function you can merge two or more tuples, creating a new iterable that contains pairs of elements from the input tuples.
>>> cities = ('Rome', 'Warsaw')
>>> countries = ('Italy', 'Poland')
>>> zip(cities, countries)
<zip object at 0x7f8058083640>
>>> for value in zip(cities, countries):
... print(value)
...
('Rome', 'Italy')
('Warsaw', 'Poland')
This functionality can be handy when you need to combine related data. By using zip()
, you can avoid complex loops and achieve a more readable and concise code.
In the code above you have used a for loop in Python to go through the output returned by the zip function.
Once again, make sure to go through all the code examples for built-in functions we have covered in this tutorial to become used to writing Python code.
Related article: Now that you know about built-in functions, go through the following tutorial that will teach you about using functions to improve code reusability in Python.
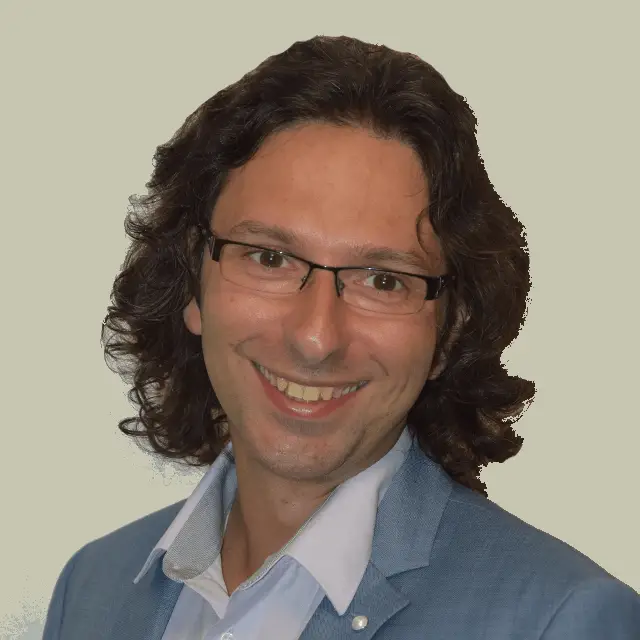
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.