This article will guide you through the process of generating a random UUID (Universally Unique Identifier) string using Python.
What is a UUID?
A UUID is a 128-bit number you can use to uniquely identify information in computer systems. The term “universally unique identifier” suggests that this ID is unique, which is nearly true, given the huge number of possible UUIDs.
As a developer, it’s important to understand why you would use a UUID.
Some common applications of UUIDs are:
- Web Development: Session IDs, token generation.
- Databases: Unique keys in databases.
- Distributed Systems: Unique IDs across different machines.
Generating a Random UUID in Python
To generate a random UUID in Python you can use the uuid module that can generate several types of UUIDs. The most common type is UUID v4 which you can generate using the uuid4 function of the uuid module.
>>> import uuid
>>> uuid.uuid4()
UUID('df78ded3-d9f0-419d-a556-78eec59d838b')
>>> type(uuid.uuid4())
<class 'uuid.UUID'>
As you can see, the object you get back is of type uuid.UUID
. To convert it into a string you can use the str() built-in function.
>>> str(uuid.uuid4())
'2bc19645-bb49-45e6-bfc7-f39304e75d27'
You now have a Python string that represents a UUID.
If you want to explore UUIDs further, below you can also see four functions available in the uuid
module to generate UUIDs (including uuid4 which you have just used).
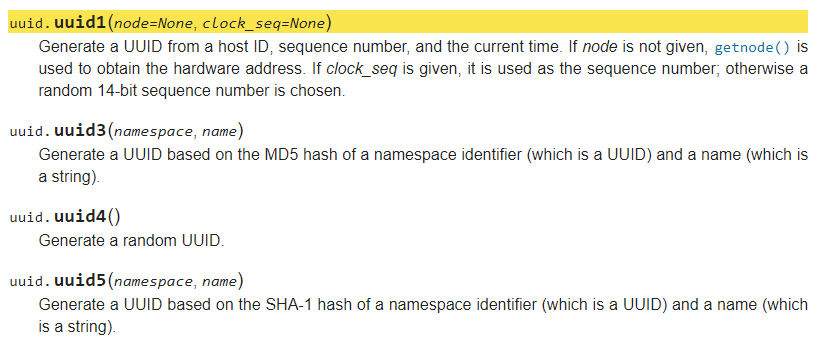
Let’s see what all the types of UUIDs look like with quick examples of code:
UUID1: Generated based on the host computer’s MAC address and the current time.
>>> uuid1 = uuid.uuid1()
>>> print("UUID1:", uuid1)
UUID1: 4495519a-8bc8-11ee-8285-1e00d11a7245
UUID3: Generated using a namespace identifier and a name, using MD5 hashing.
>>> uuid3 = uuid.uuid3(uuid.NAMESPACE_DNS, 'test.com')
>>> print("UUID3:", uuid3)
UUID3: a9da4e5d-d414-37a6-abb6-3894a0044774
UUID4: The most common, as shown before, is randomly generated.
>>> uuid4 = uuid.uuid4()
>>> print("UUID4:", uuid4)
UUID4: 75870b39-7e23-4483-bba8-be42d8d95d3b
UUID5: Similar to UUID3 but uses SHA-1 hashing.
>>> uuid5 = uuid.uuid5(uuid.NAMESPACE_DNS, 'test.com')
>>> print("UUID5:", uuid5)
UUID5: 1c39b279-6010-55d9-b677-859bffab8081
Conclusion
Generating a random UUID in Python can be very useful when you write applications that have to create objects you want to uniquely identify without significant risk of duplication.
In this article, I walked you through the basics of starting using UUIDs in your Python programs.
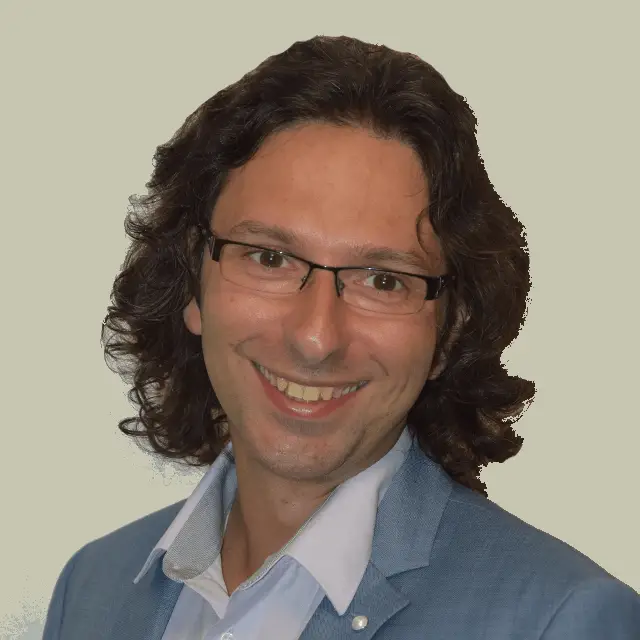
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.