Are you trying to sort a list of strings by length with Python and don’t know how? This article shows you how with clear code examples you can follow and reuse.
Python’s built-in functions support custom sorting criteria. This is the perfect choice to solve this problem whether you are dealing with a list of words, sentences, or any list of strings.
Let’s find out how to sort lists of strings with Python arranging them based on their length.
How to Sort a List of Strings By Length in Python
Let’s start with an example that shows one of the simplest ways to sort a list in Python.
To sort a list you can use Python’s sorted() built-in function that takes the list to be sorted as an argument and returns the sorted list.
Here is an example. Open the Python shell or your favourite IDE so you can test the code while going through this tutorial:
>>> planets = ['saturn', 'earth', 'mars', 'jupiter']
>>> sorted(planets)
['earth', 'jupiter', 'mars', 'saturn']
As you can see the sorted() function returns a new list sorted in alphabetical order.
Now, let’s say you want to order the list based on different criteria, for example, the length of each word.
To sort a list of strings by length you can pass the argument ‘key’ to the sorted() function. This argument allows you to apply a function to the elements of the list before comparing them as part of the sorting process.
Let’s pass the len() function as the key argument:
>>> sorted(planets, key=len)
['mars', 'earth', 'saturn', 'jupiter']
We have used the len() built-in function as a sorting criteria. That’s why the strings are sorted from the shortest to the longest (ascending order).
Sorting a List of Strings By Length in Reverse Order
How can you sort a list of strings based on the length of the strings but in descending order? You can pass an additional argument to the sorted() function: reverse=True
.
Let’s test this in the Python shell:
>>> sorted(planets, key=len, reverse=True)
['jupiter', 'saturn', 'earth', 'mars']
You can see that you have sorted the list of strings in descending order based on the length of the strings.
Practical Applications of Sorting a List of Strings Using the Length as Criteria
Sorting a list according to the length of the elements (strings) has several practical applications across different domains. Three examples are:
- Natural Language Processing (NLP): In text analysis and processing, sorting words or sentences by length can help in tasks like summarization and text analysis. For instance, when analyzing text data, longer words might be specific and informative. On the other side, shorter words could be common and less informative (e.g. conjunctions or prepositions).
- Game Development: In puzzles or word games, sorting words by length can be a good way to progressively increase the difficulty of the game.
- Data Cleanup in Machine Learning: During the preprocessing phase in machine learning, sorting based on the length of strings can help identify anomalies. Examples of anomalies could be unusually long or short entries in text data.
These applications show how important sorting strings by length can be, demonstrating its utility across multiple industries.
Conclusion
You have seen that sorting a list of strings by length in Python is not just a programming exercise. It is also a practical tool with a wide range of applications.
By mastering this skill, you have added a valuable asset to your Python expertise. Remember, the key to being a very good developer is also to know the approach to use to solve common problems.
Related article: to deepen your knowledge about sorting in Python, have a look at how to use Python lambdas to sort a list of dictionaries.
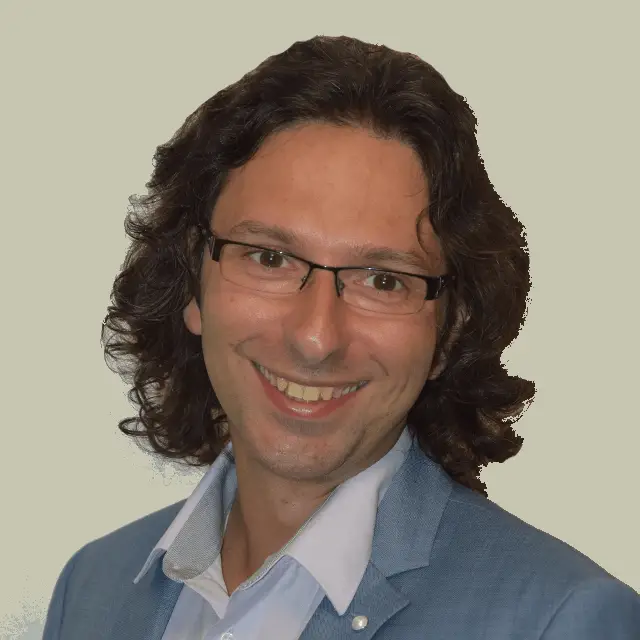
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.