I want to sort a list of dictionaries in my Python program and I’m wondering if I can use a lambda function for that. Is it possible?
Yes, lambda functions allow you to sort lists of dictionaries based on specific dictionary keys.
Suppose you have a list of dictionaries where each dictionary represents a person with the following attributes:
- firstname
- lastname
- age
Let’s say you want to sort this list of dictionaries by the age of each person.
Here’s how you can do this using a lambda function in Python:
# Define a list of dictionaries, each representing a person with a firstname, lastname, and age
people = [
{'firstname':'John', 'lastname':'Ross', 'age': 30},
{'firstname':'Mark', 'lastname':'Green', 'age': 25},
{'firstname':'Jane', 'lastname':'Black', 'age': 43}
]
# Sort the list by the 'age' of each person using a lambda function
sorted_people = sorted(people, key=lambda person: person['age'])
# Print the original list
print("Original list:", people)
# Print the sorted list
print("Sorted by age:", sorted_people)
This is the output of the code snippet above:
Original list: [{'firstname': 'John', 'lastname': 'Ross', 'age': 30}, {'firstname': 'Mark', 'lastname': 'Green', 'age': 25}, {'firstname': 'Jane', 'lastname': 'Black', 'age': 43}]
Sorted by age: [{'firstname': 'Mark', 'lastname': 'Green', 'age': 25}, {'firstname': 'John', 'lastname': 'Ross', 'age': 30}, {'firstname': 'Jane', 'lastname': 'Black', 'age': 43}]
You can see that the dictionaries in the list have been correctly sorted based on the age attribute.
Let’s go through the most important lines of this Python code:
sorted(people, key=lambda person: person['age'])
: This line sorts the listpeople
. Thesorted()
function returns a new sorted list. Thekey
argument is a lambda functionlambda person: person['age']
, which specifies that the sorting should be based on the age attribute in each dictionary.- The original list
people
remains unchanged after the sorting operation, as thesorted()
built-in function does not modify the list in place but instead returns a new sorted list. - The print statements are used to display the original list and the sorted list. This allows you to see the difference and confirm that the sorting logic is correct.
By using a lambda function in the sorted()
function, you can sort complex data structures such as a list of dictionaries, and make your code easy to read.
Sorting a List in Reverse Order Using a Lambda Function
Sorting a list of dictionaries in reverse order using a lambda is straightforward. This is an extension of the sorting technique you have seen in the previous paragraph.
By passing the reverse=True
argument to the sorted()
function, you can invert the default sorting order. This is useful when you want to sort data in descending order. For example, to arrange items from the highest to the lowest value (imagine you are writing code for an eCommerce store and you want users to be able to sort items by price).
The lambda function will remain the same, but you will add the reverse=True
argument to the sorted()
function to achieve reverse sorting. Here’s the updated code:
people = [
{'firstname':'John', 'lastname':'Ross', 'age': 30},
{'firstname':'Mark', 'lastname':'Green', 'age': 25},
{'firstname':'Jane', 'lastname':'Black', 'age': 43}
]
# Sort the list by the 'age' of each person in reverse order using a lambda function
sorted_people_descending = sorted(people, key=lambda person: person['age'], reverse=True)
# Print the original list
print("Original list:", people)
# Print the list sorted in reverse order
print("Sorted by age in reverse order:", sorted_people_descending)
The output confirms that the list has been sorted in reverse order based on the age:
Original list: [{'firstname': 'John', 'lastname': 'Ross', 'age': 30}, {'firstname': 'Mark', 'lastname': 'Green', 'age': 25}, {'firstname': 'Jane', 'lastname': 'Black', 'age': 43}]
Sorted by age in reverse order: [{'firstname': 'Jane', 'lastname': 'Black', 'age': 43}, {'firstname': 'John', 'lastname': 'Ross', 'age': 30}, {'firstname': 'Mark', 'lastname': 'Green', 'age': 25}]
Here is the main difference from the code in the previous paragraph:
sorted(people, key=lambda person: person['age'], reverse=True)
: This line sorts the list people
based on the age in reverse order. The lambda function lambda person: person['age']
specifies the key for sorting, and reverse=True
inverts the sorting order.
