Being able to add items to a list is a routine task for Python developers. Learn multiple ways to do that so you can write programs faster.
Python provides multiple ways to add an item to a list. Traditional ways are the append(), extend(), and insert() methods. The best method to choose depends on two factors: the number of items to add (one or many) and where you want to add them to the list (at the end or at a specific location/index).
By the end of this article, adding items to a Python list will be one of your skills.
Let’s dive in!
Add One Item to a List in Python Using the append() Method
Once you start writing Python code you will quickly realize that lists are one of the most commonly used data structures in Python.
The most basic way to add an item to a list in Python is by using the list append() method. A method is a function that you can call on a given Python object (e.g. a list) using the dot notation.
Create a list of strings that contains the names of three cities. You will use the append() method to add a fourth string to the list:
cities = ["London", "New York", "Rome"]
cities.append("Paris")
print(cities)
You can see that to call the append() method we have specified the name of the list (cities) followed by a dot and then followed by the append method.
We have passed the item to be added to the list to the append method.
Here is what the list looks like after appending the new item to it:
['London', 'New York', 'Rome', 'Paris']
Notice that the last item we have added to the list appears at the end of the list. That’s because Python lists are ordered data structures.
In other words…
The list append() method adds a single item to the end of a list in Python.
Can You Add Multiple Items to a Python List Using the append() Method?
How can you add several items to a Python list using the append() method?
Let’s take the previous example and try adding another string when calling the append() method.
cities = ["London", "New York", "Rome"]
cities.append("Paris", "Warsaw")
When you execute this code you will see the following exception:
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/add_item_to_list.py", line 2, in <module>
cities.append("Paris", "Warsaw")
TypeError: list.append() takes exactly one argument (2 given)
The Python interpreter raises a TypeError because the list append() method only takes one argument while in our code we have passed two strings to it.
So, what’s the solution? Can we pass a list to the append method instead?
Let’s try!
cities = ["London", "New York", "Rome"]
cities.append(["Paris", "Warsaw"])
print(cities)
Hmmm…
It doesn’t work either because the result is a nested list inside the original list (see the square brackets inside the original list).
['London', 'New York', 'Rome', ['Paris', 'Warsaw']]
You cannot add multiple items to a Python list using the append() method. To add multiple items to a Python list you have to use the extend() method.
Using the extend() Method to Add Multiple Items to a Python List
To add multiple items to a Python list, use the list extend() method. The items can come from another list or any iterable.
Let’s take the previous example and try to add two strings to the initial list of strings using the extend() method.
cities = ["London", "New York", "Rome"]
cities.extend(["Paris", "Warsaw"])
print(cities)
We passed a list to the extend() method and we expect to see a final list of strings that contains five items.
Let’s see what the output of the print function is:
['London', 'New York', 'Rome', 'Paris', 'Warsaw']
The output is correct, the extend() method does what we expected. It adds the new items after the items in the original list.
If instead of passing a list you pass a tuple to the extend() method, the result is the same:
cities = ["London", "New York", "Rome"]
cities.extend(("Paris", "Warsaw"))
print(cities)
[output]
['London', 'New York', 'Rome', 'Paris', 'Warsaw']
Adding Item to a Python List Using the insert() Method
If you want to add an item to a list at a specific position, you can use the list insert() method.
Here is the syntax of the insert() method:
list.insert(i, x)
Where the first argument (i) is the index of the item before which to insert the new item and the second argument (x) is the item to insert.
Let’s take the previous list as an example and see how the insert() method works.
cities = ["London", "New York", "Rome"]
If you want to add an item before the second item in the list (“New York”) you will use the following syntax:
cities.insert(1, "Paris")
print(cities)
[output]
['London', 'Paris', 'New York', 'Rome']
We are using index 1 because it’s the index of the second item in the list. Remember that list indices start from zero.
To insert an item as first in a list using the insert() method, you can use the index with value zero.
cities.insert(0, "Paris")
print(cities)
[output]
['Paris', 'London', 'New York', 'Rome']
To insert an item as last in a list using the insert() method, you can use the index with value len(list).
cities.insert(len(cities), "Paris")
print(cities)
[output]
['London', 'New York', 'Rome', 'Paris']
In this last scenario, the insert() method behaves like the append() method by adding the item at the end of the list.
How to Add Items to a List Using the + Operator
The + operator can be used to add items to a list in Python. It effectively concatenates one list to another (list concatenation).
Let’s start with the cities list and try to add the elements in the list new_cities to it using the + operator.
cities = ["London", "New York", "Rome"]
new_cities = ["Paris", "Warsaw"]
cities = cities + new_cities
print(cities)
Below you can see the new value of the list cities:
['London', 'New York', 'Rome', 'Paris', 'Warsaw']
When using the + operator both variables have to be Python lists. Here is what happens if you try to use the + operator between one list and one string:
cities = ["London", "New York", "Rome"]
new_city = "Paris"
cities = cities + new_city
When you execute this code, Python raises a TypeError because you can only concatenate a list to a list and not a list to a string.
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/add_item_to_list.py", line 3, in <module>
cities = cities + new_city
~~~~~~~^~~~~~~~~~
TypeError: can only concatenate list (not "str") to list
Makes sense?
Adding Elements to a List Using the * Unpacking Operator
Using the Python unpacking operator you can easily merge two or more lists. In other words, you can add the items of a list to another list.
cities = ["London", "New York", "Rome"]
new_cities = ["Paris", "Warsaw"]
cities = [*cities, *new_cities]
print(cities)
The * symbol unpacks the items in the lists and groups them in the existing list.
You can see how this works for a single list in the example below executed in a Python shell:
>>> [*cities]
['London', 'New York', 'Rome']
Learn more about unpacking a list in Python.
Conclusion
Adding items to a list in Python is straightforward. As you have seen in the examples covered in this tutorial, you have multiple ways to do it.
The approach you will choose depends on two factors:
- If you are adding a single or multiple items to a list.
- Where you are adding the item to the list (at the end or at a specific location).
Related article: Explore other methods provided by Python lists by going through the CodeFatherTech tutorial about Python list methods.
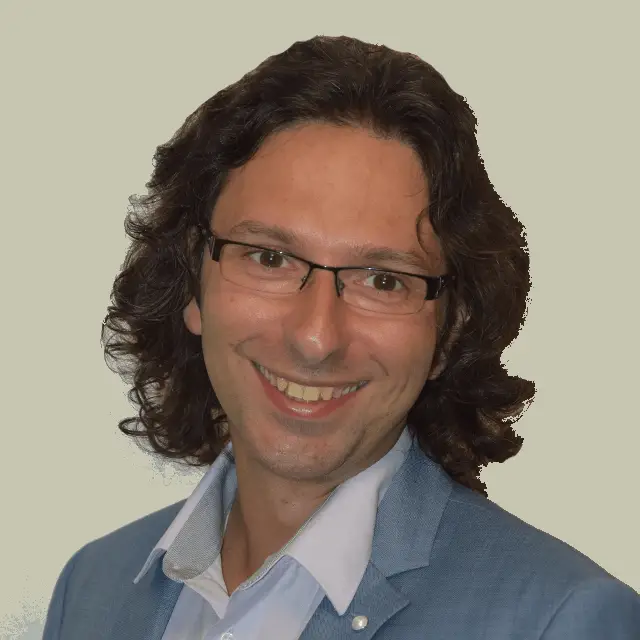
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.