Are you trying to create a random string in your Python program? This tutorial will show you how to write the code to do it, step-by-step.
Let’s get started!
Generate Random Strings in Python Using a List Comprehension
Random string generation involves selecting characters (like letters, digits, and symbols) in a random order to form a string. The randomness in Python can be implemented using the random
module, which provides functions to execute random operations.
Let’s understand the logic to generate a random string step-by-step.
We will start by using the random.choice()
function that randomly selects an element from the list of characters we pass to it:
>>> import random
>>> random.choice(['h', 'e', 'l', 'l', 'o'])
'h'
>>> random.choice(['h', 'e', 'l', 'l', 'o'])
'l'
>>> random.choice(['h', 'e', 'l', 'l', 'o'])
'e'
If you apply a list comprehension to the expression above you can create a list of random characters:
>>> [random.choice(['h', 'e', 'l', 'l', 'o']) for i in range(5)]
['l', 'o', 'h', 'o', 'l']
Now if you apply the string join() method to the list generated before, you obtain a string that contains the 5 random characters:
>>> ''.join([random.choice(['h', 'e', 'l', 'l', 'o']) for i in range(5)])
'holhl'
Note: remember that you use the string join() method to obtain a string from a list.
Now that you know how to think when it comes to generating random strings, keep reading to discover a simpler approach.
Generate Random Strings with the random.choices() Function
There is a simpler way to generate a random string in Python. You can use the random.choices()
function, this approach doesn’t require a list comprehension.
You can generate a random string of 5 characters by simply passing k=5
to the random.choices()
function. Here is an example:
>>> ''.join(random.choices(['h', 'e', 'l', 'l', 'o'], k=5))
'oheol'
Let’s try with a bigger value of the argument k
:
>>> ''.join(random.choices(['h', 'e', 'l', 'l', 'o'], k=20))
'lhloeelohhleelhoeelh'
In the next sections, I will show you how to use the string module and the random module together to generate:
- random numeric strings
- random strings of letters
- random alphanumeric string
Generate a Random Numeric String
Python’s string module provides constants that can be very useful to generate random strings. One of those constants is string.digits
.
The following code creates a random numeric string:
>>> import string
>>> string.digits
'0123456789'
>>> ''.join(random.choices(string.digits, k=20))
'94879244168153375098'
And here you have your random string of numbers.
Generate a Random String of Letters
The string module provides a constant that you can use to generate a random string of letters: string.ascii_letters
.
The following code creates a random string of letters (a mix of uppercase and lowercase letters):
>>> import string
>>> string.ascii_letters
'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
>>> ''.join(random.choices(string.ascii_letters, k=20))
'TwdshFRPhSOVrnPxrBBM'
You can also use string.ascii_lowercase or string.ascii_uppercase if you don’t want to mix uppercase and lowercase letters.
Generate a Random Alphanumeric String
To generate a random alphanumeric string using Python you can concatenate the following two constants available in the string module: string.digits
and string.ascii_letters
.
To concatenate the two string constants you can use the + operator.
>>> import string
>>> all_characters = string.digits + string.ascii_letters
>>> all_characters
'0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
>>> ''.join(random.choices(all_characters, k=20))
'k2cUzC17iQfh5ByY6Oat'
Also, try the same code but this time using the string.printable
constant.
Have a look at what other characters are included in your random string.
Conclusion
After going through this tutorial, you should have a clear idea of how to generate random strings.
If you don’t, go back to the beginning of the tutorial and make sure to execute all the examples of code explained in this tutorial on your computer. The best way to remember is to actually write code.
If you want to learn about possible applications of this knowledge have a look at how to create a password generator in Python.
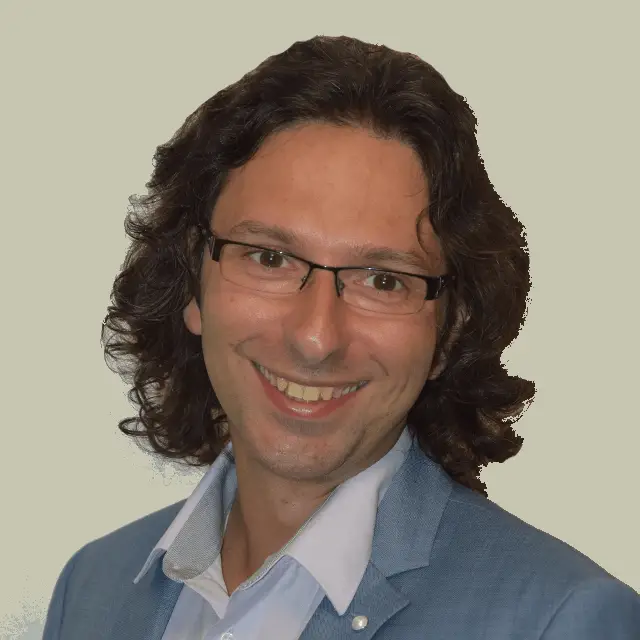
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.