Do you want to learn how to remove numbers from a string in Python? This tutorial will show you how to do it with examples of code.
To remove numbers from a string in Python you can use different techniques. The first option is to use a for loop that goes through every character in the string and creates a new string made of characters that are not numbers. You can achieve the same result using the string join() method together with a list comprehension. A third option is to use the sub() function of Python’s re (Regular expression) module.
The examples in this tutorial will show you multiple ways to remove numbers from a string using Python.
By the end of this tutorial, you will be able to remove all numbers from a string with the approach you prefer the most.
1. How to Remove Numbers From a String Using a Python For Loop
In Python, you can use a for loop to go through every character in a string. Here is an example:
message = "Today it's 25 degrees in Rome"
for character in message:
print(character)
From the output of this code you can see how the for loop goes through one character of the string at a time and prints that character:
T
o
d
a
y
i
t
'
s
2
5
d
e
g
r
e
e
s
i
n
R
o
m
e
Now add a line of code to the body of the for loop. This line of code will check if a character is a digit. If it’s not a digit it appends that character to a new string.
To check if a character is a number or not you can use the isdigit() string method.
The isdigit() string method returns True if a given character is a number and False if a character is not a number.
Below you can see how the isdigit() method works for a number, letter, and space character:
>>> '5'.isdigit()
True
>>> 'a'.isdigit()
False
>>> ' '.isdigit()
False
Let’s update the previous code that uses the for loop and add a check based on the isdigit() method.
message = "Today it's 25 degrees in Rome"
updated_message = ""
for character in message:
if not character.isdigit():
updated_message += character
print(updated_message)
Notice that we have defined the new empty string outside of the for loop. At every iteration of the for loop, you append a character to the updated_message string if that character is not a number.
You can see that the if statement inside the for loop is using the “not logical operator” because in the new string, we only want characters that are not numbers.
The new string updated_message doesn’t contain any numbers:
Today it's degrees in Rome
With this approach, we have learned how to iterate through the string that contains numbers and generate a new string without numbers. This approach works but it requires a few lines of code.
In the next section, you will learn how to obtain the same result with fewer lines of Python code.
2. Remove Numbers From a String in Python Using the join() Method and a List Comprehension
Let’s improve the Python code we created in the previous section.
By using the string join() method and a list comprehension we can make our Python code a lot more concise.
message = "Today it's 25 degrees in Rome"
updated_message = "".join([character for character in message if not character.isdigit()])
print(updated_message)
First of all, we will confirm that the output is correct:
Today it's degrees in Rome
Yes, the output is correct!
It’s great that we have managed to remove numbers from a string with a single line of code. At the same time, if this is the first time you see this type of syntax, it can get a bit confusing.
There’s quite a lot happening in a single line of code!
Let’s dissect the snippet of code above in the Python shell and start by looking at what the list comprehension returns.
>>> message = "Today it's 25 degrees in Rome"
>>> [character for character in message if not character.isdigit()]
['T', 'o', 'd', 'a', 'y', ' ', 'i', 't', "'", 's', ' ', ' ', 'd', 'e', 'g', 'r', 'e', 'e', 's', ' ', 'i', 'n', ' ', 'R', 'o', 'm', 'e']
You can see that the list comprehension returns a list in which every element is a character of the original string without including any numbers.
We can then use the Python string join() method applied to the empty string as a separator, to obtain the final string.
>>> "".join(['T', 'o', 'd', 'a', 'y', ' ', 'i', 't', "'", 's', ' ', ' ', 'd', 'e', 'g', 'r', 'e', 'e', 's', ' ', 'i', 'n', ' ', 'R', 'o', 'm', 'e'])
"Today it's degrees in Rome"
This list comprehension allows writing the same logic of the for loop in a single line.
You can see that the logic “if not character.isdigit()” that was previously in the first line of the body of the for loop, is now at the end of the list comprehension.
3. Remove Numbers From String Using the filter() Built-in Function and a Lambda Function
Let’s go through a different approach…
You can remove every number from a string using Python’s filter() built-in function together with a lambda function.
