I have noticed that sometimes when users use my Python application they provide values that contain multiple spaces. What is the simplest way to replace those spaces with a single space?
One way to replace multiple spaces with a single space in a string using Python is with the join() and split() string methods used together. You can also achieve the same result using the re.sub() function part of Python’s re module.
We will start with an approach that requires only one line of code!
Also, it doesn’t need any additional Python libraries.
We will use two methods provided by Python strings: join() and split().
To replace multiple spaces with one space in a Python string with join() and split() you can first split the string using the space as the separator character. Then you can take the resulting list of strings and use the string join() method to create the final string by specifying only one space as the separator.
Let’s see how this works in practice using the Python shell:
>>> text = "Hello, from CodeFatherTech! Let's learn Python!"
>>> text.split()
['Hello,', 'from', 'CodeFatherTech!', "Let's", 'learn', 'Python!']
Firstly, by using the string split() method we convert the initial string that contains multiple spaces into a list of strings.
This helps remove all the whitespaces between each substring in the initial string because we are using the space as the separator for the split() method.
Note: the space is the default separator used by the split() method.
The next step is to use the join() method. This creates a final string that uses only one space as the separator between each word.
>>> split_text = text.split()
>>> ' '.join(split_text)
"Hello, from CodeFatherTech! Let's learn Python!"
Now write the full code that we have tested in the Python shell:
text = "Hello, from CodeFatherTech! Let's learn Python!"
text_with_single_space = ' '.join(text.split())
print(text_with_single_space)
As a result, when you execute this code you will see the following output:
Hello, from CodeFatherTech! Let's learn Python!
To recap, the split() method splits the initial string into a list of words. Then the join() method combines these strings back into a final string, inserting a single space between each word.
Replace Multiple Spaces with a Single Space in a String Using re.sub()
The second method we will go through uses Python’s regular expressions module: the re module.
To replace multiple whitespaces in a string with a single space you can use the re.sub() function that belongs to Python’s re module. Pass a regular expression that describes multiple spaces to re.sub(), this function will then allow you to replace them with a single space.
Below you can see the basic syntax of the re.sub() function. It’s important to understand it before you can use it correctly in your program:
re.sub(pattern, repl, string)
In the syntax above, occurrences of the pattern specified (first argument) are replaced by the string repl (second argument), in the string provided (third argument).
Let’s see how this works with a simple example of code. And remember that the first thing to do is to import the re module.
import re
text = "Hello, from CodeFatherTech! Let's learn Python!"
text_with_single_space = re.sub(' +', ' ', text)
print(text_with_single_space)
The pattern ‘ +’ represents one or more consecutive whitespace characters. Those spaces are replaced with a single space, the second argument we passed to the re.sub() function in the code above.
Note that the function re.sub() is not just useful to replace multiple whitespace characters. It is very flexible as it can replace any pattern defined by a regular expression.
This makes it a powerful tool for string manipulation tasks.
How Do You Get Rid of Double Spaces in a Python String?
To get rid of double spaces in a Python string you can use the re.sub() function of the regular expression (re) module. A regular expression you can use is ‘ {2}’ which allows you to specify the number of whitespaces to match.
Let’s test this by writing some code in the Python shell.
>>> text = "Hello, from CodeFatherTech! Let's learn Python!"
You can see two whitespaces in two different places of the text string variable.
Import the re module in the Python shell and pass the following regex to the re.sub() function: ‘ {2}’.
>>> import re
>>> re.sub(' {2}', ' ', text)
"Hello, from CodeFatherTech! Let's learn Python!"
The string returned by re.sub() doesn’t contain double spaces anymore. The double spaces have been replaced by single spaces.
You can obtain the same result with two other regex patterns:
>>> re.sub(' ', ' ', text)
"Hello, from CodeFatherTech! Let's learn Python!"
>>> re.sub('\s+', ' ', text)
"Hello, from CodeFatherTech! Let's learn Python!"
The first pattern is made of two consecutive whitespaces. It’s not a very handy pattern considering that you have to manually type the correct number of whitespaces in the pattern.
The second pattern allows matching one or more consecutive whitespace characters. It’s one of the most flexible patterns we have seen so far.
What is the RegEx for One or More Spaces in Python?
So far we have seen that one of the ways to replace multiple spaces in a string is by using a regex in Python. The regex gets passed as one of the arguments to the re.sub() function.
In this section, we will go through some simple regular expressions to identify one or more space characters.
This can help you get familiar with regular expressions that often can be difficult to read. This is the case especially if you are seeing them for the first time.
The following table shows some examples of regular expressions that can be used with re.sub():
Regular expression | Description |
---|---|
‘ ‘ | Regex to match exactly one space. |
‘ {2}’ | Regex to match two consecutive spaces. Using the {n} quantifier helps because otherwise you would have to type n consecutive spaces in the expression and this would make the expression hard to read and prone to errors. |
‘ +’ ‘\s+’ | Two regex to match one or more whitespace characters. |
‘\s’ | Regex to match any whitespace character including tabs and newline characters. |
By going through the regular expression explained in the table above you can learn different ways to match one, two, or multiple whitespaces in a string.
Conclusion
Now you know two ways to replace multiple space characters with a single space in a string in Python.
The advantage of the approach with split() and join() is that you don’t have to import an external module.
At the same time, using a regular expression can make your code more powerful. That’s because it gives you flexibility in identifying a variety of patterns in strings.
For more Python tips and tricks, check out all the CodeFatherTech tutorials.
Related article: Do you want to dig deeper into how to split strings? Have a look at the step-by-step CodeFatherTech tutorial on how to split strings in Python.
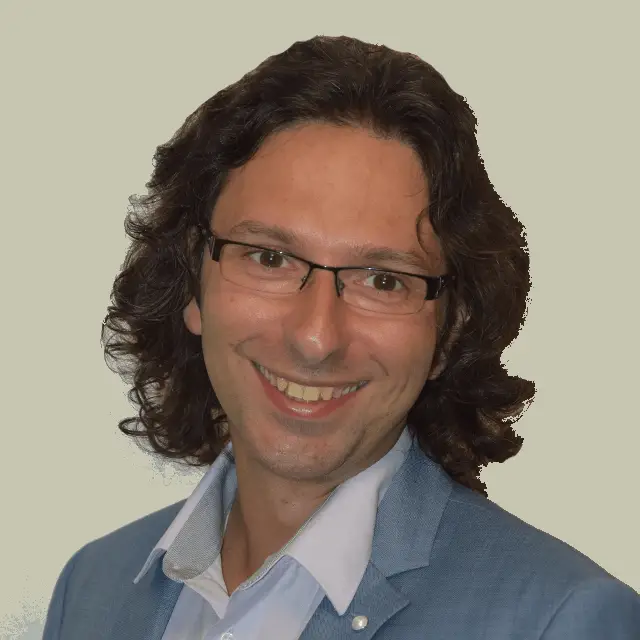
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.