The following 10 challenges have been created for students who are either new to Python or want to strengthen their existing knowledge of Python lists.
These tasks will test your comprehension and improve your ability to manipulate and interact with lists.
Exercise 1
Grocery List: Create a list of your favourite groceries, then:
- Print the entire list.
- Add a new item using
append
. - Remove an item using
remove
. - Check if a particular item is in your list using
in
.
Solution to Exercise 1
1. Create and print the entire list
groceries = ["apples", "bread", "milk", "eggs", "cheese"]
print("Current grocery list:", groceries)
# Current grocery list: ['apples', 'bread', 'milk', 'eggs', 'cheese']
2. Add a new item using append
Using the groceries
list above:
groceries.append("pasta")
print("List after adding pasta:", groceries)
# List after adding pasta: ['apples', 'bread', 'milk', 'eggs', 'cheese', 'pasta']
3. Remove an item using remove
Using the groceries
list above:
groceries.remove("eggs")
print("List after removing eggs:", groceries)
# List after removing eggs: ['apples', 'bread', 'milk', 'cheese', 'pasta']
4. Check if a particular item is in your list using the “in” operator
Using the same list above:
item_to_check = "milk"
if item_to_check in groceries:
print(f"{item_to_check} is in the list.")
else:
print(f"{item_to_check} is not in the list.")
# milk is in the list.
Note: in this example of code we are using Python f-strings.
Exercise 2
Pets: Create a list of your pets’ names:
- Sort the list of names alphabetically using the sorted function.
- Print each pet’s name with its first letter capitalized.
Solution to Exercise 2
1. Sort the list of names alphabetically using the sorted function.
pet_names = ["Luna", "Sunshine", "Charlie", "Whisker"]
# Sorting the list
sorted_names = sorted(pet_names)
print("Names sorted alphabetically:", sorted_names)
# Names sorted alphabetically: ['Charlie', 'Luna', 'Sunshine', 'Whisker']
2. Print each pet’s name with its first letter capitalized.
Using the list pet_names
after changing the first letter of each name to lowercase:
pet_names = ["luna", "sunshine", "charlie", "whisker"]
for name in pet_names:
capitalized_name = name[0].upper() + name[1:].lower()
print(capitalized_name)
# Luna
# Sunshine
# Charlie
# Whisker
Exercise 3
Numbers: Generate a list of numbers from 1 to 10 then:
- Reverse the list in two ways: using slicing and a loop.
- Find the sum and average of all numbers in the list.
- Extract a sublist containing only odd numbers (use slicing).
Solution to Exercise 3
1. Reverse the list in two ways: using slicing and a loop.
numbers = list(range(1, 11))
print("Original list:", numbers)
# Original list: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Using slicing
reversed_list_slice = numbers[::-1] # Slicing from the end to the beginning with steps of -1
print("Reversed list (slicing):", reversed_list_slice)
# Reversed list (slicing): [10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
# Using loop
reversed_list_loop = []
for i in range(len(numbers) - 1, -1, -1): # Iterate from the last index to 0 with steps of -1
reversed_list_loop.append(numbers[i])
print("Reversed list (loop):", reversed_list_loop)
# Reversed list (loop): [10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
2. Find the sum and average of all numbers in the list.
Using the newly generated list: numbers
.
sum_of_numbers = sum(numbers)
average = sum_of_numbers / len(numbers)
print("Sum of numbers:", sum_of_numbers)
print("Average of numbers:", average)
# Sum of numbers: 55
# Average of numbers: 5.5
3. Extract a sublist containing only odd numbers (use slicing).
We will again use the list numbers
above:
odd_numbers = numbers[::2] # Every other element starting from index 0 (inclusive)
print("Odd numbers:", odd_numbers)
# Odd numbers: [1, 3, 5, 7, 9]
Exercise 4
Counting Characters: Create a list of your favourite words, then:
- Find the word with the most characters using loops and conditionals.
- Access and print the third character of each word using indexing.
- Create a new list containing only words with an even number of characters.
Solution to Exercise 4
1. Find the word with the most characters using loops and conditionals
favourite_words = ["python", "programming", "beautiful", "powerful", "language"]
# Initialize variables
longest_word = ""
longest_length = 0
# Loop through each word
for word in favourite_words:
# Get the current word's length
word_length = len(word)
# Check if it's longer than the previous longest
if word_length > longest_length:
longest_word = word
longest_length = word_length
# Print the result
print("Word with the most characters:", longest_word)
# Word with the most characters: programming
2. Access and print the third character of each word using indexing
Using the list above favourite_words
:
favourite_words = ["python", "programming", "beautiful", "powerful", "language"]
# Loop through each word
for word in favourite_words:
# Check if the word has at least 3 characters
if len(word) >= 3:
third_char = word[2] # Access the third character (index 2)
print("Third character of", word, ":", third_char)
else:
print("Word", word, "is less than 3 characters long.")
