Getting every other element from a Python list might seem easy to do but it actually requires some knowledge of Python basics.
To get every other element in a Python list you can use the slice operator and use the fact that this operator allows to provide a step argument (with its extended syntax). Another approach is to use the mathematical concept of remainder and apply it to list indexes.
Let’s get creative and come up with multiple ways to solve this problem!
How Do I Show Every Other Element in a Python List?
Firstly, let’s create a list of strings…
>>> countries = ['Italy', 'United Kingdom', 'Germany', 'United States', 'France']
>>> print(countries[::2])
['Italy', 'Germany', 'France']
Cool, the slice operator returns every other element starting from the first.
The slice operator follows the syntax below (this is actually called extended slice syntax).
string[start:end:step]
When start and end are not specified the entire list is taken into account.
The step value allows to skip some of the items in the list, in our example we have passed 2 as the value of the step. That’s why the output prints every other string in the list.
If we want to start from the second element in the list the syntax becomes the following:
>>> print(countries[1::2])
['United Kingdom', 'United States']
This time we have started by index 1, remember that the index for lists starts from zero.
How to Print Every Second Element of a List Using the Range Function
You can use a for loop and the range() function to print every second element of a list
Firstly, let’s see what the range function returns by casting its output to a list:
>>> list(range(0,5))
[0, 1, 2, 3, 4]
So, the values returned include the first argument passed to the range function and don’t include the second argument.
The range function also accepts a third argument, the step. This is similar to what we have seen with the slice operator.
>>> list(range(0,5,2))
[0, 2, 4]
To return every second element we have to change the start value to 1.
>>> list(range(1,5,2))
[1, 3]
Now, create a function that uses a for loop and the range function to generate list indexes.
The first argument (start) is equal to 1 and the second argument (end) is equal to the length of the list.
def every_second_element(values):
second_values = []
for index in range(1, len(values), 2):
second_values.append(values[index])
return second_values
We use the list append method to create a new list that only contains every second value, we then return the new list at the end of the function.
You can then call the every_second_element function.
countries = ['Italy', 'United Kingdom', 'Germany', 'United States', 'France']
print(every_second_element(countries))
[output]
['United Kingdom', 'United States']
How to Get Every Other Element of a List Using a List Comprehension
The list comprehension is a Python construct that provides an alternative to for loops.
Let’s write the function we have created in the previous section but this time using a list comprehension.
We will still use the range() function.
>>> every_other_elements = [countries[index] for index in range(1, len(countries), 2)]
>>> print(every_other_elements)
['United Kingdom', 'United States']
When you see a list comprehension for the first time it might seem complex, but it’s actually straightforward when you get used to it.
And the big advantage is that it has replaced a function made of multiple lines with a single line of code.
Fantastic!
Using the Enumerate Function to Get Every Other List Element in Python
Another way to get every other element from a list is by using the Python enumerate function.
The enumerate function returns an enumerate object:
>>> print(enumerate(countries))
<enumerate object at 0x7fd2801c85c0>
What can we do with this object?
You can use an enumerate object to print index and value for each element in a list.
>>> for index, value in enumerate(countries):
... print(index, value)
...
0 Italy
1 United Kingdom
2 Germany
3 United States
4 France
That’s handy because you don’t have to handle the index yourself avoiding potential errors when working with indexes.
Use a list comprehension and the enumerate function to print every second element of a list.
>>> countries = ['Italy', 'United Kingdom', 'Germany', 'United States', 'France']
>>> every_other_countries = [value for index, value in enumerate(countries) if index % 2 == 1]
>>> print(every_other_countries)
['United Kingdom', 'United States']
If you look at the end of the list comprehension, you can see that we have also used the remainder (Python modulo operator) to print only elements with odd indexes.
Extract Every Other Element From a Python List Using a Lambda
To extract every other element from a list we can also use a Python lambda function.
When used together with filter() a lambda function allows to filter each element of a list.
filter(function, iterable)
We can use a lambda function to verify if the reminder for a given index is equal to 1.
