Are you trying to convert a list to a tuple in Python? This tutorial shows how to convert a list into a tuple in Python in different ways
Python lists and tuples are data structures that you can use to store collections of items. The main difference between the two is that lists are mutable and tuples are immutable.
The simplest way to convert a list to a tuple in Python is by using Python’s tuple() built-in function. You can also use a Python for loop or the unpacking operator. The immutability of tuples is one of the reasons why you might want to convert a list into a tuple in your Python program.
Let’s go through a few code examples to make sure converting lists to tuples becomes part of your Python core knowledge!
How Do You Convert a List into a Tuple in Python?
The simplest way to convert a list into a tuple is to use Python’s tuple() built-in function (that is technically a constructor).
This is the simplest way as it just requires a single line of code.
Below you can see an example of converting a list of numbers to a tuple of numbers using the tuple() function:
numbers_list = [1, 2, 3, 4, 5]
numbers_tuple = tuple(numbers_list)
print(numbers_tuple)
The output of the print statement is a tuple:
(1, 2, 3, 4, 5)
You can also see the data type of both collections using the type() function:
numbers_list = [1, 2, 3, 4, 5]
print("The data type of numbers_list is", type(numbers_list))
numbers_tuple = tuple(numbers_list)
print("The data type of numbers_tuple is", type(numbers_tuple))
The output of the print statements shows the ‘list’ and ‘tuple’ data types:
The data type of numbers_list is <class 'list'>
The data type of numbers_tuple is <class 'tuple'>
We have how to convert the list of numbers into a tuple of numbers:
Note: We are including “_list” and “_tuple” in the names of the variables to clearly show which one is the list and which one is the tuple. This is just for teaching purposes. You should not include the data type in the name of variables when writing your Python code.
You could actually write the code in the following way:
numbers = [1, 2, 3, 4, 5]
numbers = tuple(numbers)
print(numbers)
Execute the Python code above and confirm that the variable numbers you get back from the tuple() function is a Python tuple.
Convert a Python List into a Tuple Using a For Loop
Another way to convert a list into a tuple is by using a Python for loop. This approach requires more lines of code compared to the use of the tuple() function.
At the same time going through this implementation, will help you strengthen your Python knowledge.
To convert a list into a tuple using a for loop you can create an empty tuple and then at every iteration of the for loop, you add an item from the list to the new tuple.
Here is what the code using the for loop looks like to start from a list and convert it to a tuple:
numbers_list = [1, 2, 3, 4, 5]
numbers_tuple = ()
for number in numbers_list:
numbers_tuple += (number,)
print(numbers_tuple)
Notice that to insert each item from the list into the tuple we use the following syntax. We specify the item within parentheses followed by a single comma. This is a tuple with a single element:
tuple += (item,)
The output of the code is the following:
(1, 2, 3, 4, 5)
This approach provides more control on the conversion process considering that you could, for example, modify each item coming from the list before adding it to the tuple.
Imagine you want to multiply each number by 2 before adding it to the tuple, you could do the following:
numbers_list = [1, 2, 3, 4, 5]
numbers_tuple = ()
for number in numbers_list:
numbers_tuple += (2*number,)
print(numbers_tuple)
We have updated the line of code inside the for loop to multiply each item in the list by 2 before adding it to the tuple. Below you can see the tuple created with this code:
(2, 4, 6, 8, 10)
Using a for loop to convert a list into a tuple is a flexible approach but it also requires more lines of code than the other two approaches we cover in this tutorial.
Using Unpacking Operator to Convert List to Tuple in Python
You can convert a list into a tuple using Python’s unpacking operator. Using this approach you can convert a list to a tuple with a single line of code.
Here is an example of how to use the unpacking operator to unpack the list elements and create a tuple:
numbers_list = [1, 2, 3, 4, 5]
numbers_tuple = (*numbers_list,)
print(numbers_tuple)
print(type(numbers_tuple))
Here you can learn the syntax used with the unpacking operator. Make sure you remember it so you can use it in the future if you need to.
(*list_to_unpack,)
Let’s confirm that the data structure we have created with the code above is using the tuple data type:
(1, 2, 3, 4, 5)
<class 'tuple'>
Yes, the output of the type() function confirms that the resulting data structure is a tuple.
This approach that uses the unpacking operator is a Pythonic way to convert a list into a tuple. At the same time, it might seem a bit cryptic to those who are not familiar with the unpacking syntax.
Why Do You Convert a List to a Tuple in a Python Program?
Two common reasons why you would convert a list to a tuple when writing a Python program are:
- Working with immutable data: considering that tuples are immutable, using tuples allows you to make sure that existing items in your collections cannot be changed. This can be useful if you have a collection that you want to make sure doesn’t change during the execution of your program.
- Creating dictionary keys with multiple items: tuples can be used as dictionary keys because they are immutable. The same does not apply to Python lists because they are mutable.
Now that you know why you would use tuples you can think about it next time you have to decide if a list is necessarily needed in your Python application. Using a tuple instead might actually be enough.
Conclusion
In this article, you have learned three different ways to convert a Python list to a tuple.
Your preferred approach will depend on a couple of factors:
- If you need to apply a transformation function to each item in the list before adding it to the tuple.
- If developers in your team are familiar with unpacking a list in Python.
The simplest and most straightforward approach we have seen is the use of the tuple() built-in function.
Related article: Do you want to learn more about tuples? Have a look at the CodeFatherTech step-by-step guide about Python tuples!
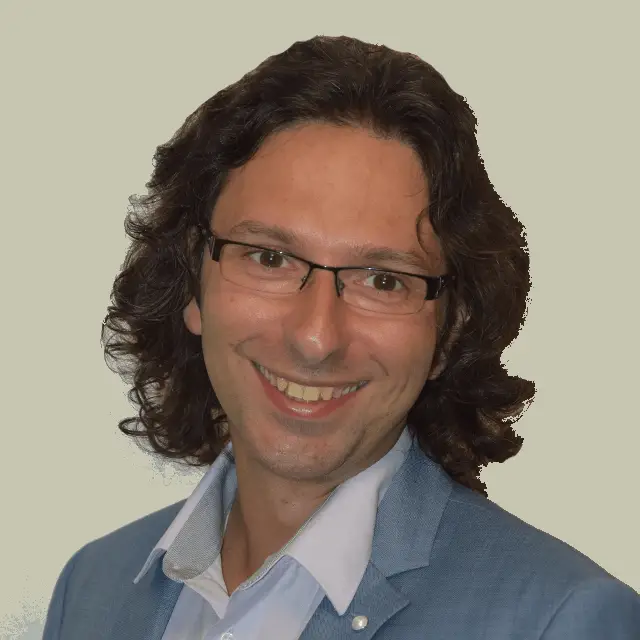
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.