The tuple is a basic Python data type. It’s important to understand it and to know how to use it. This tutorial will help you with that.
Python tuples are used to store an ordered sequence of values. Tuples are immutable, this means that the values in a tuple cannot be changed once the tuple is defined. The values in a tuple are comma-separated and they are surrounded by parentheses.
You will see some examples that clarify how you can use tuples in your programs and why.
Let’s start exploring the world of tuples!
How Do You Make a Tuple in Python?
To create a new tuple you specify a sequence of items separated by comma and surrounded by parentheses.
Here is an example of a tuple of strings:
>>> values = ('value1', 'value2', 'value3')
If you encounter an EOL SyntaxError when defining a tuple of strings make sure you haven’t missed any quotes.
>>> values = ('value1', 'value2', value3')
File "<stdin>", line 1
values = ('value1', 'value2', value3')
^
SyntaxError: EOL while scanning string literal
You can also create an empty tuple using the tuple() function.
>>> values = tuple()
>>> print(values)
()
>>> print(type(values))
<class 'tuple'>
As you will see in this tutorial you won’t be able to do much with an empty tuple… 😀
What is a Tuple in Python?
The tuple is a Python data type that can be used to store ordered sequences and it’s immutable.
For example, you could use a tuple to store names of countries.
>>> countries = ('Italy', 'United Kingdom', 'Russia', 'Poland', 'Spain')
The fact that a tuple is ordered means that we can access it by using an index that goes from zero to the size of the tuple minus one.
Retrieve the first item of a tuple
>>> print(countries[0])
Italy
Retrieve the last item of a tuple
>>> print(countries[len(countries)-1])
Spain
Notice that in the last example we have used as index len(countries)-1 where len() is a function that returns the number of items in the tuple.
Can a Tuple Have a Negative Index?
In the previous section we have seen how to use indexes to access items in a tuple.
Also…
In Python is possible to access tuple items by using negative indexes.
Here is what’s happens if I pass the index -1 to a tuple:
>>> countries = ('Italy', 'United Kingdom', 'Russia', 'Poland', 'Spain')
>>> print(countries[-1])
Spain
The index -1 in a tuple refers to the last item of the tuple.
When you decrease the value of the negative index you move in the tuple from right to left, so the index -2 would return the string ‘Poland’ and so on.
What Does Index Out of Range Mean For a Tuple?
In the previous sections we have seen how to use positive and negative indexes to access the items in a tuple.
Let’s see what happens if we use indexes that fall outside the boundaries of the tuple.
>>> countries = ('Italy', 'United Kingdom', 'Russia', 'Poland', 'Spain')
For this tuple the maximum valid positive index is 4 and it refers to the last item.
Here is what happens if we use the index 5…
>>> print(countries[5])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: tuple index out of range
Something similar also applies to negative indexes…
>>> print(countries[-6])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: tuple index out of range
The Python interpreter raises an “tuple index out of range” exception when trying to access an item in the tuple by using a positive index greater than the size of the tuple minus one. The same error also occurs when trying to access an item by using a negative index lower than the size of the tuple.
To avoid the “index out of range” error you can use the following Python for loop that handles indexes transparently for you.
>>> for country in countries:
... print(country)
...
Italy
United Kingdom
Russia
Poland
Spain
The “index out of range” exception could occur if you manually modify the index to access items of a tuple.
In that case you could introduce a bug in your code that generates a value for the index outside of the valid range.
How Do You Use a Tuple?
A tuple stores a sequence of ordered values that you can access during the execution of your program.
There are multiple things you could do with a tuple:
- Access its elements directly by using an index.
- Looping through the tuple using a for loop.
- Retrieving part of a tuple with the slice operator.
- Pass the tuple to a function for further calculations.
- Verify if a specific value is in a tuple.
We have already seen how to access individual items using an index and how to use a for loop to go through the items of a tuple.
Here is how the slice operator applies to a tuple.
>>> countries = ('Italy', 'United Kingdom', 'Russia', 'Poland', 'Spain')
Let’s say I want to retrieve the first and second items from the tuple. The slice operator allows to do that with the following syntax:
tuple[start_index:stop_index]
The start_index is included in the tuple returned while the stop_index is not included.
