I’ve heard that Python has an operator called ternary operator but I don’t know how to use it. What does the ternary operator do?
The Python ternary operator (or conditional expression) works on three operands and allows to write the logic of an if else statement in a single line of code. With the ternary operator, you specify an expression evaluated if the condition is True, the condition itself, and an expression evaluated if the condition is False.
Don’t worry if it’s not super clear from the definition, we will look at a few examples that will clarify everything.
The syntax of the ternary operator or conditional expression in Python is the following:
<expression_if_condition_is_true> if condition else <expression_if_condition_is_false>
The expression evaluated by the ternary operator depends on the boolean value of the condition.
Note: for those not familiar with booleans, a boolean variable can only have two values: True or False.
Here is an example of the ternary operator. Let’s say we want to return a different string depending on the value of a boolean variable called success.
If success is True we return the string “Operation successful” otherwise we return the string “Operation failed”.
>>> success = True
>>> "Operation successful" if success else "Operation failed"
'Operation successful'
And here is what happens if success is False:
>>> success = False
>>> "Operation successful" if success else "Operation failed"
'Operation failed'
As you can see the condition in the ternary expression is the following:
if success
This is equivalent to writing…
if success == True
Let’s confirm it…
>>> success = True
>>> "Operation successful" if success == True else "Operation failed"
'Operation successful'
>>> success = False
>>> "Operation successful" if success == True else "Operation failed"
'Operation failed'
Why Is It Called Ternary Operator?
If you are wondering why this is called ternary operator, here is the answer…
The name ternary operator comes from the fact that this operator works on three operands. The three operands are the:
- Expression evaluated if the condition is True
- Condition itself
- Expression evaluated if the condition is False.
This is equivalent to an if else statement and the advantage is that it’s written on a single line.
Assigning the Value of a Ternary Expression to a Variable
In the previous section, we have seen how to use the ternary operator.
When you write a Python program you use variables to store specific values that you want to use later on in your program.
Let’s see how to store the value returned by the previous ternary expression to a variable.
>>> success = True
>>> message = "Operation successful" if success else "Operation failed"
>>> print(message)
Operation successful
The variable message now contains the value returned by the ternary expression.
Python Shorthand Ternary Expression
Python also provides a shorter version of the ternary expression, it’s called shorthand ternary expression.
Imagine you are building a system that integrates with another system and the connection_output variable tells you if the connection between the two systems is successful.
Success scenario
>>> connection_output = "Connection OK"
>>> message = connection_output or "Connection Failed"
>>> print(message)
Connection OK
Failure scenario
>>> connection_output = None
>>> message = connection_output or "Connection Failed"
>>> print(message)
Connection Failed
As you can see in the failure scenario the shorthand ternary operator returns the string “Connection Failed” because the value of the connection_output variable is None.
Is the Ternary Operator Better than the If-Else Statement?
I want to compare how the same conditional logic can be written using the ternary operator and a standard if-else statement.
Here is the ternary operator we will work on:
message = "Operation successful" if success else "Operation failed"
And this is the way you would write it using an if-else statement:
if success:
message = "Operation successful"
else:
message = "Operation failed"
Note: make sure you give a boolean value to the variable success to execute the code above.
If you don’t give a value to the variable success you will see the following error:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'success' is not defined
I have written a tutorial that teaches you more about the Python NameError if you are interested.
As you can see the standard if-else statement requires four lines of code versus the code written using the ternary operator which only requires one line of code.
That’s pretty cool!
Should You Use the Ternary Operator? An Example With Multiple Conditions
The ternary operator allows to make your code more concise.
It helps write on a single line the same logic that would require multiple lines when using standard if-else statements.
When writing simple conditional expressions it can be a good idea to use the ternary operator to reduce the length of your code.
But…
…if the conditional expression becomes more complex using the ternary operator can make your code hard to read.
Here is an example with multiple conditions:
expression1 if condition1 else expression2 if condition2 else expression3
It’s already confusing when you read the generic syntax, let’s see an example to find out if this conditional expression gets clearer.
message = "x smaller than 10" if x < 10 else "x greater than 10" if x > 10 else "x equal to 10"
Wow…that’s a long line of code!
Let’s test it!
>>> x = 6
>>> message = "x smaller than 10" if x < 10 else "x greater than 10" if x > 10 else "x equal to 10"
>>> print(message)
x smaller than 10
>>> x = 13
>>> message = "x smaller than 10" if x < 10 else "x greater than 10" if x > 10 else "x equal to 10"
>>> print(message)
x greater than 10
>>> x = 10
>>> message = "x smaller than 10" if x < 10 else "x greater than 10" if x > 10 else "x equal to 10"
>>> print(message)
x equal to 10
The code works fine but the ternary expression is getting harder to read.
