The error “unexpected EOF while parsing” occurs when the interpreter reaches the end of a Python file before every code block is complete. This can happen, for example, if any of the following is not present: the body of a loop (for / while), the code inside an if else statement, or the body of a function.
We will go through a few examples that show when the “unexpected EOF while parsing” error occurs and what code you have to add to fix it.
How Do You Fix the EOF While Parsing Error in Python?
If an unexpected EOF error occurs when running a Python program, this is usually a sign that some code is missing.
This is a syntax error that shows that a specific Python statement doesn’t follow the syntax expected by the Python interpreter.
For example, when you use a for loop you have to specify one or more lines of code inside the loop.
The same applies to an if statement or to a Python function.
To fix the EOF while parsing error in Python you have to identify the construct that is not following the correct syntax and add any missing lines to make the syntax correct.
The exception raised by the Python interpreter will give you an idea about the line of code where the error has been encountered.
Once you know the line of code you can identify the code missing and add it in the right place (remember that in Python indentation is also important).
SyntaxError: Unexpected EOF While Parsing with a For Loop
Let’s see the syntax error that occurs when you write a for loop to go through the elements of a list but don’t complete the body of the loop.
In a Python file called eof_for.py define the following list:
animals = ['lion', 'tiger', 'elephant']
Then write the line below:
for animal in animals:
This is what happens when you execute this code…
$ python eof_for.py
File "eof_for.py", line 4
^
SyntaxError: unexpected EOF while parsing
A SyntaxError is raised by the Python interpreter.
The exception “SyntaxError: unexpected EOF while parsing” is raised by the Python interpreter when using a for loop if the body of the for loop is missing.
The end of the file is unexpected because the interpreter expects to find the body of the for loop before encountering the end of the Python code.
To solve the unexpected EOF while parsing error you have to add a body to the for loop. For example, a single line that prints the elements of the list:
for animal in animals:
print(animal)
Update the Python program, execute it, and confirm that the error doesn’t appear anymore.
Unexpected EOF While Parsing When Using an If Statement
Let’s start with the following Python list:
animals = ['lion', 'tiger', 'elephant']
Then write the first line of a if statement that verifies if the size of the animals list is great than 2:
if len(animals) > 2:
At this point we don’t add any other line to our code and we try to run this code.
$ python eof_if.py
File "eof_if.py", line 4
^
SyntaxError: unexpected EOF while parsing
We get back the error “unexpected EOF while parsing”.
The Python interpreter raises the unexpected EOF while parsing exception when using an if statement if the code inside the if condition is not present.
Now let’s do the following:
- Add a print statement inside the if condition.
- Specify an else condition immediately after that.
- Don’t write any code inside the else condition.
animals = ['lion', 'tiger', 'elephant']
if len(animals) > 2:
print("The animals list has more than two elements")
else:
When you run this code you get the following output.
$ python eof_if.py
File "eof_if.py", line 6
^
SyntaxError: unexpected EOF while parsing
This time the error is at line 6 that is the line immediately after the else statement.
The Python interpreter doesn’t like the fact that the Python file ends before the else block is complete.
That’s why to fix this error we add another print statement inside the else statement.
if len(animals) > 2:
print("The animals list has more than two elements")
else:
print("The animals list has less than two elements")
$ python eof_if.py
The animals list has more than two elements
The error doesn’t appear anymore and the execution of the Python program is correct.
Note: we are adding the print statements just as examples. You could add any lines you want inside the if and else statements to complete the expected structure for the if else statement.
Unexpected EOF While Parsing With Python Function
The error “unexpected EOF while parsing” occurs with Python functions when the body of the function is not provided.
To replicate this error write only the first line of a Python function called calculate_sum(). The function takes two parameters, x and y.
def calculate_sum(x,y):
At this point this is the only line of code in our program. Execute the program…
$ python eof_function.py
File "eof_function.py", line 4
^
SyntaxError: unexpected EOF while parsing
The EOF error again!
Let’s say we haven’t decided yet what the implementation of the function will be. Then we can simply specify the Python pass statement.
def calculate_sum(x,y):
pass
Execute the program, confirm that there is no output and that the Python interpreter doesn’t raise the exception anymore.
Unexpected EOF While Parsing With Python While Loop
The exception “unexpected EOF while parsing” can occur with several types of Python loops: for loops but also while loops.
On the first line of your program define an integer called index with value 10.
