To check if a list contains an item in Python, you can use the “in operator”. This operator returns True if the element exists in the list and False otherwise. An alternative is to use the list count() method.
Note: The terms item and element of a list used in this article have the same meaning.
Using the “in operator” Python finds out if an item is in a list or not. It returns True if the item is in the list and False if the item is not present in the list.
I will open the Python shell to show how the “in operator” works:
>>> fruits = ['orange', 'apple', 'banana', 'orange']
>>> 'orange' in fruits
True
>>> 'pineapple' in fruits
False
The “in operator” returns True when you check if the list contains the string ‘orange’ because that string exists in the list.
In the same way, the “in operator” returns False when you check if the string ‘pineapple’ is in the list considering that this string doesn’t appear in the list.
The values True and False are booleans. I have another step-by-step tutorial if you want to learn about Python booleans.
How do I check if an item is not in a list using Python?
The approach you can use to check if an element is not present in a list using Python is the opposite of the one we have seen in the previous section.
To check if an item is not in a list using Python you can use the “not in” operator that executes the opposite check compared to the “in” operator. The “not in” operator returns True if an item is not in the list and returns False if the item exists in the list.
Let’s see how this works using the Python shell.
>>> fruits = ['orange', 'apple', 'banana', 'orange']
>>> 'orange' not in fruits
False
>>> 'pineapple' not in fruits
True
As you can see in this example, when you check if the string ‘orange’ is not in the list, you get back a False boolean. That’s because the string ‘orange’ element exists in the list.
When you check if the string ‘pineapple’ is not in the list, Python returns True. That’s because the string ‘pineapple’ doesn’t exist in the list.
How to check if a Python list contains an item using an if statement
In a Python program, different logic might be required depending on whether a specific item in the list exists.
Imagine you want to check whether a given item is in a list, and based on that your program has to execute different actions.
The list we will use in the following example represents an inventory of products. The if statement checks if a new product we want to add to the inventory is already present in the inventory or not.
Here is what the Python code to do that would look like:
products = ['chair', 'table', 'lamp']
new_product = 'coffee mug'
if new_product in products:
print(f"The {new_product} is already in the inventory.")
else:
print(f"The {new_product} is not in the inventory and it will be added to it.")
First of all, create the list products
that contains the current inventory of products.
Then assign the value ‘coffee mug’ to the string variable new_product
. This is just an example, in a real-world program this value would come from the user, for example by using the input() function.
The next step involves using an if statement to verify if new_product
is already in the list products
. To do that we are using the “in operator” explained previously.
Then you print a message that varies based on whether the new_product
is in the list. The print statements in the if and else blocks use Python F-strings.
You can enhance the code by adding the new_product
to the products
list if that element is not in the list.
Here is how the body of the if statement changes:
if new_product in products:
print(f"The {new_product} is already in the inventory.")
else:
print(f"The {new_product} is not in the inventory and it will be added to it.")
products.append(new_product)
print(f"The updated inventory is {products}")
If the new_product
is not in the list of products you use the list append() method to add it to the list. Then you print the updated list of products.
Using the list count() method to check if an item exists in a list
The list count() method can be used to check if an element is in a Python list because the count() method returns the number of times a given element appears in a list. An element is in a list if the value returned by the count() method is greater than 0.
Let’s start with an example using the Python shell:
>>> fruits = ['orange', 'apple', 'banana', 'orange']
>>> fruits.count('orange')
2
>>> fruits.count('pineapple')
0
In the example above you can see something important: One difference between using the “in operator” and the count() method is that the latter can also tell if an element appears more than once in the list.
Here is how you can use the list count() method as part of an if / else statement:
products = ['chair', 'table', 'lamp']
new_product = 'coffee mug'
if products.count(new_product) > 0:
print(f"The {new_product} is already in the inventory.")
else:
print(f"The {new_product} is not in the inventory and it will be added to it.")
products.append(new_product)
print(f"The updated inventory is {products}")
The count() method can be useful because it tells how many times an item appears in a list.
But there is something else to consider…
The count() method is less efficient than the “in operator”. That’s because the “in operator” stops searching when it finds the item while the count() method scans the entire list to count occurrences. This can become time-intensive for large lists.
Related article: Python lists provide more methods that are explained in the following CodeFatherTech tutorial about Python list methods.
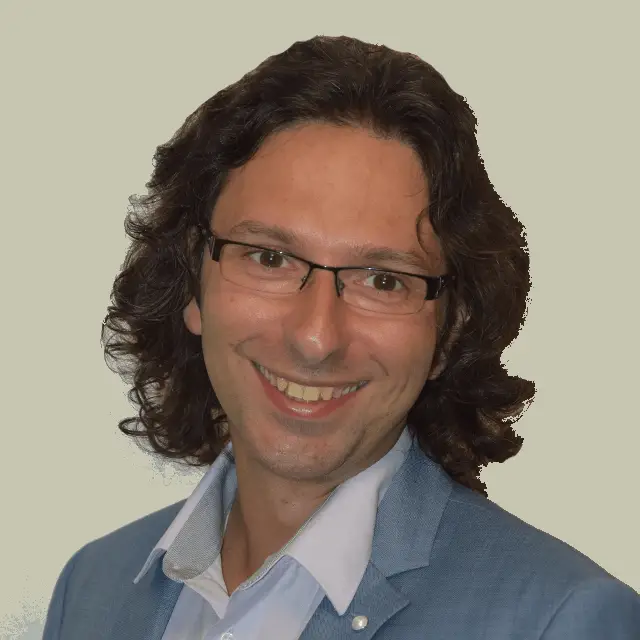
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.