Here is how a standard if else to implement the same logic looks like:
if x < 10:
message = "x smaller than 10"
else:
if x > 10:
message = "x greater than 10"
else:
message = "x equal to 10"
Execute it in the Python shell to test this code by assigning different values to x the same way we have done it before.
This code is a lot easier to read and we can improve it using the Python elif statement:
if x < 10:
message = "x smaller than 10"
elif x > 10:
message = "x greater than 10"
else:
message = "x equal to 10"
It’s even better now, I prefer this last implementation to the initial ternary operator.
As a developer, your goal is to find the best compromise between a concise code that is also readable at the same time.
And in this case, using if-elif-else makes our code a lot cleaner.
Python Ternary Operator Using Tuples
It’s also possible to write the ternary operator in a shorter way using a Python tuple.
To see how this syntax works let’s start with the basic ternary operator syntax:
If the condition is True
>>> x = 1
>>> y = 2
>>> x if x > 0 else y
1
If the condition if False
>>> x = -1
>>> y = 2
>>> x if x > 0 else y
2
Now let’s see how to write this expression using a tuple:
If the condition is True
>>> x = 1
>>> y = 2
>>> (y, x)[x > 0]
1
If the condition if False
>>> x = -1
>>> y = 2
>>> (y, x)[x > 0]
2
The ternary operator using a tuple returns the same result as the standard ternary operator.
But why?
It can be a bit confusing when you see this tuple ternary operator for the first time.
To understand how it works you first need to know how boolean values True and False are represented as integers in Python.
Let’s use the Python shell and convert True and False to integers using the built-in class int().
>>> int(True)
1
>>> int(False)
0
As shown above True corresponds to 1 and False to 0.
We can use the output of the condition as the index to access one of the items in the tuple…
…if the condition returns 0 (False) we access the first item of the tuple otherwise the second item.
This also explains why the items of the tuple in our example are reversed:
(y, x)[x > 0]
Makes sense?
Is the Ternary Operator Faster Than an If-Else Statement?
The best way to find out if the ternary operator is faster than a standard if-else statement is to compare the performance of the two approaches.
Using the timeit module we will compare the following ternary expression…
result = x if x > 0 else y
…the following if else statement:
if x > 0:
result = x
else:
result = y
and the ternary expression below that uses a tuple:
(y, x)[x > 0]
Create a Python file called ternary_operator_performance.py with the following code:
def ternary_operator(x, y):
result = x if x > 0 else y
return result
def if_else_statement(x, y):
if x > 0:
result = x
else:
result = y
return result
def ternary_tuple(x, y):
result = (y, x)[x > 0]
return result
Then use the timeit module to measure the execution time of the three functions:
Ternary operator
$ python -m timeit -s "from ternary_operator_performance import ternary_operator" "ternary_operator(1, 2)"
10000000 loops, best of 3: 0.0503 usec per loop
Standard if else statement
$ python -m timeit -s "from ternary_operator_performance import if_else_statement" "if_else_statement(1, 2)"
10000000 loops, best of 3: 0.051 usec per loop
Ternary expression using a tuple
$ python -m timeit -s "from ternary_operator_performance import ternary_tuple" "ternary_tuple(1, 2)"
10000000 loops, best of 3: 0.0688 usec per loop
The fastest is the basic ternary operator, followed by the if-else statement and then by the ternary expression with a tuple.
Conclusion
Now you should know everything you need to use the ternary operator…
…at this point you can choose: will you use the ternary operator or a standard if-else statement?
No matter what you use make sure your code is clean.
This will make your life and the lives of those who will read your code easier 🙂
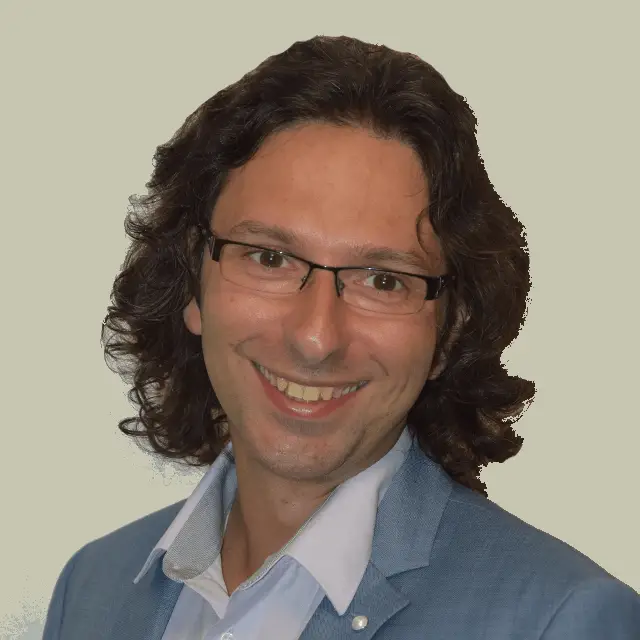
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.