I’m new to tuples in Python and I would like to know how to append elements at the end of a tuple. How can I do it?
Technically you cannot append an element to a tuple because Python tuples are immutable (they cannot be changed). But there are a few workarounds that allow you to add elements to the end of tuples.
Before learning if you can append an element to a tuple let’s have a look at what a tuple looks like:
numbers = (1, 2, 3)
If you are used to working with Python lists you might know that lists provide the append() method to add an item to the end of a list.
You might be thinking that you can use the same method to add an item to the end of a tuple.
Let’s see what happens if we try to do that:
numbers = (1, 2, 3)
numbers.append(4)
[output]
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/append_to_tuple.py", line 2, in <module>
numbers.append(4)
^^^^^^^^^^^^^^
AttributeError: 'tuple' object has no attribute 'append'
The Python interpreter tells you that tuples don’t have an append() method like lists do.
And that’s why…
A tuple is an immutable collection of elements. This means that after creating a tuple you cannot change its elements.
An important concept we will use in one of the methods covered in this tutorial is that tuples are similar to Python lists with the difference that lists are mutable and tuples are immutable.
Let’s go through a few ways to append an element to the end of a tuple.
Method 1: Use Concatenation to Append an Element to a Python Tuple
A simple way to append an element to a tuple is by concatenating the original tuple with another one containing the elements to be appended.
Here is how it works.
numbers = (1, 2, 3)
numbers = numbers + (4,)
print(numbers)
On the first line, we created a tuple called numbers containing three integers.
Then, on the second line, we used the concatenation operator (+) to concatenate the original tuple with another tuple that only contains the element we want to append to the tuple.
With the syntax (4,) we create a new single-element tuple. The trailing comma is needed for Python to recognize it as a tuple.
Below you can see the output of the print statement that shows the resulting tuple with four elements:
(1, 2, 3, 4)
Now let’s learn a more concise way to write the following line of code:
numbers = numbers + (4,)
You can also write it using the += operator:
numbers += (4,)
Verify that the result is the same before moving to the next method:
(1, 2, 3, 4)
The result is correct!
Method 2: Converting the Tuple to a List Before Appending Elements to It
Let’s look at a second approach to add elements to the end of a tuple.
A way to append elements to a tuple is to convert the tuple to a Python list, add the elements to the list, and then convert the list back to a tuple.
The following Python code shows how to implement this:
numbers = (1, 2, 3)
temp_numbers = list(numbers)
temp_numbers.append(4)
numbers = tuple(temp_numbers)
print(numbers)
On the first line of code, we create a tuple of three numbers.
Then on the second line, we convert that tuple to a list using the list() built-in function. We are doing this because lists are mutable and we can append items to a list.
We are storing this list in a temporary variable called temp_numbers. Then we use the list append() method to append a new item (an integer) to the end of the list.
The last step is to convert the temp_numbers list to a tuple using Python’s tuple() built-in function. We then assign this tuple to the numbers variable.
Execute this code and confirm that the tuple we are printing on the last line of code contains the four numbers you expect.
This method requires more lines of code compared to the first method we have covered so it’s not the best approach.
At the same time, it is an excellent way to get familiar with two Python built-in functions: list() and tuple().
Method 3: Using the Unpacking Operator to Append an Item to a Tuple
You can use the Python unpacking operator (*) to unpack elements in a tuple. Using the unpacking operator also allows you to append elements to a given tuple.
Before writing the code let’s see how the unpacking operator works with a tuple using the Python shell:
>>> numbers = (1, 2, 3)
>>> (*numbers,)
(1, 2, 3)
Now let’s write some code to see the unpacking operator for a tuple in action!
numbers = (1, 2, 3)
updated_numbers = (*numbers, 4)
print(updated_numbers)
With this Pythonic approach, we create a new tuple called updated_numbers by unpacking the three integers of the existing tuple followed by the additional integer we want to append to the tuple.
This method is as concise as the first one considering that it only needs one single line of Python code.
BONUS: Appending Another Tuple as an Element to an Existing Tuple
The element you append to an existing tuple can also be another tuple. This way you obtain what’s called a nested tuple.
Here is an example of what this looks like.
numbers = (1, 2, 3)
updated_numbers = numbers + ((4, 5),)
print(updated_numbers)
As you can see, on the second line we are using the concatenation operator (+) to append a new tuple as an element.
Notice that it’s still important to specify the comma after the tuple ((4, 5),) otherwise, Python won’t recognize this as a tuple.
Below you can see the resulting tuple:
(1, 2, 3, (4, 5))
The fourth element of the final tuple is a tuple that contains two integers: (4, 5).
Conclusion
Now you know three different ways to append an element to a tuple in Python and you will be able to use them if you need them in your future programs. This will speed up the process of writing your code.
The two best approaches are the ones that use the concatenation operator and the unpacking operator.
Converting the tuple into a list and then back to a tuple after appending the elements, requires more lines of code. That’s why this is not the ideal approach.
You have also learned that you can obtain a nested tuple by appending another tuple as an element of an existing tuple.
Related searches: To have a more in-depth understanding of Python data types and tuples in particular, explore the CodeFatherTech guide about Python tuples.
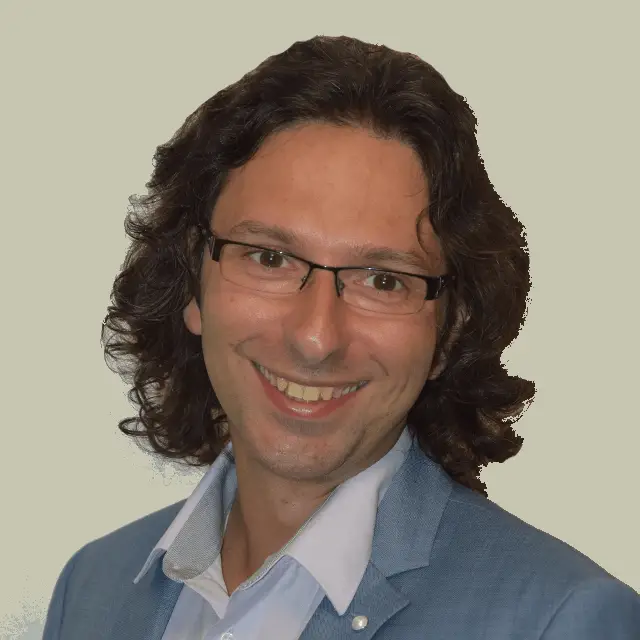
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.