At some point in your career as a developer, you will need to apply a function to a list in Python. What options do you have to do that?
Python provides multiple ways to apply a function to all the elements in a list. The most basic one is using a for loop that applies the function one element at a time. There are also more Pythonic ways to apply a function to the elements of a list: using a map function, list comprehension, lambda function, or generator expression.
In this tutorial, we will use the same list as a starting point and apply each method to generate a new list. This will allow you to decide which approach you prefer.
Let’s get started!
How Do You Apply a Function to Every Element in a Python List?
There are multiple ways to apply a function to every list element using Python. Here are some options to do that:
- Map Function
- List Comprehension
- Lambda Function
- For loop
- Generator expression
- NumPy array
Two factors in choosing the best approach for you are the number of lines of code and the readability of the code.
In each one of the examples we will start with the following list of numbers:
numbers = [34, 67, 5, 21, 7]
We will see how to create a new list in which every element is the cube of the respective element in the original list.
Method 1: Use the Map Function
In its basic form, Python’s map() built-in function takes two arguments: a function and an iterable. This allows you to apply the function passed as the first argument to each element of the iterable.
Let’s see how this works with our list of numbers.
First of all, you have to define the function to be passed to the map() function. As we said before we want to calculate the cube of each number in the original list.
def cube(x):
return pow(x,3)
In this example, we have defined a function called cube(). The pow() function returns x to the power of 3.
Now call the map() function by passing the cube() function and the numbers list to it:
cube_numbers = map(cube, numbers)
print(cube_numbers)
When you print the content of the variable cube_numbers you will see an output similar to the one below:
<map object at 0x10340eef0>
Interestingly, we are not seeing a list of numbers. We are getting back a map object instead.
To get back a list of numbers we have to apply the list() function to the value returned by the map function.
Let’s do it!
cube_numbers = list(map(cube, numbers))
print(cube_numbers)
This time we can see the new list in which each element is the cube of each number in the numbers list.
[39304, 300763, 125, 9261, 343]
Using the map() built-in function you can apply a function to all elements in a list using just one line of Python code.
Method 2: Use a List Comprehension
A powerful construct in Python is the list comprehension which allows working with lists in a single line of code.
Using a list comprehension we will apply our custom function to every element of the list of numbers.
def cube(x):
return pow(x,3)
cube_numbers = [cube(number) for number in numbers]
print(cube_numbers)
Confirm that the output is correct:
[39304, 300763, 125, 9261, 343]
To use a list comprehension you have to open and close square brackets. Then inside the square brackets, you call the function to apply to each element followed by the for…in syntax that goes through each element in the list.
Notice that the list comprehension creates a new list. In this case cube_numbers.
Pretty simple and effective!
Method 3: Use a Lambda Function
Instead of defining the cube() function using the def keyword, you can use a lambda function instead. Here is how the cube() function becomes when using a lambda.
We will test it in a Python shell first:
>>> lambda x: pow(x,3)
<function <lambda> at 0x102fabf60>
>>> (lambda x: pow(x,3))(3)
27
Now you can pass the lambda function to the map() built-in function. This is a way to apply lambda functions to each element in the list:
cube_numbers = list(map(lambda x: pow(x,3), numbers))
print(cube_numbers)
Once again the output is correct:
[39304, 300763, 125, 9261, 343]
This expression is a bit more complex than the previous ones considering that to understand it you need to have clear in your mind how map() and lambda() work.
Also, this might make it harder to read to other developers who have to maintain your code, depending on their Python knowledge.
To learn more about lambdas read the CodeFatherTech tutorial about lambda functions in Python.
Method 4: Use a For Loop
The approach based on a for loop is the most basic one. Understanding how a Python for loop works is a must-have skill for you as a Python developer.
With a for loop you can iterate through the elements of the original list, apply the function to each element, and then append the result to a new list.
def cube(x):
return pow(x,3)
cube_numbers = []
for number in numbers:
cube_number = cube(number)
cube_numbers.append(cube_number)
print(cube_numbers)
First of all, outside of the for loop, we create an empty list called cube_numbers that will contain the cube of each element in the original list.
