Knowing how to create a list of random numbers can be very useful when writing Python programs for testing, gaming, or simulations.
In this tutorial, we will go through a few techniques to generate a list of random numbers with Python.
Generate a List of Random Integers Using the Random Built-in Module
You can generate a list of random numbers by simply putting together a function provided by Python’s random module with a while loop.
The following code uses the randint()
function of Python’s random built-in module and a while loop to generate a list of random integers:
>>> random_integers = []
>>> while len(random_integers) < 10:
... random_integers.append(random.randint(1, 100))
...
>>> print(random_integers)
[5, 64, 39, 78, 85, 10, 69, 11, 20, 50]
The code inside the while loop is executed as long as the list random_numbers
has less than 10 numbers.
In the output of the print()
function you can see the final list of random numbers.
The next section shows a way to do this with less code…
Create a List of Random Integers Using a List Comprehension
You can write Python code that is a lot more concise than the previous one by using a list comprehension that generates a list of random integers.
The logic of the following line of code is identical to the logic previously implemented using the while loop. It creates a list of 10 random integers, each between 1 and 100.
random_integers = [random.randint(1, 100) for _ in range(10)]
The code for _ in range(10)
creates an iterable sequence of 10 numbers, from 0 to 9. The underscore _
is a common Python convention for a variable name when the variable itself is not going to be used.
Let’s confirm that the output of this code is correct by using the Python shell:
>>> random_integers = [random.randint(1, 100) for _ in range(10)]
>>> print(random_integers)
[12, 48, 89, 31, 87, 70, 88, 91, 10, 58]
The code you have written is correct!
Create a List of Random Floats Using a List Comprehension
You can also use a list comprehension to generate a list of floats.
The only difference compared to generating a list of integers is to replace the randint()
function with the random()
function.
random_floats = [random.random() for _ in range(10)]
Let’s verify the output of this code:
>>> random_floats = [random.random() for _ in range(10)]
>>> print(random_floats)
[0.010880145031827326, 0.38366261395011514, 0.8639732752346184, 0.3354153700807573, 0.682066532960149, 0.3286131067341429, 0.6954843256590966, 0.8733497107522957, 0.4832353071781291, 0.799746658898504]
And here they are, 10 random floats.
Generate a List of Random Numbers Using NumPy
You can also generate a list of random numbers using the NumPy library.
Below you can see some of the methods in the NumPy random module:
>>> import numpy as np
>>> np.random.ra
np.random.rand( np.random.randn( np.random.random_integers( np.random.ranf(
np.random.randint( np.random.random( np.random.random_sample( np.random.rayleigh(
To generate a list of random integers you can use the NumPy randint()
function. It has the same name as the function you have seen previously in Python’s built-in random module but it’s more flexible.
>>> np.random.randint(1, 10, 10)
array([8, 2, 6, 4, 6, 4, 2, 1, 4, 9])
We have passed three arguments to the numpy.random.randint()
function:
- First argument: specifies the start (inclusive) of the range in which the random integers will be generated.
- Second argument: specifies the end (exclusive) of the range in which the random integers will be generated.
- Third argument: used to return a NumPy array of
n
elements, in this case, 10.
As the third argument, you can also pass a tuple of integers. Here is what happens when you do that:
>>> np.random.randint(1, 10, (5,5))
array([[4, 1, 9, 3, 4],
[7, 1, 8, 1, 2],
[1, 2, 3, 8, 2],
[9, 1, 3, 6, 8],
[9, 9, 4, 8, 6]])
You get back a 5 by 5 multidimensional array (or matrix).
Conclusion
You now have all the knowledge to generate lists of random numbers in Python.
In this tutorial, you also had the opportunity to practice list comprehensions.
Related article: another operation you can find useful in a Python program is to select random numbers from a Python list.
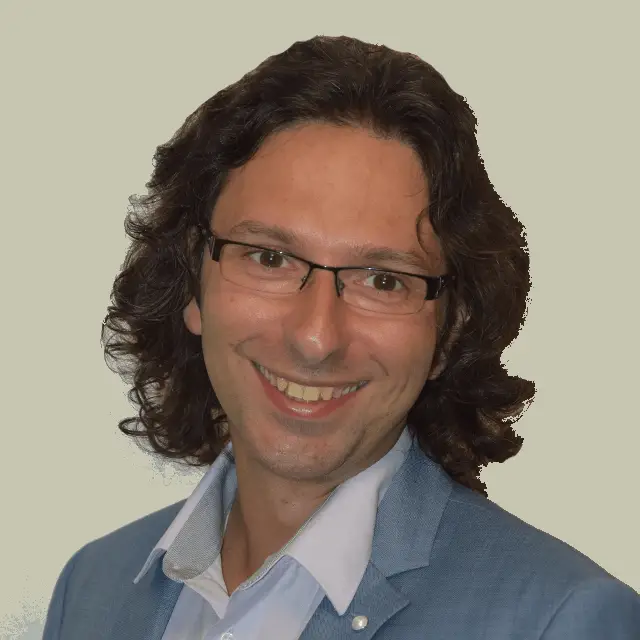
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.