Disclosure: Some of the links and banners on this page may be affiliate links, which can provide compensation to CodeFatherTech (https://codefather.tech) at no extra cost to you. CodeFatherTech is a participant in the Amazon Services LLC Associates Program, an affiliate advertising program designed to provide a means for sites to earn fees by linking to Amazon.com and affiliated sites. CodeFatherTech also participates in affiliate programs from DataCamp, Coursera, and other sites. Our affiliate disclaimer is available here.
Do you know that when you define a Python function you can set a default value for its parameters? This tutorial will show you how to do it.
Generally, when calling a Python function you provide arguments for all the parameters defined in the function. When you use default parameters you give default values to specific parameters defined in a function. If a value is passed for that parameter when calling the function that value is assigned to the parameter otherwise the parameter keeps its default value.
In this tutorial, we will first see how to use default parameters and then you will understand in which scenario you would use them.
Let’s see a few examples!
How to Add a Default Parameter to a Python Function
Before starting I want to remind you of the difference between parameters and arguments.
Very often the difference between these two concepts is not very clear to developers…
Parameters are the variables listed in the definition of a Python function within parentheses. Arguments are the variables you pass when calling a function (again within parentheses). The values of arguments are assigned to the parameters of a function.
Let’s define a Python function that calculates the sum of two numbers.
def sum(a, b):
return a + b
When we call this function we have to pass two arguments:
print(sum(1, 2))
The output is:
$ python default_parameters.py
3
If you only pass one argument…
print(sum(1))
…you get the following error because the Python interpreter is expecting two arguments:
$ python default_parameters.py
Traceback (most recent call last):
File "default_parameters.py", line 4, in <module>
print(sum(1))
TypeError: sum() missing 1 required positional argument: 'b'
To set a default parameter in our function we use the equal sign followed by a value next to the parameter for which we want a default value.
For example, let’s set a default value for the parameter b.
def sum(a, b=1):
return a + b
The parameter b has now a default value of 1.
And if we call the function with one argument we don’t see an error anymore.
print(sum(1))
Output:
$ python default_parameters.py
2
When we call the function using sum(1) the argument 1 gets passed to the first parameter a and the value of b is the default value 1.
Can You Use a Default Value for the First Parameter in a Python Function?
Let’s see what happens when we set a default value just for the first parameter of the function: the parameter a.
The function becomes…
def sum(a=1, b):
return a + b
Note: if you try to make this code change in your IDE you will notice immediately that your IDE will display a syntax error.
And here is what we get back when we call the function using print(sum(1)).
$ python default_parameters.py
File "default_parameters.py", line 1
def sum(a=1, b):
^
SyntaxError: non-default argument follows default argument
Hmmm, we get back an error…
But, why?!?
Actually, the error is not caused by the function call, as mentioned before it’s a syntax error.
The problem here is that an argument without a default value, b, follows an argument that has a default value a.
Let’s find out more about this error.
SyntaxError: non-default argument follows default argument. What Does it Mean?
The message in this error it’s clear…
It’s complaining about the fact that an argument that doesn’t have a default value follows an argument that has a default value.
To solve this error make sure non-default arguments (or parameters) appear before default arguments (or parameters).
In a Python function parameters with default values always have to follow parameters that don’t have a default value.
Can You Pass a Value to a Default Parameter in Python?
The answer is yes.
Even if you define a default parameter in a Python function you can always pass a value to it when you call the function. When you pass a value to it that value replaces the default value of the parameter.
For example, in our function we assign the default value 1 to the parameter b:
def sum(a, b=1):
print("The value of a is", a)
print("The value of b is", b)
return a + b
In this function, we use the Python print function to show the value of the two parameters.
This is an example of how a Python function can call another function (print is a Python built-in function).
Then when calling the sum function we pass two values:
print(sum(3, 2))
- The value 3 is assigned to the parameter a.
- The value 2 is assigned to the parameter b (the default value of b is not used).
And the output is…
$ python default_parameters.py
The value of a is 3
The value of b is 2
5
How to Assign Default Values to Multiple Parameters in a Python Function
Let’s see how to define multiple parameters that have a default value.
