Are you trying to get the last element in a Python list? You are in the right place.
There are multiple ways to get the last element in a Python list. There are two approaches that use indexes: the first with the negative index -1 and the second with the last index of the list. An alternative is also to use the list pop() method but this approach changes the original list while the first approach doesn’t.
Are you ready for a few Python examples that will make things clear?
Let’s learn multiple ways to get the last element of a Python list!
How Do You Get the Last Element of a List in Python Using a Negative Index?
The simplest way to get the last element of a Python list is by using the negative index -1. When using negative indexes in a list you access the elements in the list starting from the last one. That’s why the index with value -1 allows accessing the last element in a Python list.
In the same way, if you access the element with index -2 you get back the value of the element before the last.
Let’s create a list and show how to retrieve the last element:
numbers = [1, 4, 56, 78, 32, 3, 45]
last_number = numbers[-1]
print("Last element in the list:", last_number)
In this code, we have:
- created a list of numbers
- accessed the last element of the list using the index -1
- used the print() function to print a message that shows the last element in the list
And here is the output of this code:
Last element in the list: 45
It’s important to know that when you access the last element in a list using this technique, you don’t change the original list.
I want to make sure this is clear because there are other ways to get the last element in the list that change the original list.
Get the Last Element of a List in Python Using the Last Index
In the previous section, you learned how to access the last element in a list using the negative index -1.
There is also another way to get to the last element of a list using indexes…
To get the last element in a list you can access the last index of the list. You calculate the last index of a list by using the Python len() function. Considering that the indexes of a Python list start from 0, the value of the last index in a list is len(list) – 1.
Let’s access the last item in a list using this approach. Remember that to specify the index of a list you use the list variable name followed by square brackets. The index value is specified within square brackets.
First of all, calculate the value of the last index of the list using the len() function.
numbers = [1, 4, 56, 78, 32, 3, 45]
print("Last index of the list:", len(numbers)-1)
[output]
Last index of the list: 6
The last index of the list is 6 because the list has 7 elements. The index of the first element is 0, the index of the second element is 1 and so on.
Now that you know how to calculate the last index of the list, you can use it to access the last element in the list.
numbers = [1, 4, 56, 78, 32, 3, 45]
last_number = numbers[len(numbers)-1]
print("Last element in the list:", last_number)
[output]
Last element in the list: 45
The value is correct and it’s the same you have seen when using the index -1.
Retrieve the Last Element of a List Using the Python reverse() Method
You can also get the last element in a list by reversing the elements of the list. After reversing the list you simply access the first element in the reversed list.
The list reverse() method doesn’t return any value. It simply reverses the list in place.
Here is an example:
numbers = [1, 4, 56, 78, 32, 3, 45]
numbers.reverse()
print(numbers)
[output]
[45, 3, 32, 78, 56, 4, 1]
After calling the reverse() method on the list numbers, the values in the list are reversed and now the first element is the one that was previously the last element.
Let’s use the index 0 to access the first element in the reversed list (last element in the original list).
Here is the final Python code:
numbers = [1, 4, 56, 78, 32, 3, 45]
numbers.reverse()
last_number = numbers[0]
print("Last element in the list:", last_number)
[output]
Last element in the list: 45
This approach works but it doesn’t really make sense to reverse a list when you can simply use the negative index -1 to access the last element of the original list as we have seen before.
At the same time, this example shows you how to reverse a list, and this is something you might find useful when writing Python code in the future.
Before, we also mentioned that the list reverse() method doesn’t return any value.
Let’s confirm it:
numbers = [1, 4, 56, 78, 32, 3, 45]
return_value = numbers.reverse()
print(return_value)
[output]
None
The reverse() method returns None which means “no value” in Python.
How Can You Get the Last Item in a Python List Using the pop() Method?
Are there other ways to get the last element from a Python list?
A way to get the last element from a Python list is by using the list pop() method. The pop() method removes from the list the item at the position specified by the index passed to pop() and returns it. You can also get the last item with pop() without providing an index (optional) considering that pop() defaults to the last index in the list.
Let’s start with an example in which we pass the negative index -1 to pop():
numbers = [1, 4, 56, 78, 32, 3, 45]
print("List before pop:", numbers)
last_number = numbers.pop(-1)
print("List after pop:", numbers)
print("Last element in the list:", last_number)
We are still using the -1 index we have seen in the previous section. The value returned is correct:
List before pop: [1, 4, 56, 78, 32, 3, 45]
List after pop: [1, 4, 56, 78, 32, 3]
Last element in the list: 45
From the output, you can also see that after calling pop() the last item is not present anymore in the list.
Important: after calling pop() the original list changes.
Here is what happens when you call pop() without passing an index.
numbers = [1, 4, 56, 78, 32, 3, 45]
print("List before pop:", numbers)
last_number = numbers.pop()
print("List after pop:", numbers)
print("Last element in the list:", last_number)
The only line of code we changed is the one in which we call the pop() method. We have removed the index -1 and we haven’t passed any index to pop().
Here is the output that, once again, is correct:
List before pop: [1, 4, 56, 78, 32, 3, 45]
List after pop: [1, 4, 56, 78, 32, 3]
Last element in the list: 45
Conclusion
In this article, you learned multiple ways to access the last element in a Python list.
You have seen two ways to get the last element of a list:
- using the negative index -1
- using the last index of the list: len(list) – 1.
You also learned that lists provide the pop() method that can be used to retrieve the last element in a list but also changes the original list by removing the last element from it.
We have also seen that you can reverse the elements of the list and then access the element with index 0 in the updated list.
Which approach will you use in your Python programs?
Related article: to continue building your Python knowledge, learn more about the methods supported by Python lists. Read the CodeFatherTech tutorial on Python list methods.
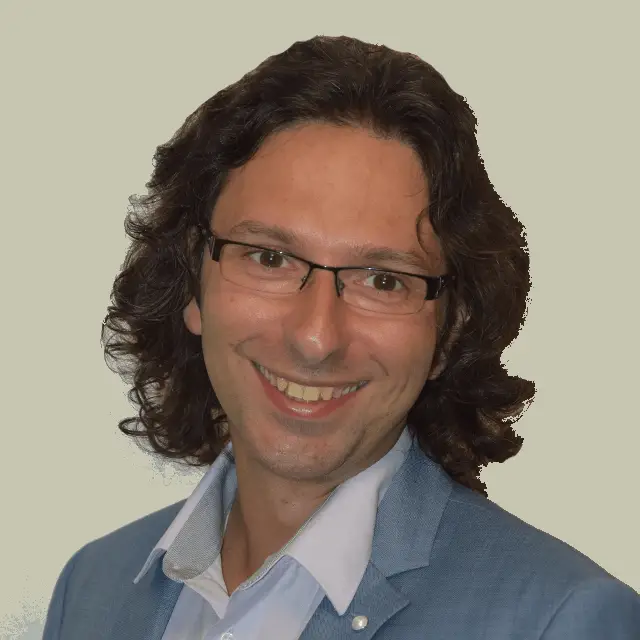
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.