You can convert a list of strings to a list of integers in Python with a for loop that goes through each item in the list of strings, converts it into an int, and appends it to a new list. A more concise alternative is to use a list comprehension. A third option is using the map() function to convert each string in the list to an int and then pass the output of the map() function to the list() function.
Let’s see how these different implementations work in practice!
Convert a List of Strings to a List of Integers with a For Loop
We will start with the most obvious approach that uses a for loop to go through each element of the list of strings, converts it to an int, and then appends that number to an empty list.
When using this approach, you create a new empty list outside the for loop. This empty list will be our final list of integers.
list_of_strings = ['1', '5', '7', '45', '67']
list_of_ints = []
for item in list_of_strings:
list_of_ints.append(int(item))
print(list_of_ints)
The variable list_of_strings
is a list because it starts and ends with square brackets. It’s a list of strings because every item in the list is a string (it’s enclosed within single quotes).
In the first line of code, we create the list of strings. In the second line of code, we create an empty list that will be our final list of integers.
Then we write a for loop that goes through each element of the list of strings and converts each of them to an integer using the Python int() built-in function.
After converting each string in the list to an int we add the integer to the list_of_ints
using the list append() method.
In the last line, we use the print() function to print the final list of integers. Execute this code and confirm you can see the same output:
[1, 5, 7, 45, 67]
Let’s explore a second way to convert lists of strings to ints in Python.
Convert List of Strings to List of Ints with a Python List Comprehension
Another way to convert lists of strings to lists of ints in Python is by using a list comprehension. You will see that with this approach you can reduce the length of our Python code to a single line.
The first two differences with this approach compared to the previous one are that you don’t have to create a new empty list and you don’t need a for loop.
list_of_strings = ['1', '5', '7', '45', '67']
list_of_ints = [int(item) for item in list_of_strings]
print(list_of_ints)
The internal logic of a list comprehension replaces the logic previously implemented using a for loop.
If you are just getting started with Python it might take you some time to get your head around the syntax of a list comprehension. Don’t worry, it’s a very common challenge that many Python developers experience.
Once again confirm that the output of the Python code executed on your computer matches the output below:
[1, 5, 7, 45, 67]
Let’s move to the third approach.
Converting a List of Strings to Integers Using the Map() and List() Functions
This approach is more complex than the previous ones so I wouldn’t necessarily use it in a real-world scenario. At the same time, it’s a way to learn all the options you have available with Python.
First of all, we use the map() function to convert each string in the list into an int.
map(int, list_of_strings)
As you can see, the map() function takes another function as the first argument. In this example the function we pass to the map() function as the first argument is the int() function. This function is applied to every item in the list (or iterable) passed as a second argument to map().
Let’s print the output of the map() function to see what we get back:
print(map(int, list_of_strings))
[output]
<map object at 0x1050c74c0>
The map() function returns a map() object.
So, how can we get back a list of ints?
We have to pass the object returned by the map() function to the Python list() built-in function as you can see below.
list_of_strings = ['1', '5', '7', '45', '67']
list_of_ints = list(map(int, list_of_strings))
print(list_of_ints)
The output is correct:
[1, 5, 7, 45, 67]
Using a While Loop to Convert a List of Strings to a List of Integers
We have another way to convert a list of strings to ints in Python!
You can use a while loop. The principle is similar to the for loop. We use the while loop to:
- Go through each item in the list of strings.
- Convert that item to an integer.
- Use the list append() method to add the integer at the end of the new list of ints we create outside of the while loop.
Here is the Python code for this approach:
list_of_strings = ['1', '5', '7', '45', '67']
list_of_ints = []
index = 0
while index < len(list_of_strings):
list_of_ints.append(int(list_of_strings[index]))
index += 1
print(list_of_ints)
The main difference compared to the approach using the for loop is that we create the variable index
outside the while loop.
You set the initial value of the variable index
to zero and then increase its value by one at every iteration of the while loop. When the value of the index becomes 5 the condition of the while loop becomes false and this terminates the execution of the while loop.
The condition of the while loop becomes false because the length of the list_of_strings
is 5:
list_of_strings = ['1', '5', '7', '45', '67']
print(len(list_of_strings))
[output]
5
The result of the code using the while loop is also correct:
[1, 5, 7, 45, 67]
Conclusion
In this Python tutorial, we have seen multiple ways to convert a list of strings to ints in Python.
As we have seen, you can use a for loop or while loop to iterate explicitly through the list of strings. Alternatives to these approaches are list comprehensions or the map() function.
Well done for improving your Python skills!
Related articles: one of the ways we have seen to generate a list of ints is list comprehensions. To familiarise yourself with them go through the CodeFatherTech tutorial about Python list comprehensions.
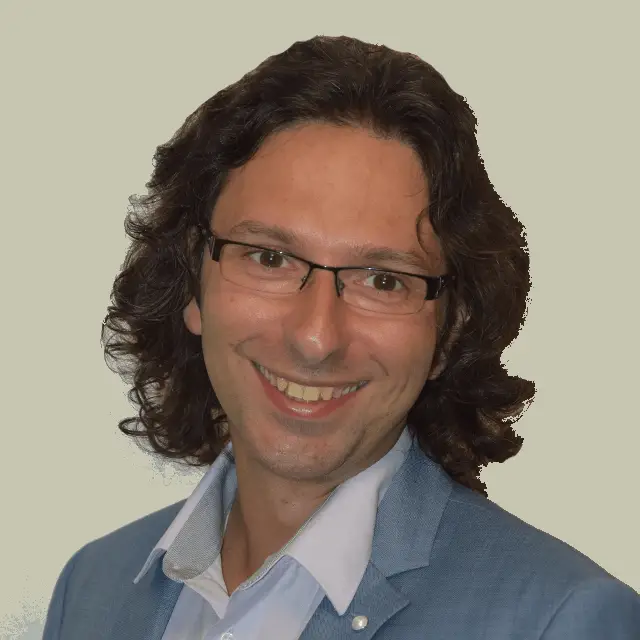
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.