A common error you could encounter while writing Python code is “list indices must be integers or slices, not str”. Here you will learn how to fix it.
The Python error “list indices must be integers or slices, not str” occurs when you try to access an element of a list using a string as an index instead of an integer. As the error explains, when accessing list elements you must use integers or slices.
The examples in this tutorial will show you when this error happens and what you can do to fix it. We will also cover a scenario in which the cause of the error is not so obvious.
Let’s get this Python error fixed!
Cause 1: Accidentally Using a String as Index To Access Python List
The main reason why you see the Python error “list indices must be integers or slices, not str” is that you are using a string as an index to access an element of a list.
As you know, to access list elements you should pass the index of the element to access after the list variable between square brackets as shown below:
numbers = [1, 2, 3, 4, 5]
print(numbers[1])
[output]
2
Let’s see what happens if you accidentally pass a string as an index instead:
print(numbers['1'])
The Python interpreter raises the TypeError “list indices must be integers or slices, not str” below:
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/list_indices_must_be_integers_or_slices_not_str.py", line 2, in <module>
print(numbers['1'])
TypeError: list indices must be integers or slices, not str
The traceback also shows the line of code that is causing the error. This helps you in the process of identifying and fixing this error.
Solution 1: Make Sure to Use an Integer Index to Access an Element of a List
To solve the Python TypeError we have seen in the previous section, you have to replace the string between square brackets with a valid integer index.
An index is an integer that starts with the value zero to access the first element of a list. The index with value 1 allows accessing the second element of the list, and so on.
For example, the following Python code shows how to correctly access the first element of the list numbers:
numbers = [1, 2, 3, 4, 5]
print(numbers[0])
[output]
1
As you can see, an integer is not surrounded by single quotes while a string is surrounded by single quotes (and can also be surrounded by double quotes).
In the next section, we will see that it’s not always straightforward to understand why this Python error occurs.
Cause 2: Forgetting to Convert Strings That Are Used As Indexes
This second scenario in which the error “list indices must be integers or slices, not str” can occur is somehow similar to the first scenario.
At the same time, this is harder to identify because the index you pass to the list comes from the output of Python’s input() function.
It’s a common approach in a Python program, to get user input and then use the value provided by the user in the execution of the program.
Here is an example…
numbers = [1, 2, 3, 4, 5]
index = input("Provide the list index you want to access: ")
print(numbers[index])
From a first look, this Python code looks correct.
Let’s execute it:
Provide the list index you want to access: 1
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/list_indices_must_be_integers_or_slices_not_str.py", line 3, in <module>
print(numbers[index])
TypeError: list indices must be integers or slices, not str
That’s interesting, the code is not working…
After specifying the index 1 when requested by the input() function, the execution of the program continues and Python raises the error “list indices must be integers or slices, not str”.
Why?
After all the value we have provided for the index is 1 and the value 1 is an integer.
Add the following line after the line that calls the input() function. This will allow you to see the data type of the value returned by the input function.
As shown in the code below, to see the data type of a variable you can use Python’s type() built-in function:
print(type(index))
When you execute the program you see that this line prints the following output:
<class 'str'>
This shows you that the output of the input() function is a string and not an integer. This is a standard behavior of the input() function in Python 3.
That’s the reason why Python is complaining about the fact that we are using a string as an index to access an element of our list.
Solution 2: Convert Strings Used As Indexes into Integers
Now it’s time to solve the problem caused by the fact that the input() function returns a string instead of an integer.
To fix this error we can use the int() built-in function to convert the string returned by the input() function into an integer before assigning its value to the variable index.
Here is the updated code that uses the int() built-in function:
numbers = [1, 2, 3, 4, 5]
index = int(input("Provide the list index you want to access: "))
print(type(index))
print(numbers[index])
And now let’s verify the output of the updated code:
Provide the list index you want to access: 1
<class 'int'>
2
This time Python doesn’t raise a TypeError and you can see from the output of the type() function that the index variable is now an integer and not a string anymore.
That’s the reason why the code works correctly and Python doesn’t raise anymore the previous error.
Conclusion
The Python error “list indices must be integers or slices, not str” is quite straightforward to troubleshoot and fix considering that Python provides the line number where the error occurs as part of the traceback.
Sometimes it can be a bit more tricky to understand why a variable you expect to be an integer is a string instead (see the example we have covered when using an index coming from the output of the input() function).
Now that you completed this tutorial, you have the knowledge to fix this Python error!
Related article: Keep learning Python core concepts by going through the CodeFatherTech tutorial about the Python input() function.
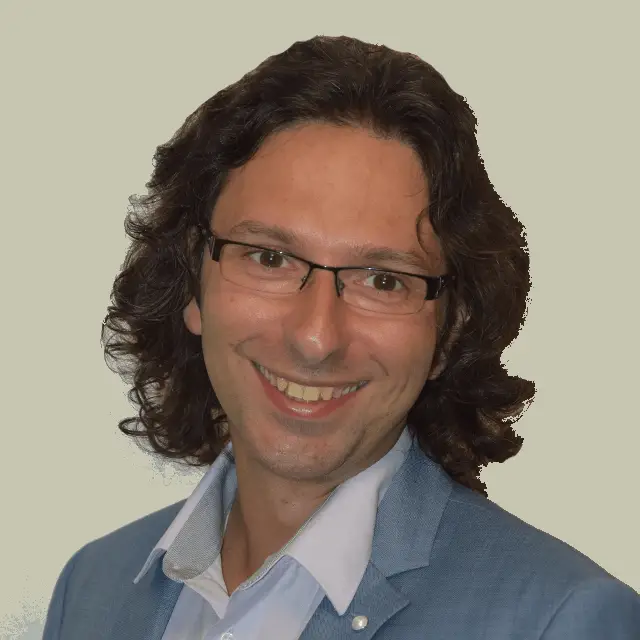
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.