The Python error “too many values to unpack” occurs when you try to extract multiple values from a data structure into variables that don’t match the number of values. For example, if you try to unpack the elements of a list into variables that don’t match the number of elements in the list.
We will look together at some scenarios in which this error occurs, for example when unpacking lists, dictionaries, or when calling Python functions.
How Do You Fix the Too Many Values to Unpack Error in Python
A common scenario in which the error “too many values to unpack” happens is when you try to unpack values from a Python list.
Let’s see a simple example:
>>> week_days = ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday']
>>> day1, day2, day3 = week_days
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: too many values to unpack (expected 3)
The error complains about the fact that the values on the right side of the expression are too many to be assigned to the three variables day1
, day2
, and day3
.
As you can see from the traceback this is an error of type ValueError.
So, what can we do to fix this error?
One option could be to use a number of variables on the left that matches the number of values to unpack, in this case, five:
>>> day1, day2, day3, day4, day5 = week_days
>>> day1
'Monday'
>>> day5
'Friday'
This time there’s no error and each variable has one of the values inside the week_days
array.
In this example, the error was raised because we had too many values to assign to the variables in our expression.
Let’s see what happens if you don’t have enough values to assign to variables:
>>> weekend_days = ['Saturday' , 'Sunday']
>>> day1, day2, day3 = weekend_days
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: not enough values to unpack (expected 3, got 2)
In this example, we are trying to assign two values to the three variables day1
, day2
, and day3
.
That’s why the error says “not enough values to unpack (expected 3, got 2)”. Because Python is expecting 3 values to assign to the variables on the left but it only got 2 values.
In this case, the correct expression would be:
>>> day1, day2 = weekend_days
ValueError When Calling a Python Function
The same error can occur when you call a Python function incorrectly.
I will define a simple function that takes a number as input, x
, and returns as output two numbers, the square and the cube of x
.
>>> def getSquareAndCube(x):
return x**2, x**3
>>> square, cube = getSquareAndCube(2)
>>> square
4
>>> cube
8
What happens if, by mistake, we assign the values returned by the function to three variables instead of two?
>>> square, cube, other = getSquareAndCube(2)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: not enough values to unpack (expected 3, got 2)
We see the error “not enough values to unpack” because the values to unpack are two but the variables on the left side of the expression are three.
And what if you assign the output of the function to a single variable?
>>> output = getSquareAndCube(2)
>>> output
(4, 8)
Everything works well and Python makes the output variable a tuple that contains both values returned by the getSquareAndCube()
function.
Too Many Values to Unpack when Using the Input Function
Another common scenario where this error can occur is when you use the Python input() function to ask users to provide inputs.
The Python input() function reads the input from the user and converts it into a string before returning it.
Here’s a simple example:
>>> name, surname = input("Enter your name and surname: ")
Enter your name and surname: John Smith
Traceback (most recent call last):
File "<pyshell#4>", line 1, in <module>
name, surname = input("Enter your name and surname: ")
ValueError: too many values to unpack (expected 2)
Why Python is complaining about too many values to unpack?
That’s because the input() function converts the input into a string, in this case, “John Smith”, and then it tries to assign each character of the string to the variables on the left.
So we have multiple characters on the right part of the expression being assigned to two variables, that’s why Python is saying that it expects two values.
What can we do about it?
We can apply the string method split() to the output of the input function:
>>> name, surname = input("Enter your name and surname: ").split()
Enter your name and surname: John Smith
>>> name
'John'
>>> surname
'Smith'
The split method converts the string returned by the input function into a list of strings and the two elements of the list get assigned to the variables name and surname.
By default, the split method uses the space as a separator. If you want to use a different separator you can pass it as the first parameter to the split method.
Using Maxsplit to Solve The Python Error “too many values to unpack”
There is also another way to solve the problem we have observed while unpacking a list.