# Third character of python : t
# Third character of programming : o
# Third character of beautiful : a
# Third character of powerful : w
# Third character of language : n
3. Create a new list containing only words with an even number of characters
Using the favourite_words
list here also:
even_length_words = []
# Loop through each word
for word in favourite_words:
# Check if the word length is even using the modulo operator (%)
if len(word) % 2 == 0:
even_length_words.append(word)
# Print the new list
print("Words with even number of characters:", even_length_words)
# Words with even number of characters: ['python', 'powerful', 'language']
Exercise 5
Text Twist: Generate a list of your favourite quotes, then:
- Convert all quotes to uppercase using a list comprehension.
- Count the occurrences of a specific word across all quotes.
- Create a new list containing only quotes longer than a certain length.
Solution to Exercise 5
1. Convert all quotes to uppercase using list comprehensions
quotes = ["The best way to predict the future is to create it.", "The only way to do great work is to love what you do.", "If you can dream it, you can do it."]
uppercase_quotes = [quote.upper() for quote in quotes]
print("Quotes in uppercase:", uppercase_quotes)
# Quotes in uppercase: ['THE BEST WAY TO PREDICT THE FUTURE IS TO CREATE IT.', 'THE ONLY WAY TO DO GREAT WORK IS TO LOVE WHAT YOU DO.', 'IF YOU CAN DREAM IT, YOU CAN DO IT.']
2. Count the occurrences of a specific word across all quotes
Using the list quotes
:
target_word = "dream" # Replace with your desired word
word_count = sum(1 for quote in quotes if target_word.lower() in quote.lower())
print("Number of occurrences of", target_word, ":", word_count)
# Number of occurrences of dream : 1
3. Create a new list containing only quotes longer than a certain length
Using the same list above: quotes
.
min_length = 50
long_quotes = [quote for quote in quotes if len(quote) > min_length]
print("Quotes longer than", min_length, "characters:", long_quotes)
# Quotes longer than 50 characters: ['The best way to predict the future is to create it.', 'The only way to do great work is to love what you do.']
Exercise 6
Friend Frenzy: Create a list of friends, each represented as a dictionary containing name, age, and hobbies (as another list). Then:
- Print all friends with a specific hobby.
- Sort the list alphabetically by name using the sorted function.
- Find the average age of all your friends.
Solution to Exercise 6
1. Print all friends with a specific hobby
friends = [
{"name": "Alice", "age": 25, "hobbies": ["reading", "hiking", "cooking"]},
{"name": "Bob", "age": 30, "hobbies": ["gaming", "music", "sports"]},
{"name": "Charlie", "age": 28, "hobbies": ["photography", "travel", "coding"]},
{"name": "Diana", "age": 22, "hobbies": ["dancing", "painting", "volunteering"]}
]
target_hobby = "coding" # Replace with your desired hobby
friends_with_hobby = [friend for friend in friends if target_hobby in friend["hobbies"]]
print("Friends with", target_hobby, "hobby:", friends_with_hobby)
# Friends with coding hobby: [{'name': 'Charlie', 'age': 28, 'hobbies': ['photography', 'travel', 'coding']}]
2. Sort the list alphabetically by name using the sorted function.
Using the list above:
sorted_friends = sorted(friends, key=lambda friend: friend["name"])
print("Friends sorted alphabetically by name:", sorted_friends)
# Friends sorted alphabetically by name: [{'name': 'Alice', 'age': 25, 'hobbies': ['reading', 'hiking', 'cooking']}, {'name': 'Bob', 'age': 30, 'hobbies': ['gaming', 'music', 'sports']}, {'name': 'Charlie', 'age': 28, 'hobbies': ['photography', 'travel', 'coding']}, {'name': 'Diana', 'age': 22, 'hobbies': ['dancing', 'painting', 'volunteering']}]
3. Find the average age of all your friends
We will use the same list above:
total_age = sum(friend["age"] for friend in friends)
average_age = total_age / len(friends)
print("Average age of friends:", average_age)
# Average age of friends: 26.25
Exercise 7
Random activities: Create a list of your favourite activities, then:
- Pick a random activity to do using
random.choice
. - Create a list of “would you rather” questions and randomly pick one to ponder.
Solution to Exercise 7
1. Pick a random activity to do using random.choice
import random
activities = ["read a book", "watch a movie", "go for a walk", "play a game", "learn something new"]
random_activity = random.choice(activities)
print("Pick a random activity:", random_activity)
# Pick a random activity: learn something new
2. Create a list of “would you rather” questions and randomly pick one to ponder
import random
questions = [
"Would you rather travel to the past or the future?",
"Would you rather have the ability to fly or be invisible?",
"Would you rather have super strength or super speed?"
