I have written this article to help you understand how functions can make your life easier when writing a program in Python.
The concept of function in Python is the same as in many other programming languages.
Functions allow you to organize code in modular blocks and make code reusable. The more your Python code grows the more difficult it can get to manage it if you don't use functions. Once you define a function you can call it multiple times in your code without having to rewrite that code again (avoid code duplication).
Below you can see the full syntax of a function:
def function_name(parameters):
"""docstring"""
function_body
The components used to define a Python function are:
- header: this is made of the
def
keyword that starts the definition of the function, the function name, parameters enclosed within parentheses, and the colon symbol. Parameters are optional, a function can also have no parameters. Also, a function can have any number of parameters. - docstring: provides documentation about the function.
- body: this is a sequence of Python statements and can end with an optional
return
statement if the function returns a value.
I have written an example of function that accepts a single parameter and prints a message that depends on the value passed when calling the function.
def say_hello(name):
print("Hello " + name)
The name of the function is say_hello()
and it accepts one parameter called name
.
The function executes a single print statement to print the word “Hello” concatenated to the value of the parameter passed to the function.
To call a function in Python specify the name of the function followed by parentheses. Within parentheses, provide any values to be passed to the function based on the parameters in the function header. The values passed to parameters when you call a function are called arguments.
say_hello("Codefather")
# Hello Codefather
A Visual Representation of the Way a Function Works
We have seen how to define a function and how to call it.
The following diagram shows how a function works during the execution of a program.
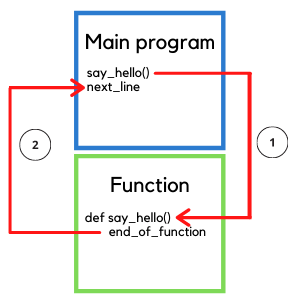
The Python program is executed line by line until the call to the function is encountered, in this case, say_hello()
.
At that point, the execution of the main program jumps to the function and goes through all the lines of code in the function until the function ends or a return
statement is found.
Then the execution of the program continues from the next line after the function call and it continues until the last line of the main program.
How to Define a Function using the def Keyword
In the previous example, you created a function containing a single line of code.
A function can have multiple lines of code and every line in the function body must have the same indentation. If the indentation of lines within a function is not the same the Python interpreter raises a syntax error.
I will update the previous function to print today’s date using the datetime module:
from datetime import date
def say_hello(name):
today = str(date.today())
message = "Hello " + name + ". Today is " + today
print(message)
The first line of the function retrieves today’s date and converts it into a string. The second line of the function creates the message to be printed by concatenating multiple strings.
The function prints the following message when you call it (see the code on the third line of the function).
Hello Codefather. Today is 2021-07-31
If you forget to convert today’s date into a string you will get the following TypeError
exception:
Traceback (most recent call last):
File "functions.py", line 9, in <module>
say_hello("Codefather")
File "functions.py", line 5, in say_hello
message = "Hello " + name + ". Today is " + today
TypeError: can only concatenate str (not "datetime.date") to str
Notice that the three lines in the function use the same indentation.
Let’s modify one of the lines in the function to use an incorrect indentation.
def say_hello(name):
today = str(date.today())
message = "Hello " + name + ". Today is " + today
print(message)
And see what happens:
File "functions.py", line 6
print(message)
^
IndentationError: unexpected indent
When the indentation of one or more lines in a function is incorrect, the Python interpreter raises an IndentationError exception.
What is the keyword “return” in a Python function?
In the function we have seen so far, the code in the function prints a message.
The most common approach is for a function to return one or more values to the caller (the line of code where the function is called).
To explain this concept I will create a program that calculates the sum of two numbers. Without using a function I write the following code:
number1 = 10
number2 = 15
result = number1 + number2
print("The sum of the two numbers is " + str(result))
What if we want to write a function we can reuse in the future when we want to calculate the sum of two numbers?
We can add a return statement to it:
def calculate_sum(a, b):
result = a + b
return result
What does the return statement do in Python?
As you can see, in the function above we:
- take two parameters
a
andb
. - calculate the sum of the two parameters and store it in the variable
result
. - use the
return
keyword to return theresult
of the sum.
This allows you to use the return value in the part of the program that calls the function.
number1 = 10
number2 = 15
print("The sum of the two numbers is " + str(calculate_sum(number1, number2)))
Can you see that this time in the print statement we call the function calculate_sum()
?
We can do that because the function returns the sum using the return statement.
Continue building your knowledge about functions with the following tutorials:
- Working with built-in functions in Python
- Understand the difference between function parameters and arguments
If you have any questions feel free to email me at hello@codefather.tech.
To help you learn Python I created a tutorial on how to start programming in Python.
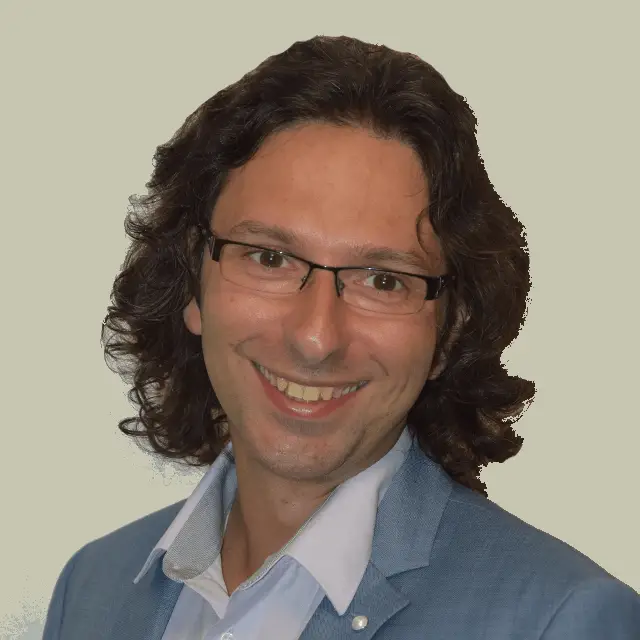
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.