The process of converting one Python data type to another data type is called type casting. Type casting can be useful when you want to ensure that your data is compatible with specific functions or operations (e.g. converting a variable to a string before concatenating it to another string).
How Can You Do Type Casting in Python?
Python provides four built-in functions that can be used for typecasting. These functions are:
- str()
- int()
- float()
- bool()
Each one of these functions if applied to a value returns the value converted into a different data type, respectively a string, an integer, a float, or a boolean.
One important aspect is that not all values can be cast to all data types. For example, if you try to convert a string that doesn’t represent a number to an int or float you will see a ValueError.
Here is the ValueError raised by the Python interpreter if you try to convert an invalid numeric string to an integer.
>>> incorrect_number = '34%'
>>> int(incorrect_number)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '34%'
And here is the error you get when trying to convert an incorrect numeric string to a float.
>>> float(incorrect_number)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: could not convert string to float: '34%'
How to Typecast an int to a string in Python
To convert an integer to a string you can use the Python str() function. If x is the int you want to convert then use str(x) to obtain it in string format.
Let’s see this in practice. Open the Python shell and assign a numeric value to the variable x.
>>> x = 1
Then apply the str() function to x:
>>> str(x)
'1'
Using the type() built-in function you can verify that the type returned by the str() function is a string.
>>> type(str(x))
<class 'str'>
If you want to use this value as part of a mathematical operation you have to convert it back into an integer or a float. If you don’t you will get the following error TypeError:
>>> str(x) + 10
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: can only concatenate str (not "int") to str
As explained by the error, you can only concatenate a string to another string (not an int).
The following expression would give you the correct result:
>>> int(str(x)) + 10
11
How Do You Convert integer to float Using Python?
To convert an integer to a float you can use Python’s float() function.
Assign a value to the integer x and then use the function float() to convert it to a float.
>>> x = 1
Call the float() function and pass x to it:
>>> float(x)
1.0
Using the type() built-in function verify that the type returned by the float() function is a floating point number.
>>> type(float(x))
<class 'float'>
How to use Python to Typecast a float to an int
To convert a float to an integer Python provides the int() built-in function.
Assign a float value to a variable y:
>>> y = 3.14
How can we convert this floating-point number to an integer?
Call the int() function and pass the float number to it.
>>> int(y)
3
As you can see, the output returned by the int() function is an integer. It doesn’t have any digits after the decimal point.
Conversion from float to string Using Python
To convert a float to a string Python provides the str() built-in function.
Create a floating point variable:
>>> z = 24.567
How do you convert this floating point number to a string?
Call the str() function and pass the float number to it.
>>> str(z)
'24.567'
The output returned by the str() function is the string representation of the float. If you want to perform a mathematical operation on this number you have to convert it back to float first.
>>> float(str(z)) + 34.44
59.007
How to Convert a string to an integer Using Python
To convert a string to an int Python provides the int() function. If x is the string you want to convert then use int(x) to convert it to an integer.
Open the Python shell and create a string:
>>> number = '45'
Then call the int() function and pass the string variable number to it.
>>> int(number)
45
Verify that the type returned by the int() function is an integer using the type() built-in function.
>>> type(int(number))
<class 'int'>
Using Python to Convert a string to a float
To convert a string to a float in Python use the float() function. The float() function takes the string as an argument and returns its float representation.
Let’s create a string representing a float:
>>> number = '78.3'
Then call the float() function and pass the string variable to it.
>>> float(number)
78.3
Verify that Python converts the string into a float:
>>> type(float(number))
<class 'float'>
Converting a List to a String in Python
You can use the str() function to convert a Python list to its string representation.
Create a list of numbers:
>>> numbers = [1, 2, 3, 4, 5]
And pass this list as an argument to the str() function:
>>> str(numbers)
'[1, 2, 3, 4, 5]'
>>> type(str(numbers))
<class 'str'>
Conclusion
In this tutorial, we have seen how typecasting works in Python.
Now you know how to convert int, float, string, and boolean from one data type to another.
Related article: in this article, we have talked about integers, strings, and floats in Python. Have a look at boolean values in the CodeFatherTech tutorial about the Python boolean data type.
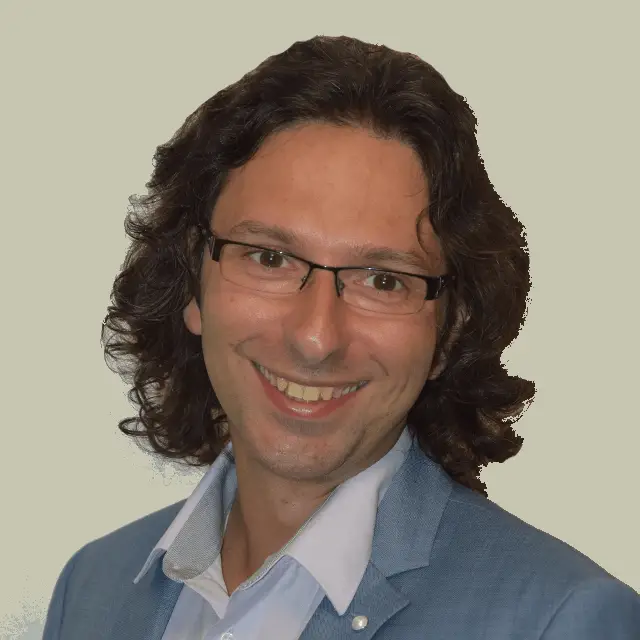
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.