I have written a Python function that creates a list. How can the function return the list so I can use it in other parts of my program?
To return a list from a function in Python, use the return keyword followed by the list you want to return. The approach is the same as if you were returning any variable from a function. The code calling the function will then be able to use the list returned by the function.
Here is a simple example of a function that returns a list:
def get_numbers():
numbers = [4, 32, 109, 23, 1056]
return numbers
result = get_numbers()
print(result)
# [4, 32, 109, 23, 1056]
In this code snippet, first define a function called get_numbers()
using the def keyword. This function creates a list called numbers
and then returns it using the return
keyword on the last line of the function.
Store the return value of the function in the variable result
, then pass this variable to the print()
function that prints the list returned by the function.
This is a basic example of how to return a list from a function in Python.
How do I return multiple lists from a function in Python?
A Python function can return multiple lists. To do that you have to pass the lists to the return statement of the function using the comma as a separator.
Let’s see how this works with an example of code:
def get_numbers_and_words():
numbers = [4, 32, 109, 23, 1056]
words = ['learn', 'program', 'beginner', 'concepts', 'coding']
return numbers, words
The last line of the function get_numbers_and_words()
returns two lists, the first one that contains numbers (integers) and the second one that contains words (strings). Notice that the two lists are comma-separated.
What data type do you think is returned by the function?
You can find this out using the type() built-in function.
data = get_numbers_and_words()
print(type(data))
# <class 'tuple'>
You can see that the function above returns a tuple. The two lists returned by the function are inside the tuple.
Let’s confirm it by printing the value of the variable returned by the get_numbers_and_words()
function:
data = get_numbers_and_words()
print(data)
# ([4, 32, 109, 23, 1056], ['learn', 'program', 'beginner', 'concepts', 'coding'])
You can see that the function returns a tuple in which the first element is the list of integers (numbers) and the second element is the list of strings (words) returned by the function.
You can access each list in the tuple by using indexing. Below we access the first and second elements (both lists) in the tuple:
First element
data = get_numbers_and_words()
print(data[0])
# [4, 32, 109, 23, 1056]
Second element
data = get_numbers_and_words()
print(data[1])
# ['learn', 'program', 'beginner', 'concepts', 'coding']
Remember that sequence indexing in Python starts from zero.
How can I return a list created using a list comprehension?
In Python, you can build a list in a function using a list comprehension and then return that list with the return keyword.
Let’s write a function that returns a list of numbers from 0 to 10 using a list comprehension and the range() function:
def get_numbers():
return [x for x in range(1023, 1043)]
Now call the function and confirm that it returns the correct list:
numbers = get_numbers()
print(numbers)
# [1023, 1024, 1025, 1026, 1027, 1028, 1029, 1030, 1031, 1032, 1033, 1034, 1035, 1036, 1037, 1038, 1039, 1040, 1041, 1042]
In the last snippet of Python code, you called the get_numbers()
function and stored the list returned by the function in the numbers
variable.
How do you verify if a function returns a list?
When you write real programs you could end up with complex functions in which it’s not so straightforward to see if the object you are returning in a function is a list.
To confirm that a Python function returns a list, you can use Python's type() built-in function. This function shows the data type of the variable you are returning and confirms if that's a list.
Here is an example:
def get_words():
message = "Write one Python program every day"
words = message.split()
print(type(words))
return words
We are creating a list from a string using the string split() method.
Before returning this list we are using the type() function to print the data type of the variable words
.
words = get_words()
# <class 'list'>
The output confirms that the variable returned by the function get_words()
is a list.
You can use the print()
function to see the content of the list the function returns:
print(words)
# ['Write', 'one', 'Python', 'program', 'every', 'day']
You can see that this list is the result of splitting the initial string using the space as a separator.
With this approach, the function can take a text of any length and return a list in which every element is one word of the text.
You can then write additional logic in your code to analyze the text based on your objective (e.g. finding the words that appear more often in the text).
Related article: Continue building your knowledge by going through the step-by-step CodeFatherTech tutorial about Python functions.
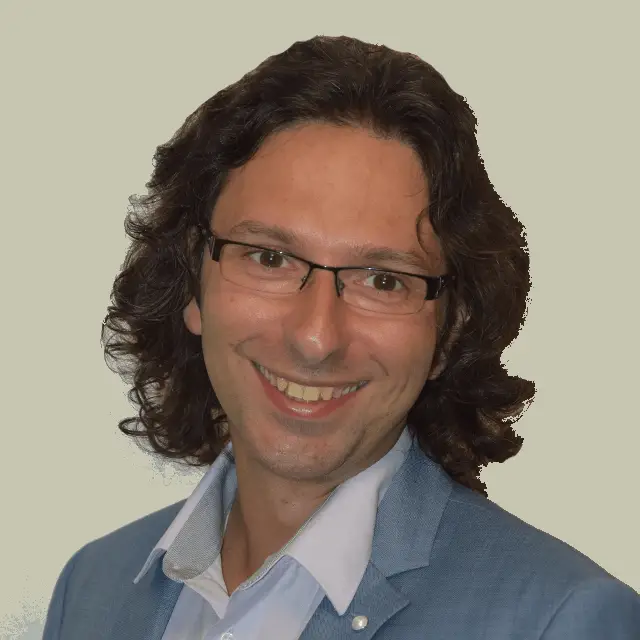
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.