Then you use a for loop to go through each number in the numbers list. Apply the cube() function to each number and then use the list append() method to add the cube of the number to the new list.
Execute this code and verify that the output is correct.
As you can see, this approach is the one that requires the most lines of code compared to the other ones we have explained before.
We could reduce the lines of code in the for loop by calling the cube() function and the list append() method in the same line of code.
cube_numbers = []
for number in numbers:
cube_numbers.append(cube(number))
This method based on a for loop is potentially the simplest approach to understand because it just requires basic knowledge about for loops in Python.
Method 5: Use a Generator Expression
Generator expressions are a more advanced concept in Python and they have some similarities with list comprehensions.
The main difference is that a generator expression yields one item at a time. This makes it memory efficient.
To convert a list comprehension into a generator expression replace the enclosing square brackets with parentheses.
def cube(x):
return pow(x,3)
cube_numbers = (cube(number) for number in numbers)
print(cube_numbers)
Here is what the generator expression returns:
<generator object <genexpr> at 0x10423cba0>
The generator expression returns a generator object. To get back a list instead, you have to pass the output of the generator expression to the list() function.
This is similar to what we have done with the map() function in one of the previous sections.
cube_numbers = list(cube(number) for number in numbers)
print(cube_numbers)
Et voilà, we get the expected output:
[39304, 300763, 125, 9261, 343]
Method 6: Use a NumPy Array
The last method we will go through uses the NumPy module and to be more precise the concept of array in NumPy.
When you need to execute mathematical operations in Python, you can use the NumPy module that allows you to execute operations on arrays of data without using for loops.
First of all, we have to import the NumPy module and replace the original list with a NumPy array.
import numpy as np
numbers = np.array([34, 67, 5, 21, 7])
Then you can use a single line of code to apply the cube() function to each element of the NumPy array.
def cube(x):
return pow(x,3)
cube_numbers = cube(numbers)
print(cube_numbers)
You will notice that the output is slightly different from the output in the previous examples considering that we are working with a NumPy array instead of a list.
[ 39304 300763 125 9261 343]
Conclusion
Well done for getting to the end of this tutorial!
You now know 6 different methods in Python to apply functions to each element of a list and you can pick the one you prefer.
Remember to make your code simple and keep in mind that other developers will have to understand it in the future.
Related article: And now, to continue growing your Python knowledge have a look at the CodeFatherTech tutorial about Python functions.
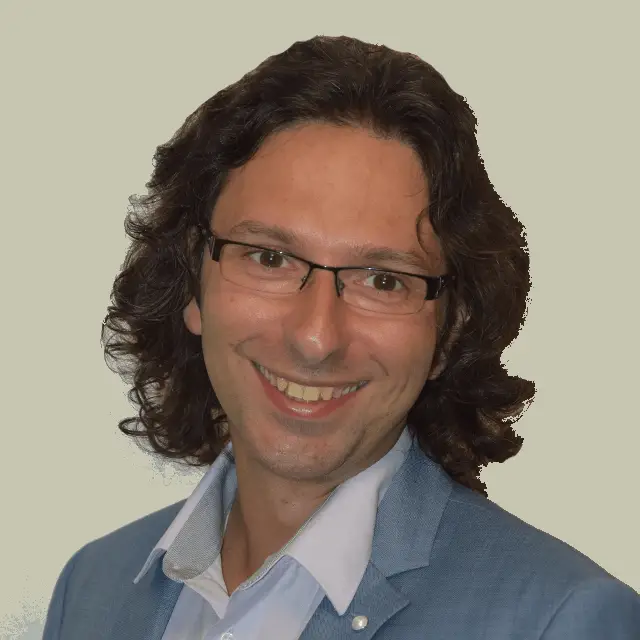
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.