So, to get back the first and second items from the tuple we would use start_index = 0 and stop_index = 2.
>>> print(countries[0:2])
('Italy', 'United Kingdom')
If you omit the 0 you get the same result:
>>> print(countries[:2])
('Italy', 'United Kingdom')
And to retrieve all the items after the third (included) you can pass the following parameters to the slice operator.
>>> print(countries[2:])
('Russia', 'Poland', 'Spain')
The extended syntax for the slice operator allows to specify a step argument that allow to skip some items.
tuple[start_index:stop_index:step]
For example, to print every second item of a tuple you can use the following expression.
>>> print(countries[::2])
('Italy', 'Russia', 'Spain')
Is a Tuple Mutable or Immutable?
A tuple is immutable, this means that once created you cannot add more elements to a tuple.
Define a tuple that contains several languages:
>>> languages = ('Italian', 'English', 'Spanish', 'Polish')
Try to update the third element of this tuple…
>>> languages[2] = 'Russian'
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
When you do that you get back a TypeError exception that says that an object of type tuple does not support item assignment.
Note: we have passed the index 2 to update the third element of the tuple because Python sequences are zero-indexed (the first index is 0).
Can a Tuple Have Only One Element?
Yes, a tuple can have only one element. That’s what you call a 1-tuple.
Here is how you can define a tuple with 1 element.
>>> numbers = (1,)
>>> print(numbers)
(1,)
>>> print(type(numbers))
<class 'tuple'>
This syntax might seem a bit weird at the beginning.
Now you know that when you see parentheses and a single value followed by a comma that’s a tuple with one item.
You can access the item of a 1-tuple by using an index as usual:
>>> print(numbers[0])
1
Accessing any other index will raise a “tuple index out of range” exception.
What Does the tuple() Function Do?
As we have seen before you can use the tuple() function to create a new empty tuple.
Let’s see what happens when tuple() is applied to data structures of other types.
Apply tuple() to a list to convert it into a tuple.
>>> numbers = [1, 2, 3, 4, 5]
>>> print(tuple(numbers))
(1, 2, 3, 4, 5)
Apply tuple() to a string to convert it into a tuple of characters.
>>> day = 'Monday'
>>> print(tuple(day))
('M', 'o', 'n', 'd', 'a', 'y')
Apply tuple() to a set to convert it into a tuple.
>>> numbers = {1, 2, 3, 4, 5}
>>> print(type(numbers))
<class 'set'>
>>> print(tuple(numbers))
(1, 2, 3, 4, 5)
>>> print(type(tuple(numbers)))
<class 'tuple'>
When tuple() is applied to a dictionary it returns a tuple that contains the keys of the dictionary.
>>> values = {'a':1, 'b':2}
>>> print(tuple(values))
('a', 'b')
What is a Nested Tuple?
A nested tuple is a tuple that contains other tuples.
Let me explain it to you with an example…
The following tuple is an example of nested tuple because each item in the tuple is also a tuple.
>>> nested_values = ((1,2), (3, 4), (5, 6))
>>> print(type(nested_values))
<class 'tuple'>
>>> print(type(nested_values[0]))
<class 'tuple'>
What is the Difference Between a Tuple and a List?
The main difference between a tuple and a list in Python is that a tuple is immutable and a list is mutable.
Let’s see what this means in practice, define a list of languages.
languages = ['Italian', 'English', 'Spanish', 'Polish']
And now update the value of the third item:
>>> languages[2] = 'Russian'
>>> print(languages)
['Italian', 'English', 'Russian', 'Polish']
Our list has been updated. As we have seen before the same operation fails for a tuple and raises a TypeError exception.
Now let’s add a new item to the list using the list append() method.
>>> languages.append('Spanish')
>>> print(languages)
['Italian', 'English', 'Russian', 'Polish', 'Spanish']
We have added a new language at the end of the list.
Now, convert our list into a tuple using the tuple() “function”.
