Strings in Python enable you to work with text-based data, which is fundamental in every programming project, whether it’s for data analysis, web development, or automation.
In Python, think of strings as a collection of individual text characters, including letters, numbers, symbols, or spaces. In Python, every character is treated as a tiny string on its own, each one just a piece of a larger text.
A string in Python is surrounded by quotes. You can use either single quotes (‘) or double quotes (“), as long as you start and end the string with the same type of quote.
Here’s how you create a string in Python:
String using single quotes
# Create a string with single quotes
message = 'Hello, Codefather!'
print(message)
String using double quotes
# Create a string with double quotes
message = "Hello, Codefather!"
print(message)
In Python, you can also create multiline strings. Creating a multiline string in Python can be done using triple quotes (”’ or “””). Here’s an example using triple double quotes:
multiline_string = """This is a multiline string.
It spans several lines.
You can write as much as you want here."""
print(multiline_string)
And here’s an example using triple single quotes:
multiline_string = '''This is also a multiline string.
It works just like the double-quoted one.
Choose the style that you prefer.'''
print(multiline_string)
Both of these examples produce the same output, displaying the string on multiple lines. This feature is useful for creating strings that contain formatted text or for writing long comments or documentation within your code.
Action step: Before moving to the next section, execute the four examples of code above on your computer to start practicing working with Python strings.
Using Strings in a Python Program
As a beginner in Python, you can start using strings to make your programs interact with users. For instance, you could write a program that asks for the user’s name and then greets them with a personalized message.
Here’s how you would do it:
- First, you use the input() function to collect the user’s name and store it in a string variable.
- Then, you use that string to create a greeting message, which you can print out using the print() function.
# Ask for the user's name
user_name = input("What is your name? ")
# Create a greeting message
greeting = "Hello, " + user_name + "! Welcome to Python programming."
# Print the personalized greeting
print(greeting)
This use of strings helps you make interactive programs that can respond differently based on the user’s input. This is the first step to making programming feel more engaging and dynamic.
Another practical use of Python strings for beginners is creating a program that combines different pieces of information into a single, formatted message.
Let’s say you’re planning a small event and want to generate a message that includes the event’s name, and organizer. Here’s how you can do it:
# Event details
event_name = "Python Workshop"
event_organizer = "Codefather"
# Combine the details into a message
event_message = "Event: " + event_name + " organized by " + event_organizer + "."
# Print the event information
print(event_message)
The output of the code above is:
Event: Python Workshop organized by Codefather.
This code snippet demonstrates how you can concatenate (join together) different strings using the +
operator to create a single, informative message. It’s a straightforward example of how strings can be used to organize and present information clearly in your Python programs.
Challenge time: Can you modify the example of code to add the time of the event to the message using a variable called event_time?
Learning String Concatenation with Variables
A natural next step in mastering Python strings involves learning about string formatting for more complex scenarios. String formatting allows you to inject variables into your strings in a more flexible and readable way compared to simple concatenation.
Python offers several methods for string formatting, but one of the most convenient and modern approaches is using f-strings, introduced in Python 3.6.
With f-strings, you can directly embed expressions inside strings using curly braces {}
. This method not only makes your code cleaner but also more intuitive. For example, if you want to create a message that includes a user’s name and their action in a program, you can write:
user_name = "Jake"
action = "completed the Python quiz"
# Create a detailed message using an f-string
detailed_message = f"{user_name} has {action} successfully."
# Print the detailed message
print(detailed_message)
What happens when you execute this code? Test it on your code editor, you should see the following output:
Jake has completed the Python quiz successfully.
This example illustrates how f-strings can make string manipulation more straightforward and your code more readable. By incorporating variables and expressions directly into your strings, you enhance both the functionality and maintainability of your Python code.
Learn more about string concatenation in Python.
Debugging Errors in the Code with Strings
Strings can be helpful for debugging errors in your Python programs. When something goes wrong, you can use strings to print out messages that tell you what’s happening in your code. This is a way to see which parts are working and where things start to go wrong.
For example, if you’re not sure whether a part of your code is being executed, you can add a print statement with a string message like this:
print("This part of the code has run.")
If you see the message printed out, you know that the specific section of your code is working. If not, you’ve found a place to investigate further.
You can also use strings to display the values of variables at different points in your program:
speed = 5
print(f"The value of the speed is {speed}.")
# Increase the speed
speed += 10
print(f"The value of the speed is {speed}.")
This can help you track how the data in your program changes as it runs, making it easier to spot errors in the code.
Related article: Continue learning how you can use Python strings with variables in your programs.
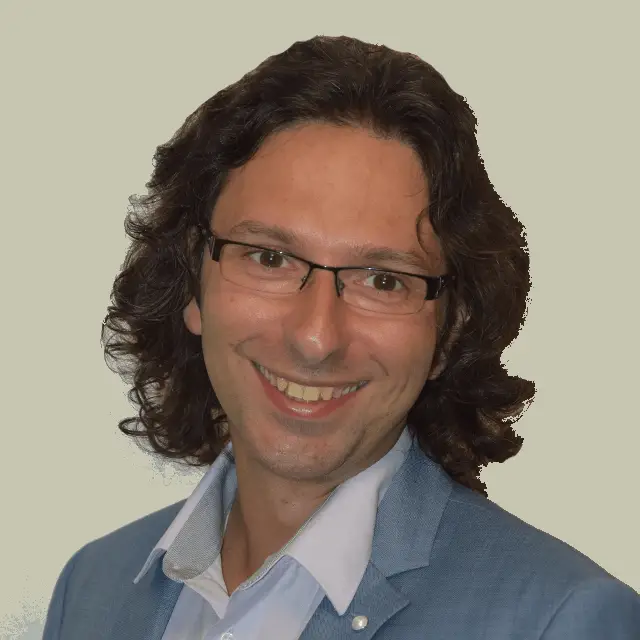
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.