This example demonstrates the flexibility of lambda functions in sorting operations. Lambdas allow for both ascending and descending order sorting with minimal changes to your code.
Sorting Lists of Dictionaries by Multiple Attributes Using Lambdas
Sorting a list of dictionaries in Python based on multiple attributes is also possible. By using a lambda function within the sorted()
function, you can provide multiple sorting criteria, and order data based on a primary attribute, and then a secondary attribute in case of ties.
This can be useful when sorting by a single attribute is not sufficient to achieve the order you desire.
In the example of code, you will use the same dictionary structure for people used before. If you have several people of the same age, you might want to sort the list first by age and then by firstname.
Let’s see this in practice. Add a few more dictionaries to the list to have people with the same age value.
# List of dictionaries with some people having the same age
people = [
{'firstname':'John', 'lastname':'Ross', 'age': 30},
{'firstname':'Mark', 'lastname':'Green', 'age': 25},
{'firstname':'Jane', 'lastname':'Black', 'age': 43},
{'firstname': 'David', 'lastname':'White', 'age': 30}, # Same age as John
{'firstname': 'Eve', 'lastname':'Red', 'age': 25} # Same age as Mark
]
# Sorting the list first by age, then by firstname
sorted_people = sorted(people, key=lambda person: (person['age'], person['firstname']))
# Displaying the sorted list
print("Sorted by age and then firstname:", sorted_people)
Have a look at the sorted list. Sorting based on two attributes works fine:
Sorted by age and then firstname: [{'firstname': 'Eve', 'lastname': 'Red', 'age': 25}, {'firstname': 'Mark', 'lastname': 'Green', 'age': 25}, {'firstname': 'David', 'lastname': 'White', 'age': 30}, {'firstname': 'John', 'lastname': 'Ross', 'age': 30}, {'firstname': 'Jane', 'lastname': 'Black', 'age': 43}]
In this code, the lambda function lambda person: (person['age'], person['firstname'])
is used as the sorting key.
The tuple (person['age'], person['firstname'])
specifies that the list should be sorted by age in the first instance, and then by firstname in case of age ties.
In conclusion, Python’s ability to sort lists of dictionaries using lambda functions makes data manipulation very easy and clear to read. Whether it’s sorting based on a single attribute, in reverse order, or by multiple attributes.
By mastering these sorting techniques, you can work with any data structure. This makes Python an invaluable tool for you as a software developer.
Related article: Do you want to learn more about lambda functions? If you haven’t already, test your knowledge with the CodeFatherTech tutorial about Python lambda functions.
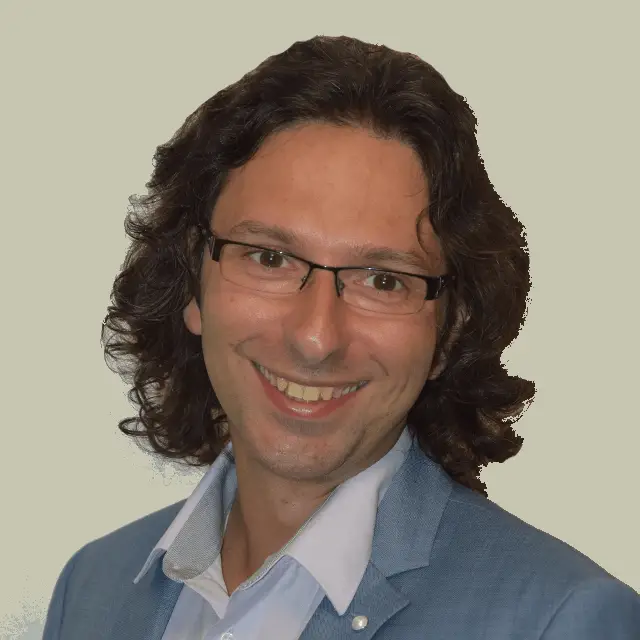
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.