>>> languages = tuple(languages)
>>> print(languages)
('Italian', 'English', 'Russian', 'Polish', 'Spanish')
>>> print(type(languages))
<class 'tuple'>
And call the append method against the tuple to see what happens.
>>> languages.append('French')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'tuple' object has no attribute 'append'
The tuple doesn’t have an append method because a tuple cannot be changed (it’s immutable).
For the same reason you cannot remove elements from a tuple.
Why is a Tuple Better than a List?
First of all we have to clarify the scenario in which we want to use a tuple as opposed to a list.
A tuple is better than a list if you need to use a sequence you only read from and never update in your program. In that case a tuple is better because by being immutable it requires less memory than a list. This also makes a tuple faster than a list especially if it has a huge number of elements.
If you need a sequence that requires modifications during the execution of your program then you should use a list instead of a tuple.
Which Operator Can Be Used to Check if an Element is in a Tuple?
The in operator verifies if a specific value is present in a sequence. Considering that a tuple is a sequence the in operator also applies to tuples.
Define a tuple of languages…
>>> languages = ('Italian', 'English', 'Spanish', 'Polish')
Then verify if the string ‘Italian’ is in the tuple.
>>> 'Italian' in languages
True
The Python in operator returns a boolean equal to True if a given value is present in the tuple.
>>> 'Russian' in languages
False
The Python in operator returns a boolean equal to False if a given value is not present in the tuple.
You can use this in your programs together with conditional statements.
>>> if 'Italian' in languages:
... print("Language detected")
...
Language detected
How to Concatenate Two Tuples in Python
If you have two or more tuples in your Python program you might also want to concatenate them to generate a single tuple.
>>> countries1 = ('Italy', 'United Kingdom')
>>> countries2 = ('Poland', 'Spain')
To concatenate two tuples you can use the + operator.
>>> countries = countries1 + countries2
>>> print(countries)
('Italy', 'United Kingdom', 'Poland', 'Spain')
>>> print(type(countries))
<class 'tuple'>
As you can see the output of the concatenation is still a tuple.
What Types of Tuples Can You Create?
In all the examples seen so far we have worked with tuples of strings.
You can also create tuples that contain other data types.
For example, a tuple of integers…
>>> numbers = (1, 3, 5, 67, 89)
A tuple of integers and floats…
>>> numbers = (1, 3.2, 5, 67.34, 89)
A tuple of booleans…
>>> conditions = (True, False, False, True)
A tuple of lists…
>>> values = ([1, 2], [3, 4], [5, 6])
A tuple of dictionaries…
>>> values = ({'a':1}, {'b':2})
What other types of tuples could you create?
I will leave it to you to experiment with.
Conclusion
We have covered all the basics about Python tuples and by now you should be able to use tuples in your applications.
If you have any doubts about the topics we have covered I suggest to go back to the specific section and try to write the code by yourself.
Happy coding!
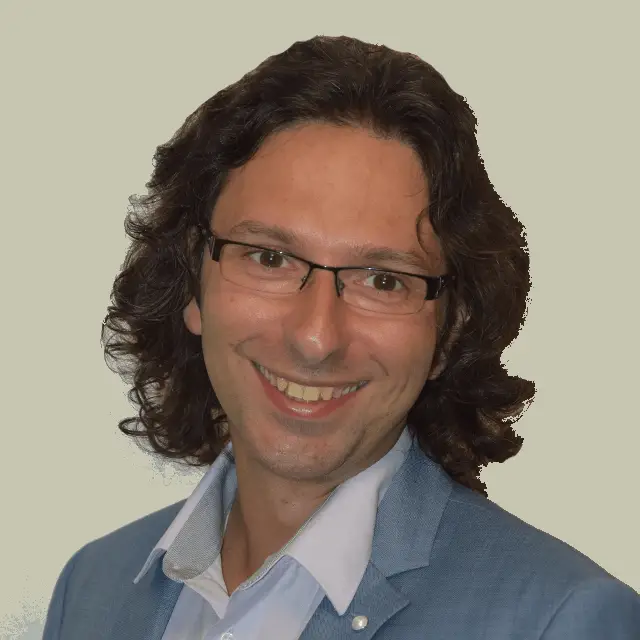
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.