Let’s start again with the following code:
>>> name, surname = input("Enter your name and surname: ").split()
This time we will provide a different string to the input function:
Enter your name and surname: Mr John Smith
Traceback (most recent call last):
File "<pyshell#29>", line 1, in <module>
name, surname = input("Enter your name and surname: ").split()
ValueError: too many values to unpack (expected 2)
Similarly, as we have seen before, this error occurs because split() converts the input string into a list of three elements. And three elements cannot be assigned to two variables.
There’s a way to tell Python to split the string returned by the input function into values that match the number of variables, in this case, two.
Here is the generic syntax for the split() method that allows to do that:
<string>.split(separator, maxsplit)
The maxsplit parameter defines the maximum number of splits used by the Python split method when converting a string into a list. Maxsplit is an optional parameter.
So, in our case, let’s see what happens if we set maxsplit to 1.
>>> name, surname = input("Enter your name and surname: ").split(' ', 1)
Enter your name and surname: Mr John Smith
>>> name
'Mr'
>>> surname
'John Smith'
The error is gone, the logic of this line is not perfect considering that surname
is ‘John Smith’. But this is just an example to show how maxsplit works.
So, why are we setting maxsplit to 1?
Because in this way the string returned by the input function is only split once when being converted into a list, this means the result is a list with two elements (matching the two variables on the left of our expression).
Too Many Values to Unpack when You Iterate Over a Dictionary
In the last example, we will use a Python dictionary to explain another common error that shows up while developing.
I have created a simple program to print every key and value in the users
dictionary:
users = {
'username' : 'jsmith',
'name' : 'John',
}
for key, value in users:
print(key, value)
When you run it you see the following error:
$ python dict_example.py
Traceback (most recent call last):
File "dict_example.py", line 6, in <module>
for key, value in users:
ValueError: too many values to unpack (expected 2)
Where is the problem?
Let’s try to execute a for loop using just one variable:
for key in users:
print(key)
The output is:
$ python dict_example.py
username
name
When we loop through a dictionary using its name we get back just the keys.
That’s why we were seeing an error before, we were trying to unpack each key into two variables: key and value.
To retrieve each key and value from a dictionary we need to use the dictionary items() method.
Let’s run the following:
for user in users.items():
print(user)
This time the output is:
('username', 'jsmith')
('name', 'John')
At every iteration of the for loop we get back a tuple that contains a key and its value. This tuple can be assigned to the two variables we were using before.
So, the program becomes:
users = {
'username' : 'jsmith',
'name' : 'John',
}
for key, value in users.items():
print(key, value)
The program prints the following output:
$ python dict_example.py
username jsmith
name John
All good, the error is fixed!
And you? Where are you seeing this error?
Let me know in the comments below.
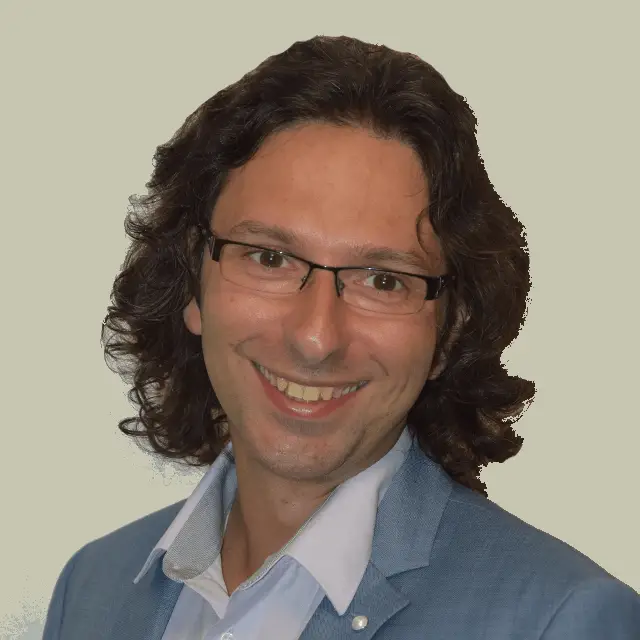
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
Nice explains, Your article so easy to understand