The filter function takes as its first argument another function that detects the characters in the string that are not numbers and as its second argument the original string (in this example the message variable).
The function we will pass as the first argument to the filter() function is a lambda function.
What will this lambda function do?
Given a character, the lambda function will return True if that character is not a number and False if that character is a number. This logic will be applied to every character in the original string by passing the lambda to the filter() function.
I will show you how the lambda works in isolation before we use it together with the filter() function. I want to make sure it’s clear to you what this lambda does:
>>> lambda character: not character.isdigit()
<function <lambda> at 0x104a475b0>
>>> (lambda character: not character.isdigit())('5')
False
>>> (lambda character: not character.isdigit())('a')
True
>>> (lambda character: not character.isdigit())(' ')
True
In the first line, you can see the definition of the lambda function. The next three expressions show what the lambda returns when we pass a number, letter, and space character to it.
Now, let’s see how the filter() function and the lambda function work together:
>>> message = "Today it's 25 degrees in Rome"
>>> filter(lambda character: not character.isdigit(), message)
<filter object at 0x104a48940>
As you can see from the output above the filter() function returns a filter object.
What can we do with this object?
We can pass the output of the filter() function to the string join() method and use the empty character as the separator.
>>> "".join(filter(lambda character: not character.isdigit(), message))
"Today it's degrees in Rome"
Et voilà, we get back the original string without numbers!
4. Using Python’s re Module to Remove All Numbers From a String Using Regex
A different technique from the ones we have seen so far also allows removing all numbers from a string. This approach uses Python’s re module which is a module to handle regular expressions.
The re module provides the re.sub() function that you can use to substitute numbers in a string with empty characters.
The following code shows that you can use the re.sub() function to replace characters identified by a numeric pattern (first argument), with an empty character (second argument), in the original string (third argument).
Notice that the first difference compared to the previous three approaches is that on the first line, we are importing the re module using an import statement.
import re
message = "Today it's 25 degrees in Rome"
updated_message = re.sub(r'\d', '', message)
print(updated_message)
The expression r’\d’ is a regular expression (regex) pattern used in Python to match any single digit in a string.
This the the output of our code:
Today it's degrees in Rome
It is correct!
Conclusion
In this Python tutorial, we went through four different ways to remove numbers from a string in Python.
We started with a basic approach using a for loop and then moved to more concise approaches based on the string join() method, list comprehensions, filter() built-in function, lambda function, and regular expressions.
Make sure you understand how every implementation works so you can use the one you prefer in your Python code without any doubts.
Related article: We have used lambdas as a filtering function. Learn more about lambdas as they can be a challenging topic when you are getting started with Python. Go through the CodeFatherTech tutorial about Python lambdas.
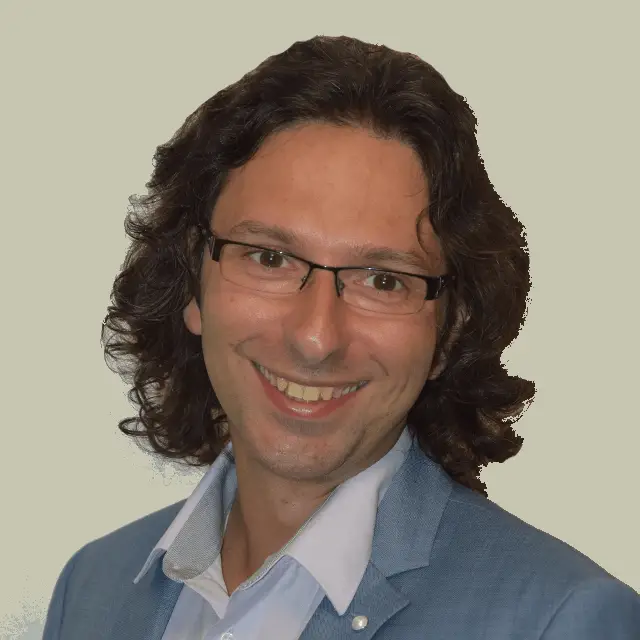
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.