Imagine you are developing a game, and you need a mechanism to determine the impact of a player’s attack on their enemy.
However, this mechanism should not just indicate a successful or failed attack. It should also provide feedback based on the outcome of the attack, such as a successful hit, a critical strike, or a near miss.
This is when Python functions with multiple return paths can help you.
Creating a Python Function with Multiple Return Paths
To understand a function with multiple return paths, you must have a good grip on the basics first. So let’s start by defining a function using the def
keyword.
Begin by giving your function a sensible name and specifying any input parameters it may require.
Let’s continue with our gaming example.
def attack(attacker_level, attack_roll):
# The magic happens here!
You have defined a function that accepts multiple values (they are called parameters). This Python function determines the outcome of an attack in a game based on the level of the attacker and a roll of dice.
Now let’s write the body of the function.
Inside the function, you may use if
, elif
, and else
statements to control the value returned by the function in every scenario. Each condition can terminate the function with a different outcome in the game.
We will add three conditions to handle three different outcomes for the function:
def attack(attacker_level, attack_roll):
threshold = attacker_level * 0.8
if attack_roll >= threshold + 5:
return True, "Critical hit!"
elif attack_roll >= threshold:
return True, "Successful hit!"
else:
return False, "Missed!"
The threshold variable represents the minimum value the attack_roll
must meet or exceed for an attack to be successful.
This function has multiple return paths. It has three return
statements that are executed depending on which if
, elif
, or else
condition is True (boolean check):
- Critical Hit: If the
attack_roll
is greater than or equal tothreshold + 5
, the function returnsTrue, "Critical hit!"
indicating not only that the attack was successful but also achieved a critical impact. - Successful Hit: If the
attack_roll
doesn’t meet the critical hit criteria but is still greater than or equal to thethreshold
, the function returnsTrue, "Successful hit!"
This means the attack was successful, but it wasn’t a critical hit. - Miss: If the
attack_roll
is below thethreshold
, the function returnsFalse, "Missed!"
indicating the attack failed to hit the target.
Let’s test this function:
hit, message = attack(10, 18)
print(hit, message)
# True Critical hit!
hit, message = attack(8, 13)
print(hit, message)
# True Critical hit!
hit, message = attack(5, 4)
print(hit, message)
# True Successful hit!
hit, message = attack(8, 4)
print(hit, message)
# False Missed!
In this example, we have seen how to use multiple return statements in a Python function.
Going Further with Multiple Return Paths
In Python, functions can return more than simply boolean results. Depending on what your function does, you can return several data types.
For example, in a game, instead of simply knowing whether a hit was successful, you might want to know how much damage was delivered. Let’s give it a try:
def calculate_damage(attacker_power, defender_armor):
damage = attacker_power - defender_armor
if damage > 0:
return True, damage
else:
return False, 0
print(calculate_damage(10, 9))
# (True, 1)
print(calculate_damage(5,5))
# (False, 0)
In the code above, you are calculating the damage returned by the function together with a boolean that tells if there was damage or not. Let’s execute the code above and see the output.
Notice that the function returns a Python tuple because it returns more than one value. The first element of the tuple is a boolean and the second element is an integer that represents the damage.
Related article: Do you want to strengthen your foundations in Python functions? Here is a tutorial that will help you review the basics of Python functions.
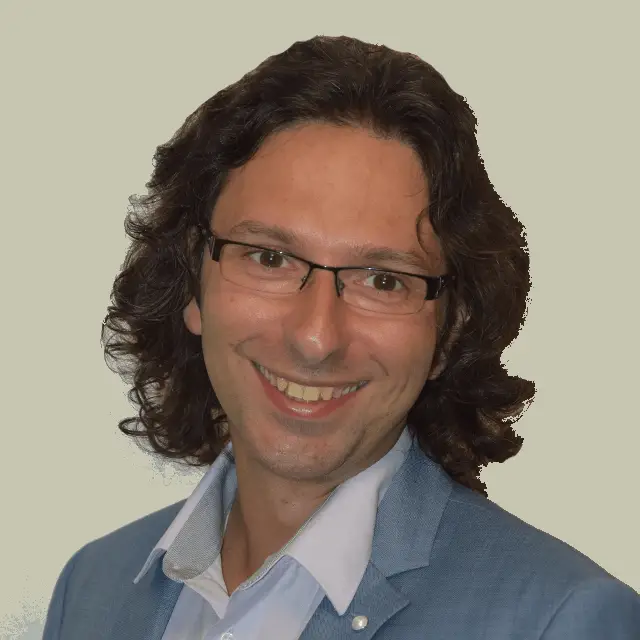
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.