]
random_question = random.choice(questions)
print("Random 'would you rather' question:", random_question)
# Random 'would you rather' question: Would you rather travel to the past or the future?
Exercise 8
Matrix Manipulation: Create a 2D list (a matrix) representing a grid or table, then:
- Print the matrix in a well-formatted way.
- Access and print a specific row and column.
- Modify an element and print the updated matrix.
Solution to Exercise 8
1. Print the matrix:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in matrix:
print(row)
# [1, 2, 3]
# [4, 5, 6]
# [7, 8, 9]
2. Access and print a specific row and column:
Accessing the same list above:
# Access the second row
second_row = matrix[1]
print("Second row:", second_row)
# Second row: [4, 5, 6]
# Access the third element of the first row
element = matrix[0][2]
print("Third element of the first row:", element)
# Third element of the first row: 3
3. Modify an element and print the updated matrix:
Using the same list:
# Change the element in the second row, third column to 10
matrix[1][2] = 10
for row in matrix:
print(row)
# [1, 2, 3]
# [4, 5, 10]
# [7, 8, 9]
Exercise 9
List of Tuples: Work with a list of tuples, where each tuple contains the name of a city and its population, then:
- Sort the list by population in ascending order.
- Find and print the city with the highest population.
- Filter and print cities with a population greater than a specified value.
Solution to Exercise 9
1. Sort the list by population in ascending order
cities = [("Tokyo", 37400068), ("Delhi", 28514000), ("Shanghai", 25582000)]
sorted_cities = sorted(cities, key=lambda city: city[1])
print("Cities sorted by population:", sorted_cities)
# Cities sorted by population: [('Shanghai', 25582000), ('Delhi', 28514000), ('Tokyo', 37400068)]
2. Find and print the city with the highest population.
Accessing the list above:
largest_city = max(cities, key=lambda city: city[1])
print("City with the highest population:", largest_city)
# City with the highest population: ('Tokyo', 37400068)
3. Filter and print cities with a population greater than a specified value
threshold = 30000000
large_cities = [city for city in cities if city[1] > threshold]
print("Cities with population greater than", threshold, ":", large_cities)
# Cities with population greater than 30000000 : [('Tokyo', 37400068)]
Exercise 10
Merging and Splitting Lists: Combine and manipulate multiple lists, then:
- Merge two lists and sort the resulting list in descending order.
- Split a list into two equal parts. If the list has an odd number of elements, the extra element should go to the first part.
- Find the intersection (common elements) of two lists without using set operations.
Solution to Exercise 10
1. Merge two lists and sort the resulting list in descending order
list1 = [1, 3, 5, 7]
list2 = [2, 4, 6, 8]
merged_list = sorted(list1 + list2, reverse=True)
print("Merged and sorted list:", merged_list)
# Merged and sorted list: [8, 7, 6, 5, 4, 3, 2, 1]
2. Split a list into two equal parts. If the list has an odd number of elements, the extra element should go to the first part
original_list = [1, 2, 3, 4, 5, 6, 7]
mid = (len(original_list) + 1) // 2
first_half = original_list[:mid]
second_half = original_list[mid:]
print("First half:", first_half)
# First half: [1, 2, 3, 4]
print("Second half:", second_half)
# Second half: [5, 6, 7]
3. Find the intersection (common elements) of two lists without using set operations
list_a = [1, 2, 3, 4, 5]
list_b = [4, 5, 6, 7, 8]
intersection = [element for element in list_a if element in list_b]
print("Intersection:", intersection)
# Intersection: [4, 5]
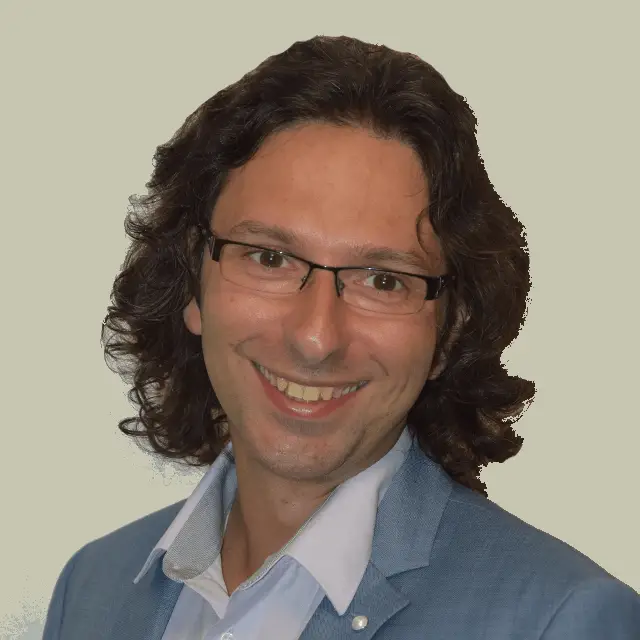
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.