Do you have to sort a list of tuples in your Python program? Are you trying to figure out if you should use sorted() or sort()? You are in the right place.
The first way to sort a list of tuples is by using the Python sorted() function that returns a new sorted list of tuples. An alternative is the list sort() method that doesn’t return a new list but updates the original list in-place. Both sorted() and sort() accept the optional arguments key and reverse to provide additional sorting options.
We will go through several examples that will show you how to use sorted() and sort().
Let’s get started!
How to Sort a List of Tuples with the Sorted Function
Let’s take the following list of tuples:
numbers = [(2,5,3), (3,1,2), (0,0,1)]
I want to sort the tuples in the list based on the first item in each tuple.
Python provides a function called sorted() that can be used to sort items in iterables.
Considering that a list is an iterable I would expect the sorted() function to be able to sort our list of tuples.
Let’s find out:
>>> print(sorted(numbers))
[(0, 0, 1), (2, 5, 3), (3, 1, 2)]
As you can see the order of the tuples in the list has changed.
By default, the sorted() function sorts the tuples based on the first item in each tuple. That’s why the first tuple in the sorted list starts with 0, the second with 2, and the third with 3.
I wonder what happens if the first element of each tuple is the same:
>>> numbers = [(2,5,3), (2,1,2), (2,0,1)]
>>> print(sorted(numbers))
[(2, 0, 1), (2, 1, 2), (2, 5, 3)]
The next sorting criteria used by the sorted() function is the second element of each tuple.
And if all the tuples have the same second element?
>>> numbers = [(2,5,3), (2,5,2), (2,5,1)]
>>> print(sorted(numbers))
[(2, 5, 1), (2, 5, 2), (2, 5, 3)]
This time the tuples in the list are sorted based on the third element once they have been sorted based on the first and the second elements in each tuple that in this case are the same.
How Can You Sort a List of Tuples by the Second Element?
By default, the Python sorted() function sorts a list of tuples based on the first item of the tuple.
But, what if you want to sort the tuples in the list based on the second item in each tuple?
To do that you can pass an optional argument called key to the sorted() function.
The key argument allows you to specify a function of one argument that returns a comparison key used to compare the tuples in the list.
In other words, you can use the argument key to provide a function that defines the element in the tuple to be used as criteria to sort the tuples in the list.
A quick way to provide a function for the key argument is with a lambda function.
For example, the following lambda applied to a tuple returns the second item of the tuple:
lambda data: data[1]
Here is how you can test this lambda function in the Python shell by passing a tuple to it:
>>> (lambda data: data[1])((3, 4, 6))
4
Note: Remember that data[1] returns the second item of the tuple because the index for Python sequences starts from zero.
Now we can pass this lambda function as the optional key argument of the sorted function:
>>> numbers = [(1,7,3), (4,9,6), (7,3,9)]
>>> print(sorted(numbers, key=lambda data: data[1]))
[(7, 3, 9), (1, 7, 3), (4, 9, 6)]
As you can see this time the tuples in the list are sorted based on the second element in each tuple.
How to Sort a List of Tuples in Reverse Order
And what if you want to sort a list of tuples in reverse order?
Let’s take the following list of tuples:
>>> numbers = [(1,7,3), (4,9,6), (7,3,9)]
The Python sorted function allows you to sort a list of tuples in reverse order simply by passing an optional boolean argument called reverse. By default reverse is False but if you set it to True you will sort the list of tuples in reverse order.
Let’s confirm it by adding the reverse argument to the statement we have used in the previous section.
>>> print(sorted(numbers, key=lambda data: data[1], reverse=True))
[(4, 9, 6), (1, 7, 3), (7, 3, 9)]
Can you see how the tuples in the list are sorted in reverse order based on the second item in each tuple?
In other words, the tuples in the list are sorted in descending order.
Using the Python Sorted Function Without Lambda
In the previous example, we have passed a lambda function to the optional argument key of the sorted function.
A lambda is a function and we should also be able to pass a normal function to the optional key argument.
Let’s give it a try!
Define a function called select_item(), this function takes a tuple as input and returns the second item of the tuple.
def select_item(data):
return data[1]
Now we will call the sorted function and pass the select_item function to the key argument:
>>> print(sorted(numbers, key=select_item))
[(7, 3, 9), (1, 7, 3), (4, 9, 6)]
The result is correct.
You can compare it with the result we have obtained using a lambda function in one of the previous sections.
Sort a List of Tuples Using Itemgetter
There is another alternative to passing a lambda or a standard function to the optional key argument of the sorted function.
You can use the itemgetter function of the Python operator module.
Let’s see how the itemgetter function works when we apply it to a single tuple:
>>> itemgetter(1)((2,5,3))
5
It returns the second element of the tuple based on the index 1 passed to it.
