Knowing how to split a Python string is a skill to learn as a Python developer. Let’s see how you can split strings in multiple ways.
The Python string data type provides the split() method that allows to split a string into a list of strings. You can split a string by using the space as separator or any other sequence of characters. A string can also be split using the split() function part of the Python re (regular expression) module.
Let’s go through a few examples that will help you understand how to split strings as part of your Python programs.
Are you ready?
What Does Split() Do in Python?
Let’s start with the basics…
Split() is a method provided by the string data type in Python. It allows to split a string into a list of strings.
For example, I want to split the following string:
>>> message = "Learn how to split a string in Python"
How can you split this string by space?
This is what the split() method returns when applied to our string:
>>> message.split()
['Learn', 'how', 'to', 'split', 'a', 'string', 'in', 'Python']
As you can see the split() method applied to a string returns a list in which every item is a string.
The separator used by default to split the string is the space.
Note: to call the split() method of the string variable message I have used the dot notation:
{variable_name}.{method_name}()
How to Split a Python String by a Specific Character?
In the previous section we have seen how by default the split() method splits a string into a list of strings by using the space character as delimiter.
But what if you want to split a string by a specific character?
For example, let’s take the following string created by replacing the space with @ characters in the previous string:
>>> message = "Learn@how@to@split@a@string@in@Python"
This is what happens if we call the split() method without passing any arguments:
>>> message.split()
['Learn@how@to@split@a@string@in@Python']
We get back a list that has a single element because there are no spaces in the string and the split() method by default uses the space as delimiter.
To split the string using the @ as delimiter instead of the space we have to pass the @ character to the split() method:
>>> message.split('@')
['Learn', 'how', 'to', 'split', 'a', 'string', 'in', 'Python']
Et voilà!
To split a string by a different character than the space you have to pass the character as argument to the split() method when applied to a given string.
How Do you Split a Python String in Half?
To split a Python string in half you can use the fact that a Python string is made of multiple characters where every character has an index.
If you use the len() function you will get back the number of characters in a string:
>>> message = "Learn@how@to@split@a@string@in@Python"
>>> len(message)
37
Do you want to see how to access a character in a string using its index?
For example, here is how we get the value of the third character in the string message:
>>> print(message[2])
a
Note: indexes in a Python string (and in sequences in general) start from zero.
To split a string in half we have to identify the middle character of the string and more specifically the index of the middle character of the string.
The string will have a middle character only if it has an odd number of characters.
Let’s take for example a string made of 5 characters. We want to get the index of the middle character by dividing the length of the string by 2:
>>> len("hello")/2
2.5
We get back a float but an index has to be integer.
So what can we do?
We can use the math.floor() function that applied to a number returns the largest integer less than or equal to the number.
>>> import math
>>> math.floor(len("hello")/2)
2
To split a string based on an index you can use the slice operator.
>>> msg = "hello"
>>> mid_index = math.floor(len(msg)/2)
>>> print(mid_index)
2
>>> print(msg[:mid_index])
he
>>> print(msg[mid_index:])
llo
If you want to remove the middle character you can do the following:
>>> print(msg[mid_index+1:])
lo
How Can You Split a Python String without the Split() Method?
It’s also possible to split a Python string without using the split method…
…it’s not necessarily something you would do but it’s an opportunity to get familiar with the Python re module.
The re module, used to work with regular expressions, provides the split function that in its basic syntax looks like this:
re.split(pattern, string)
Let’s test it first with a string that uses spaces as separators. We will pass the space character as first argument of the re.split function:
>>> message = "Learn how to split a string in Python"
>>> import re
>>> re.split(' ', message)
['Learn', 'how', 'to', 'split', 'a', 'string', 'in', 'Python']
It works!
And now let’s do the same with the string that uses the @ as separator:
>>> message = "Learn@how@to@split@a@string@in@Python"
>>> re.split('@', message)
['Learn', 'how', 'to', 'split', 'a', 'string', 'in', 'Python']
It works too…
Now you know an alternative way to split Python strings.
In the next section we will see how re.split() can be useful if you need to work with multiple delimiters instead of just one.
How Do You Split a String with Multiple Delimiters in Python?
Do you need to split a string that uses multiple delimiters?
With the re.split() function you can do it by passing all the delimiters as part of the “pattern” argument.
re.split(pattern, string)
Let’s say we want to split the following string using the space and the @ as separators:
>>> message = "Learn@how to@split a@string in@Python"
We can tell the re.split() function to use both separators by using the following syntax:
>>> re.split(' |@', message)
['Learn', 'how', 'to', 'split', 'a', 'string', 'in', 'Python']
Notice that the “pattern” argument contains the space and the @ sign separated by the | sign.
Let’s say we want to split a string using three separators, the approach is the same:
>>> message = "Learn@how to@split!a@string!in@Python"
>>> re.split(' |@|!', message)
['Learn', 'how', 'to', 'split', 'a', 'string', 'in', 'Python']
This time the three separators are the space, the @ and the exclamation mark.
How Can You Split a String by Multiple Delimiters using the String Split() Method?
Is it also possible to split a string by multiple delimiters with the string split() method?
We could do it by using the string replace method() first…
For example, let’s take the following string:
>>> message = "Learn@how to@split!a@string!in@Python"
We could split it using the space, @ and ! characters as delimiters by simply replacing any occurrences of @ and ! with spaces first.
This is what I mean…
>>> print(message.replace('@',' ').replace('!',' '))
Learn how to split a string in Python
By calling the string replace() method twice I have replaced @ and ! signs with spaces.
We can then call the split() method to split the string into a list of strings in the same way we have done before.
>>> print(message.replace('@',' ').replace('!',' ').split())
['Learn', 'how', 'to', 'split', 'a', 'string', 'in', 'Python']
Makes sense?
If it’s not clear try it in your Python shell. We are simply calling three string methods in sequence using the dot notation.
Conclusion
We have seen multiple ways to split a string in Python.
From the basic usage of the string split() method to the use of the re.split() function.
You have also learned how the split() method works when you want to split a string by spaces or by a different character.
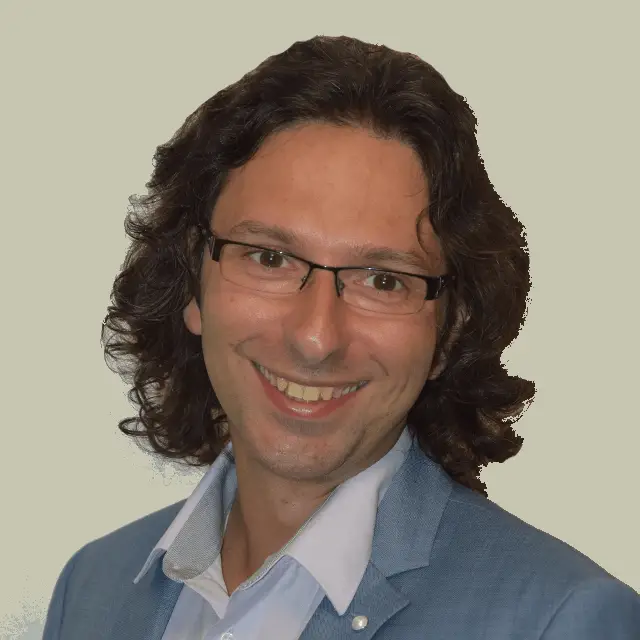
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.