>>> lambda index: index % 2 == 1
<function <lambda> at 0x7fd28016dc10>
Here is how it looks like when applied to the indexes of our list:
>>> countries = ['Italy', 'United Kingdom', 'Germany', 'United States', 'France']
Let’s call the filter function and pass the following arguments to it:
- Lambda function: it verifies the remainder for a given list index. If the lambda function returns True the index is included in the output of the filter function.
- Indexes from the list: you can retrieve those indexes by using the range() and len() functions.
>>> list(filter(lambda x: x % 2 == 1, range(len(countries))))
[1, 3]
Now, use the call to the filter function inside a list comprehension…
>>> every_other_elements = [countries[index] for index in filter(lambda x: x % 2 == 1, range(len(countries)))]
>>> print(every_other_elements)
['United Kingdom', 'United States']
The result is the same we have seen in previous sections but the implementation is a lot more complex.
Still, this is an opportunity for you to learn how to use a few Python constructs:
- List comprehension
- For loop
- Filter function
- Lambda function
- Modulo operator.
- range() function
- len() function
How to Get Every nth Element from a Python List
We will complete this tutorial by looking at how to retrieve every nth element from a Python list.
Let’s create a function that takes three arguments:
- original list
- start index for the filtered list
- n: if n is 2 then we get every second element, if n is 3 then we retrieve every third element, and so on.
def get_every_nth_element(values, start_index, n):
return values[start_index::n]
Now, use the input function to get the values of start index and n from the command line when executing the Python program.
start_index = int(input("Specify the start index: "))
n = int(input("Specify the value of n: "))
Note: we have used the int() function to convert the strings returned by the input() function into integers.
Finally we can call our function:
countries = ['Italy', 'United Kingdom', 'Germany', 'United States', 'France']
print("Original list: {}".format(countries))
print("Filtered list: {}".format(get_every_nth_element(countries, start_index, n)))
The two print statements use the string format() method to print two messages when you execute the program.
Here are three runs of the program to make sure it returns the expected result.
Specify the start index: 0
Specify the value of n: 2
Original list: ['Italy', 'United Kingdom', 'Germany', 'United States', 'France'
Filtered list: ['Italy', 'Germany', 'France']
Specify the start index: 1
Specify the value of n: 2
Original list: ['Italy', 'United Kingdom', 'Germany', 'United States', 'France']
Filtered list: ['United Kingdom', 'United States']
Specify the start index: 0
Specify the value of n: 3
Original list: ['Italy', 'United Kingdom', 'Germany', 'United States', 'France']
Filtered list: ['Italy', 'United States']
All good, the code works fine 🙂
Conclusion
We have seen so many different ways to get every other element in a Python list.
You have seen how to use:
- slice operator (extended syntax).
- for loop with range() function.
- list comprehension with range() function.
- list comprehension with enumerate function.
- list comprehension with filter, lambda, range, and len functions.
The simplest approach is the extended syntax for the slice operator.
Which one do you prefer?
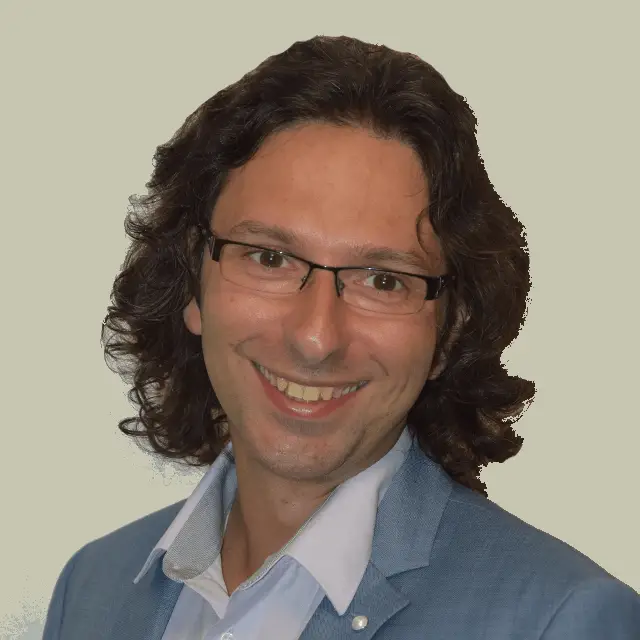
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.