Have you seen the TypeError: ‘list’ object is not an iterator when executing your Python program? Learn why and how to fix it.
The reason why you see the error “TypeError: ‘list’ object is not an iterator” when running a Python program is that you are using a list as if it was an iterator. The list data type in Python is an iterable but is not an iterator. To be able to use a list as an iterator you have to apply to it a function that returns an iterator first.
Don’t worry if this doesn’t make sense right now. We will go through a few examples that will help you understand this Python error.
Let’s get started!
How Do You Fix the TypeError ‘list’ object is not an iterator in Your Python Code?
Before learning how to fix this error, let’s understand why it happens.
The error “TypeError ‘list’ object is not an iterator” occurs because in your Python program, you are executing an operation against a Python list when that operation is only supported by an iterator.
Here is an example of code that can cause this error:
numbers = [1, 4, 67, 5, 43]
next_number = next(numbers)
On the first line of code, we are creating a Python list that contains numbers. On the second line, we pass the list to the next() built-in function.
And the output of this code is:
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/list_iterator.py", line 2, in <module>
next_number = next(numbers)
^^^^^^^^^^^^^
TypeError: 'list' object is not an iterator
The reason why Python says that a “list” object is not an iterator is that the next() function accepts as an argument an iterator and a list is an iterable but it’s not an iterator.
To get an iterator from a list you have to call the iter() built-in function first, which returns an iterator object.
Let’s print the object returned by the iter() function when it’s applied to a list.
numbers = [1, 4, 67, 5, 43]
numbers_iterator = iter(numbers)
print(numbers_iterator)
[output]
<list_iterator object at 0x1042999f0>
The variable returned by the iter() function is an iterator. This means that now we can pass it to the next() function to get the first item of the list.
numbers = [1, 4, 67, 5, 43]
numbers_iterator = iter(numbers)
next_number = next(numbers_iterator)
print(next_number)
[output]
1
You can also call the next() function multiple times and this returns every element of the list until there are no more elements. At this point, Python raises a StopIteration exception.
next_number = next(numbers_iterator)
print("Element #1:", next_number)
next_number = next(numbers_iterator)
print("Element #2:", next_number)
next_number = next(numbers_iterator)
print("Element #3:", next_number)
next_number = next(numbers_iterator)
print("Element #4:", next_number)
next_number = next(numbers_iterator)
print("Element #5:", next_number)
next_number = next(numbers_iterator)
print("Element #6 (raises a StopIteration exception):", next_number)
Here is the output of this code:
Element #1: 1
Element #2: 4
Element #3: 67
Element #4: 5
Element #5: 43
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/list_iterator.py", line 18, in <module>
next_number = next(numbers_iterator)
^^^^^^^^^^^^^^^^^^^^^^
StopIteration
Now you know why this error occurs and how to fix it.
Why a List is Not an Iterator in Python
The reason why a list is not an iterator is that it doesn’t implement a specific method: the __next__() method.
An iterator in Python has to implement the __iter__() and __next__() methods. A Python list is an iterable but it’s not an iterator because it doesn’t implement the __next__() method. To get an iterator from a list you have to pass a list to the Python built-in function iter().
Let’s take as an example the following list of numbers and use the dir() function to analyze the methods provided by this list (and any Python lists in general):
numbers = [1, 4, 67, 5, 43]
print(dir(numbers))
Here are the list methods:
['__add__', '__class__', '__class_getitem__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getstate__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
You can see that the list implements the __iter__() method but it doesn’t have the __next__() method, reason why it’s not an iterator.
How to Convert a List to an Iterator in Python
There are two ways to get an iterator from a list:
- Pass the list to the Python iter() built-in function
- Call the __iter__() method of a list
Let’s see an example of both, starting with the iter() function.
numbers = [1, 4, 67, 5, 43]
print(iter(numbers))
[output]
<list_iterator object at 0x100c659f0>
You can see from the output of the print() function that the object returned by the iter() function is an iterator object.
In the second example, we can call the __iter__() method of this list.
What do you get back?
numbers = [1, 4, 67, 5, 43]
print(numbers.__iter__())
[output]
<list_iterator object at 0x1010319f0>
Once again, we get back an iterator object.
This means that…
Passing a list to the iter() function or calling the __iter__() method of a list has the same result, it returns an iterator for that list.
How Do You Check If a Python Object is an Iterator?
Two ways to check if a Python object is an iterator are:
- passing the object to the next() function and confirming no TypeError exception is raised.
- verifying that the object has the following two methods: __iter__() and ___next__().
Here is the Python code to implement the first approach. We will apply it to a list:
numbers = [1, 4, 67, 5, 43]
try:
next(numbers)
except:
print("This object is not an iterator")
else:
print("This object is an iterator")
The code in the except block is executed if Python raises an exception in the try block. If no exception is raised in the try block Python executes the code in the else block.
The output is:
This object is not an iterator
With the second approach, we use the hasattr() built-in function to find out if the list implements the two methods __iter__() and ___next__() required by an iterator.
numbers = [1, 4, 67, 5, 43]
if hasattr(numbers, '__iter__') and hasattr(numbers, '__next__'):
print("This object is an iterator")
else:
print("This object is not an iterator")
In the output, we expect to see that this object is not an iterator. Verify that the output is correct.
Conclusion
In this tutorial, you learned why the exception “TypeError ‘list’ object is not an iterator” is raised by the Python interpreter and what you can do to fix it.
We also covered basic knowledge about iterables and iterators so that you now understand how to work with them in future Python programs.
Related article: to go more in-depth about this topic have a look at the CodeFatherTech tutorial about the Python next() function.
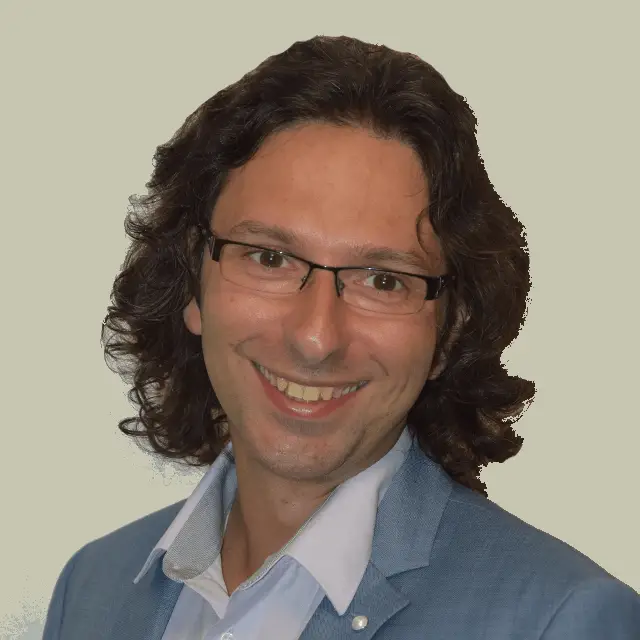
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.