Increase the number of parameters in the definition of the sum function and assign a default value to the last three parameters.
def sum(a, b, c=1, d=2, e=3):
print("The value of a is", a)
print("The value of b is", b)
print("The value of c is", c)
print("The value of d is", d)
print("The value of e is", e)
return a + b + c + d + e
Then call the function…
print(sum(3, 2))
What result would you expect?
I’d say 11, let’s find out…
$ python default_parameters.py
The value of a is 3
The value of b is 2
The value of c is 1
The value of d is 2
The value of e is 3
11
That’s correct!
As you can see we have passed values to the parameters a and b. In the calculation of the sum, the function has used the default values for the other three parameters c, d, and e.
How to Pass a Value to a Default Parameter When Multiple Default Parameters are Defined
Now, let’s say that when calling the sum() function we want to pass arguments for the parameters a, b, and d.
How can we do that?
If we simply call the function this way…
print(sum(3, 2, 6))
The value 6 is assigned to the parameter c.
$ python3 default_parameters.py
The value of a is 3
The value of b is 2
The value of c is 6
The value of d is 2
The value of e is 3
16
How can you assign 6 to the parameter d instead?
You can specify the name of the parameter when calling the function…
print(sum(3, 2, d=6))
With the latest code the output is…
$ python3 default_parameters.py
The value of a is 3
The value of b is 2
The value of c is 1
The value of d is 6
The value of e is 3
15
As you can see this time the value 6 has been assigned to the parameter d. And the parameter c still has its default value 1.
Why Do You Use Default Parameters in a Python Function?
So far we have seen how to use default parameters in a Python function.
But…
We haven’t really explained why and in which scenario you would use default parameters.
Here is an example…
I want to create a Python function that describes a house. Let’s say there are only certain aspects we want to customize in the house.
For example, we want to customize the number of bedrooms but we are fine with the default color of the main door.
We would create the following function…
def describe_house(bedrooms, door_colour="red"):
print(f"This is a {bedrooms} bedroom house with a {door_colour} door")
When we call this function we only have to pass the bedrooms argument.
describe_house(3)
And the output is…
This is a 3 bedroom house with a red door
If we want to change the color of the door we can pass a different value for the default parameter door_colour.
describe_house(3, door_colour="blue")
This time the output of the function is…
This is a 3 bedroom house with a blue door
Makes sense?
Conclusion
In this tutorial, we have learned together how default function parameters work in Python and how you can use them in your code.
You have seen that the order of parameters with and without a default value is important in the definition of a function.
You have also learned that when calling a function you can specify values that replace the default value for parameters.
Knowing about default parameters will make your code more flexible so start thinking about where in your code you could start using default parameters.
Have you enjoyed this tutorial? Are you wondering what to create with Python next?
DataCamp has designed a course that teaches Data Science in Python, which is becoming extremely popular.
Discover the DataCamp course Introduction to Data Science in Python today.
Happy coding!
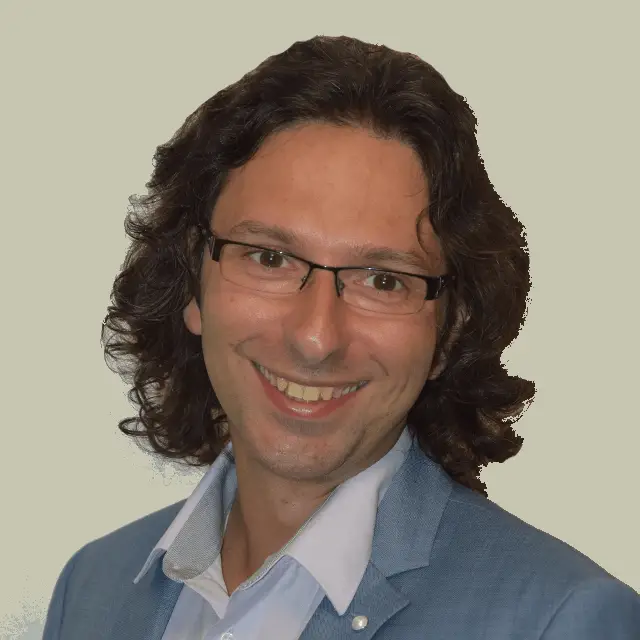
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.