If you are either familiar with Python or if you are starting with it now, it’s likely that you have heard about the Python input function.
The Python input built-in function allows to capture the input provided by a user during the execution of a program. The input function returns the input in string format and its value can be assigned to a variable for later use.
Let’s experiment with the Python input function and see what the function returns when we pass a string or an integer to it.
This tutorial is based on Python 3, this is important to highlight considering that the behaviour of the input function is different between Python 2 and Python 3.
I will use Python 2 only in one of the sections of this tutorial to show you how input works differently between the two Python versions.
Let’s go!
An Example of How to Use Input in Python
I want to create a Python program that asks the user to provide a number and then tells if the number is even or odd.
To do that we will use the input function to read a number from the user. The input function stops the execution of the program until the user provides an input and presses Enter:
print("Enter a number")
number = input()
print(type(number))
As you can see from the code above, I want to check the type of the value returned by the input function.
Here is the ouput:
$ python number.py
Enter a number
3
<class 'str'>
So, despite the fact that I have provided a number, the input function returns an object of type string.
What do I need to do to be able to check if the number is even or odd?
I have to convert it from a string into an integer.
The int() function allows to convert a string into an integer, this is called type casting.
After reading the number we use an if else statement to check if the number provided by the user is even or odd.
I’m curious to see what error I get if I try to check if the number is even or odd before converting it into an integer.
$ python number.py
Enter a number
3
Traceback (most recent call last):
File "number.py", line 4, in <module>
if number % 2 == 0:
TypeError: not all arguments converted during string formatting
An error of type TypeError is raised.
This time let’s use the int() function to convert the number from a string to an integer.
print("Enter a number")
number = input()
if int(number) % 2 == 0:
print("The number is even")
else:
print("The number is odd")
The output becomes:
$ python number.py
Enter a number
3
The number is odd
All good this time!
How to Display a Message to the User with the Input Function
In the previous example I have used a print statement to print a message before asking for an input to be provided.
I can also remove the print statement and pass the prompt argument to the input function.
The prompt argument for the Python input() function is optional and it allows to display a message to our user.
Here is how our program becomes:
number = input("Enter a number: ")
if int(number) % 2 == 0:
print("The number is even")
else:
print("The number is odd")
It’s a more concise option and the output is:
$ python number.py
Enter a number: 3
The number is odd
This time our program doesn’t go to a newline before allowing the user to provide a number. That’s because the prompt message is printed without trailing newline.
The casting from string to integer can also be done immediately on the line where we call the input function instead of doing it in the condition of the if else statement.
number = int(input("Enter a number: "))
if number % 2 == 0:
print("The number is even")
else:
print("The number is odd")
Handling Float Numbers with the Input Function
In the same way we have handled an integer number before, we can also use the input function to read float numbers.
Considering that the Python 3 input() function always returns a string we will need to use a function to convert the string into float. The built-in function to convert a string into a float is called float().
number = float(input("Enter a number: "))
print(type(number))
print("The number is", number)
Let’s execute the program:
$ python float.py
Enter a number: 3.5
<class 'float'>
The number is 3.5
And what if I pass the number 3 as input?
$ python float.py
Enter a number: 3
<class 'float'>
The number is 3.0
Python converts it into a float, 3.0. And the same applies to negative numbers.
Handling Multiple Values with the Input Function
We can also get the user to provide multiple values using the input() function.
For example, let’s say I create a program that asks for name, surname and nationality.
You can use the string split() method together with the input() function to handle multiple values.
name, surname, nationality = input("Enter your name, surname and nationality: ").split()
print("Name: ", name)
print("Surname: ", surname)
print("Nationality: ", nationality)
Let’s execute it!
$ python app.py
Enter your name, surname and nationality: Claudio Sabato italian
Name: Claudio
Surname: Sabato
Nationality: italian
The split() method generates a list from the string returned by the input function. The space character is used as separator because it’s the default separator for the split() method.
Then each element in the list is assigned to the variables name, surname and nationality (this is called unpacking).
The Difference Between Input and Raw_input in Python
Python also provides a function called raw_input.