And now let’s use the itemgetter function together with the sorted function.
>>> numbers = [(1,7,3), (4,9,6), (7,3,9)]
>>> print(sorted(numbers, key=itemgetter(1)))
[(7, 3, 9), (1, 7, 3), (4, 9, 6)]
The list of tuples is sorted based on the second element of each tuple (in the same way we have seen in previous examples using a lambda or a standard function with the optional key argument).
How Do You Sort a List of Tuples In-Place Using Python?
So far we have used the Python sorted() function that returns a new sorted list of tuples.
And what if we want to update the list of tuples in place instead of creating a new list of tuples?
We can use the list method sort().
Start from the following list:
numbers = [(2,5,3), (3,1,2), (0,0,1)]
Execute the sort method on the list numbers and then verify the value of numbers:
>>> numbers.sort()
>>> print(numbers)
[(0, 0, 1), (2, 5, 3), (3, 1, 2)]
The numbers list has been sorted in place.
Makes sense?
We have used this approach with a list of tuples but we could have used it with other lists (e.g. a list of integers or a list of lists).
The sort() method also accepts the optional key and reverse arguments in the same way the sorted function does.
Optional key argument
>>> numbers = [(2,5,3), (3,1,2), (0,0,1)]
>>> numbers.sort(key=lambda data: data[1])
>>> print(numbers)
[(0, 0, 1), (3, 1, 2), (2, 5, 3)]
Optional reverse argument
>>> numbers = [(2,5,3), (3,1,2), (0,0,1)]
>>> numbers.sort(reverse=True)
>>> print(numbers)
[(3, 1, 2), (2, 5, 3), (0, 0, 1)]
What is the Difference Between Sorted and Sort in Python?
We have already seen the difference between sorted and sort in the previous sections, let’s recap it to make sure it’s clear.
Sorted is a Python function that takes as input an iterable and returns a new sorted list. Sort is a list method that sorts a list in place (it doesn’t return a new list).
You can either use the Python sorted() function or the list method sort() depending on what you prefer in your application.
For example, if you don’t need to keep the original list you might decide to use the list sort() method.
How to Sort a List of Tuples Based on Two Elements
So far we have seen how to specify a single element to be used to compare tuples in the list in order to sort the list.
But it’s also possible to specify two or more elements to be used for comparisons when sorting the list.
We will still use the optional key argument and in this example, we will use a lambda function.
For example, let’s say we want to sort the list based on the second and third elements of each tuple.
We can use a lambda that given a tuple as input returns a tuple of two values that define the position of the elements in each tuple to be used for the sorting.
>>> numbers = [(2,4,7), (3,0,1), (3,0,0)]
>>> print(sorted(numbers, key=lambda data: (data[1], data[2])))
[(3, 0, 0), (3, 0, 1), (2, 4, 7)]
In this case, the tuples in the list are sorted first based on the second element in each tuple (data[1]) and then based on the third element in each tuple (data[2]).
Analyze the output returned by the sorted function to make sure you fully understand how this works.
Conclusion
After this tutorial, you should have a pretty good idea of how to sort a list of tuples in Python.
You have learned how to use the sorted() function and the list sort() method and what is the difference between the two.
We have also seen how optional arguments like key and reverse allow us to make Python’s sorting capability more flexible.
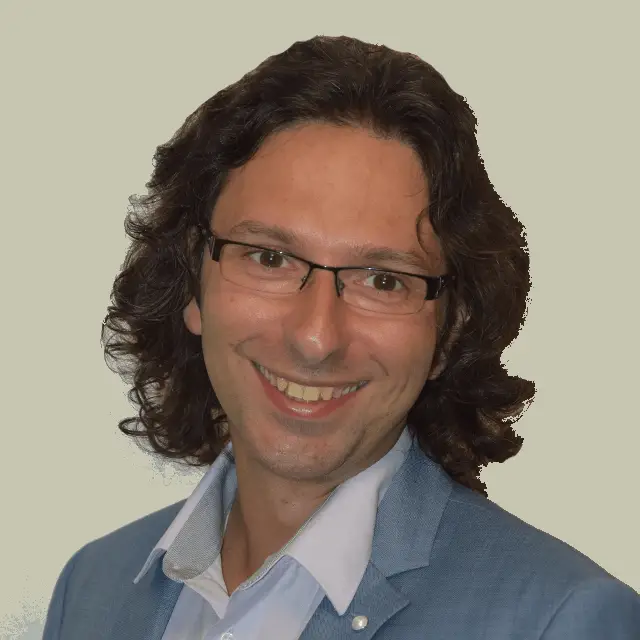
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.