Then write a while condition that gets executed as long as index is bigger than zero.
index = 10
while (index > 0):
There is something missing in our code…
…we haven’t specified any logic inside the while loop.
When you execute the code the Python interpreter raises an EOF SyntaxError because the while loop is missing its body.
$ python eof_while.py
File "eof_while.py", line 4
^
SyntaxError: unexpected EOF while parsing
Add two lines to the while loop. The two lines print the value of the index and then decrease the index by 1.
index = 10
while (index > 0):
print("The value of index is " + str(index))
index = index - 1
The output is correct and the EOF error has disappeared.
$ python eof_while.py
The value of index is 10
The value of index is 9
The value of index is 8
The value of index is 7
The value of index is 6
The value of index is 5
The value of index is 4
The value of index is 3
The value of index is 2
The value of index is 1
Unexpected EOF While Parsing Due to Missing Brackets
The error “unexpected EOF while parsing” can also occur when you miss brackets in a given line of code.
For example, let’s write a print statement:
print("Codefather"
As you can see I have forgotten the closing bracket at the end of the line.
Let’s see how the Python interpreter handles that…
$ python eof_brackets.py
File "eof_brackets.py", line 2
^
SyntaxError: unexpected EOF while parsing
It raises the SyntaxError that we have already seen multiple times in this tutorial.
Add the closing bracket at the end of the print statement and confirm that the code works as expected.
Unexpected EOF When Calling a Function With Incorrect Syntax
Now we will see what happens when we define a function correctly but we miss a bracket in the function call.
def print_message(message):
print(message)
print_message(
The definition of the function is correct but the function call was supposed to be like below:
print_message()
Instead we have missed the closing bracket of the function call and here is the result.
$ python eof_brackets.py
File "eof_brackets.py", line 6
^
SyntaxError: unexpected EOF while parsing
Add the closing bracket to the function call and confirm that the EOF error disappears.
Unexpected EOF While Parsing With Try Except
A scenario in which the unexpected EOF while parsing error can occur is when you use a try statement and you forget to add the except or finally statement.
Let’s call a function inside a try block without adding an except block and see what happens…
def print_message(message):
print(message)
try:
print_message()
When you execute this code the Python interpreter finds the end of the file before the end of the exception handling block (considering that except is missing).
$ python eof_try_except.py
File "eof_try_except.py", line 7
^
SyntaxError: unexpected EOF while parsing
The Python interpreter finds the error on line 7 that is the line immediately after the last one.
That’s because it expects to find a statement that completes the try block and instead it finds the end of the file.
To fix this error you can add an except or finally block.
try:
print_message()
except:
print("An exception occurred while running the function print_message()")
When you run this code you get the exception message because we haven’t passed an argument to the function. The print_message() function requires one argument to be passed.
Modify the function call as shown below and confirm that the code runs correctly:
print_message("Hello")
Conclusion
After going through this tutorial you have all you need to understand why the “unexpected EOF while parsing” error occurs in Python.
You have also learned how to find at which line the error occurs and what you have to do to fix it.
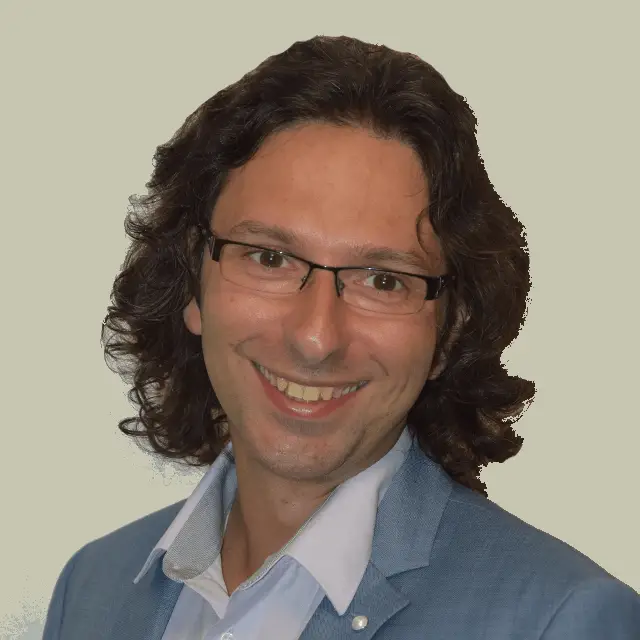
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
THANK YOU SO MUCH OMG YOU HAVE SAVED ME FROM FAILING MY COMPUTING GRADE THIS TERM THANK YOU