I would like to understand…
What is the difference between input and raw_input?
Let’s try to replace input with raw_input in our program:
number = int(raw_input("Enter a number: "))
if number % 2 == 0:
print("The number is even")
else:
print("The number is odd")
When I run the program I receive the following error:
$ python number.py
Traceback (most recent call last):
File "number.py", line 1, in <module>
number = int(raw_input("Enter a number: "))
NameError: name 'raw_input' is not defined
According to the error ‘raw_input’ is not defined, that’s because I’m using Python 3 and the raw_input function is only available in Python 2.
Here is the version of Python I was using by default and the Python 2 version I will be using instead to test the raw_input function:
$ python --version
Python 3.7.4
$ python2 --version
Python 2.7.14
And here’s what happens when I run the program with Python 2:
$ python2 number.py
Enter a number: 3
The number is odd
The program works well, but it’s not clear yet the difference between input and raw_input…
I will create another simple program to compare the type of the objects returned by the input and raw_input functions:
number = input("Enter a number (input test): ")
raw_number = raw_input("Enter a number (raw_input test): ")
print "Input type: ", type(number)
print "Raw input type: ", type(raw_number)
As you can see the print statement doesn’t have parentheses because I will be running this program using Python 2. The output is:
$ python2 input_vs_raw_input.py
Enter a number (input test): 3
Enter a number (raw_input test): 3
Input type: <type 'int'>
Raw input type: <type 'str'>
With Python 2, the value returned by the input function is an integer while the value returned by the raw_input function is a string.
At the beginning of this tutorial we have seen that the value returned by the input function with Python 3 is a string.
So…
The input() function works differently between Python 2 and Python 3 when it comes to the type of value returned when an input is provided by the user. The raw_input() function in Python 2 has become the input() function in Python 3, they both return an object of type string.
Using the Eval Function to Evaluate Expressions
So far we have seen how to use the int() and float() functions to convert strings into integer and float numbers.
We can do something similar with the eval built-in function with the difference that with eval you can also evaluate expressions in string format.
Let’s see first what eval returns when applied to a string representation of an integer and float number:
>>> eval('3')
3
>>> eval('3.5')
3.5
And here is how eval can calculates full expressions:
>>> eval('2+3')
5
>>> eval('2+3.5')
5.5
Definitely something useful to know for your Python programs!
Conclusion
In this tutorial we have covered all the basics of the Python input() function.
You now know that this function returns a string with Python 3 and that based on the type of input we can convert it into integer or float numbers using the int() and float() built-in functions.
We have also seen:
- How to get multiple values from the user.
- What is the difference between input and raw_input.
- How to use the eval built-in function.
Do you have any questions?
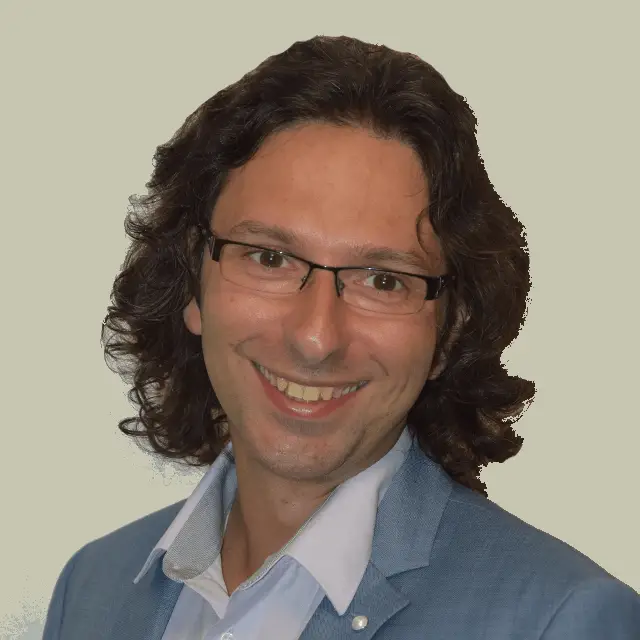
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.