Are you here because you have tried to concatenate a string and an int in Python and you have seen an error? You are in the right place to fix it.
In Python, you cannot concatenate a string and an int using the concatenation operator (+). One way to make the concatenation work is to convert the int into a string first using the str() function. Other options are to use the string format() method or f-strings.
Let’s see some examples of how to concatenate a string to an int!
1. Concatenate a String to an Int using the str() Function
Open the Python shell. In this example, we will create one string and one int variable and then we will try to concatenate them using the concatenation operator (+).
This is quite a common thing you would do in a Python program, for example, if you want to show a user the number of points they have reached in a game you are programming.
>>> message = "Your points are: "
>>> number_of_points = 23
>>> print(message + number_of_points)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: can only concatenate str (not "int") to str
In this example, we want to print the message:”Your points are: 23″.
But as you can see, Python raises the TypeError
“can only concatenate str (not “int”) to str” because it cannot concatenate an integer to a string. It can only concatenate a string to another string.
Explanation: a TypeError
occurs in Python when an operation is applied to a variable (object) of incorrect type.
How can you fix this error?
One way to concatenate a string to an integer is to first convert the integer into a string using the str() function that returns the string version of the integer.
>>> print(message + str(number_of_points))
You points are: 23
This time the print() function doesn’t raise any errors and you can see the full message printed.
This is not the only way to solve this error, let’s see what other options you have so you can choose the one you prefer.
2. Using the format() Method to Concatenate a String to an Int in Python
You can use the string format() method to concatenate a string to an int. The format() method allows you to specify placeholders in your string that get replaced by variables.
Here is how you can use the format() method in this example:
>>> message = "Your points are: "
>>> number_of_points = 23
>>> print("{} {}".format(message, number_of_points))
You points are: 23
The two placeholders {} in the string are replaced by the variables passed to the format() method in the same order in which they are passed to it.
3. Concatenate a String to an Int Using Python f-strings
A more recent approach to fix the error we have seen when concatenating a string to an int is using f-strings that have been introduced in Python 3.6.
Here is how you can use f-strings in this example:
>>> print(f"{message} {number_of_points}")
You points are: 23
This expression is a lot more concise than the one with the format() method. Notice that an f-string requires the letter f before the double quotes for the string you want to create.
Inside the f-string expression, you have placeholders {} that contain each variable in the order in which they appear in the string you are creating.
Example of When to Concatenate a String and an Int in a Python program
You have now learned 3 ways to overcome errors due to trying to concatenate a string and an int.
But what are some common scenarios in which you might find useful concatenating a string and an int in a Python program?
Scenario 1: Generating usernames
If you are building a web application or a game you will likely give users the option to select a custom username. Users could also choose to autogenerate their username.
Here is a simple example of Python code to generate two usernames:
>>> import random
>>> username = f"user{random.randrange(100, 10000)}"
>>> print(username)
user1561
>>> username = f"user{random.randrange(100, 10000)}"
>>> print(username)
user7469
Here you are using f-strings as seen previously and to generate a random number to concatenate to the string “user” you are using the randrange function of the random module.
Scenario 2: Generating filenames
Another example of using string an int concatenation is the generation of filenames. Imagine you are downloading a ticket you purchased online. It’s very common to see that the web application you download the ticket from generates a filename that is a mix of strings and numbers.
Imagine, for example, that every day your system generates a number that increments on that day starting from zero and depending on the number of tickets purchased on that day.
>>> import random
>>> date = "05112023"
>>> ticket_id = 100
>>> ticket_filename = "ticket-{}-{}.pdf".format(date, ticket_id)
>>> print(ticket_filename)
ticket-05112023-100.pdf
In this example, you have used the string format() method. The ticket in this example is ticket 100 on the day specified by the date variable.
Obviously this is just an example and you can change this logic depending on how you want your web application to work.
Scenario 3: Generating URLs
The third example we will look at is the generation of URLs. Let’s take, for example, an eCommerce website that allows filtering items based on their price range.
A common approach to do this is by using URL parameters that define the output to be returned to the user. Let’s take an example of URL to filter items with a price between 100 and 500 dollars.
https://ecommercetest.com/search?price_low=100&price_high=500
This is a simple example but it’s enough to show you that in this case, we are concatenating the values of URL parameters “price_low” and “price_high” to the URL string.
To generate this URL with Python code you could do the following:
>>> price_low = 100
>>> price_high = 500
>>> website_search_url = "ecommercetest.com/search"
>>> search_url = f"https://{website_search_url}?price_low={price_low}&price_high={price_high}"
>>> print(search_url)
https://ecommercetest.com/search?price_low=100&price_high=500
You are using f-strings to concatenate multiple strings and integers.
The logic of your Python code will set the values of the integers price_low and price_high depending on the values provided by the user in the User Interface of your application.
Conclusion
This tutorial has shown you the importance of understanding different data types in Python and what kind of operations you can execute on different data types.
You have also seen three real-world scenarios in which you would use Python code to concatenate strings and integers.
Make sure to practice the examples we went through in this tutorial. If you haven’t tried them on your computer go back and give them a try.
By writing this code yourself you will be able to remember it and to use it in the future when doing concatenation of strings and integers.
Related article: Do you want to learn more? I have created a CodeFatherTech tutorial that will teach you more about string concatenation in Python.
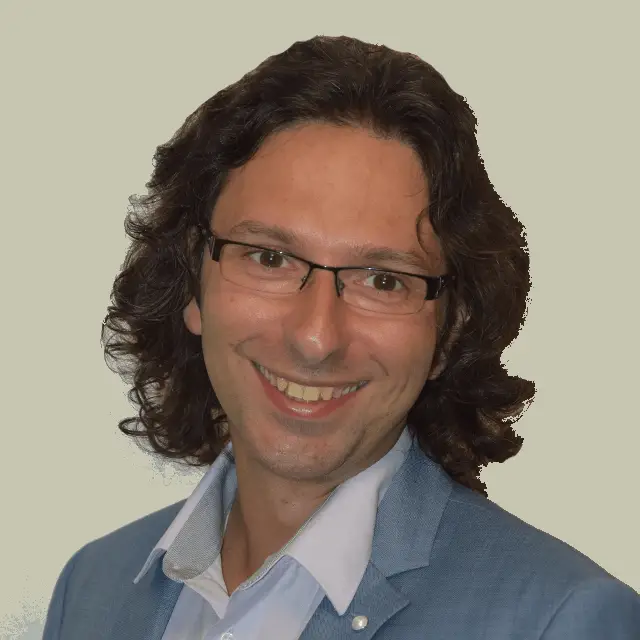
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.