You execute your Python program and you see an error, “NameError: name … is not defined”. What does it mean?
In this article I will explain you what this error is and how you can quickly fix it.
What causes a Python NameError?
The Python NameError occurs when Python cannot recognise a name in your program. A name can be either related to a built-in function or to something you define in your program (e.g. a variable or a function).
Let’s have a look at some examples of this error, to do that I will create a simple program and I will show you common ways in which this error occurs during the development of a Python program.
Ready?
A Simple Program to Print the Fibonacci Sequence
We will go through the creation of a program that prints the Fibonacci sequence and while doing that we will see 4 different ways in which the Python NameError can appear.
First of all, to understand the program we are creating let’s quickly introduce the Fibonacci sequence.
In the Fibonacci sequence every number is the sum of the two preceding numbers in the sequence. The sequence starts with 0 and 1.
Below you can see the first 10 numbers in the sequence:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, …
That’s pretty much everything we need to know to create a Python program that generates this sequence.
Let’s get started!
To simplify things our Python program will print the sequence starting from the number 1.
Here is the meaning of the variables n1, n2 and n:
Variable | Meaning |
n | nth term of the sequence |
n1 | (n-1)th term of the sequence |
n2 | (n-2)th term of the sequence |
And here is our program.
At each iteration of the while loop we:
- Calculate the nth term as the sum of the (n-2)th and (n-1)th terms.
- Assign the value of the (n-1)th terms to the (n-2)th terms.
- Assign the value of the nth terms to the (n-1)th terms.
We assign the values to the (n-2)th and (n-1)th terms so we can use them in the next iteration of the while loop to calculate the value of the nth term.
number_of_terms = int(input("How many terms do you want for the sequence? "))
n1 = 1
n2 = 0
while count < number_of_terms:
n = n1 + n2
print(n)
n2 = n1
n1 = n
count += 1
I run the program, and….
How many terms do you want for the sequence? 5
Traceback (most recent call last):
File "fibonacci.py", line 5, in <module>
while count < number_of_terms:
NameError: name 'count' is not defined
What happened?
This syntax error is telling us that the name count is not defined.
It basically means that the count variable is not defined.
So in this specific case we are using the variable count in the condition of the while loop without declaring it before. And because of that Python generates this error.
Let’s define count at the beginning of our program and try again.
number_of_terms = int(input("How many terms do you want for the sequence? "))
count = 0
n1 = 1
n2 = 0
.....
....
...
And if we run the program, we get…
How many terms do you want for the sequence? 5
1
2
3
5
8
So, all good.
Lesson 1: The Python NameError happens if you use a variable without declaring it.
Order Really Counts in a Python Program
Now I want to create a separate function that calculates the value of the nth term of the sequence.
In this way we can simply call that function inside the while loop.
In this case our function is very simple, but this is just an example to show you how we can extract part of our code into a function.
This makes our code easier to read (imagine if the calculate_nth_term function was 50 lines long):
number_of_terms = int(input("How many terms do you want for the sequence? "))
count = 0
n1 = 1
n2 = 0
while count < number_of_terms:
n = calculate_nth_term(n1, n2)
print(n)
n2 = n1
n1 = n
count += 1
def calculate_nth_term(n1, n2):
n = n1 + n2
return n
And here is the output of the program…
How many terms do you want for the sequence? 5
Traceback (most recent call last):
File "fibonacci.py", line 7, in <module>
n = calculate_nth_term(n1, n2)
NameError: name 'calculate_nth_term' is not defined
Wait…a NameError again!?!
We have defined the function we are calling so why the error?
Because we are calling the function calculate_nth_term before defining that same function.
We need to make sure the function is defined before being used.
So, let’s move the function before the line in which we call it and see what happens:
number_of_terms = int(input("How many terms do you want for the sequence? "))
count = 0
n1 = 1
n2 = 0
def calculate_nth_term(n1, n2):
n = n1 + n2
return n
while count < number_of_terms:
n = calculate_nth_term(n1, n2)
print(n)
n2 = n1
n1 = n
count += 1
How many terms do you want for the sequence? 4
1
2
3
5
Our program works well this time!
Lesson 2: Make sure a variable or function is declared before being used in your code (and not after).
Name Error With Built-in Functions
I want to improve our program and stop its execution if the user provides an input that is not a number.
With the current version of the program this is what happens if something that is not a number is passed as input:
How many terms do you want for the sequence? not_a_number
Traceback (most recent call last):
File "fibonacci.py", line 1, in <module>
number_of_terms = int(input("How many terms do you want for the sequence? "))
ValueError: invalid literal for int() with base 10: 'not_a_number'
That’s not a great way to handle errors.
A user of our program wouldn’t know what to do with this error…
We want to exit the program with a clear message for our user if something different that a number is passed as input to the program.
To stop the execution of our program we can use the exit() function that belongs to the Python sys module.
The exit function takes an optional argument, an integer that represents the exit status status of the program (the default is zero).
Let’s handle the exception thrown when we don’t pass a number to our program. We will do it with the try except statement
try:
number_of_terms = int(input("How many terms do you want for the sequence? "))
except ValueError:
print("Unable to continue. Please provide a valid number.")
sys.exit(1)
Let’s run it!
How many terms do you want for the sequence? not_a_number
Unable to continue. Please provide a valid number.
Traceback (most recent call last):
File "fibonacci.py", line 2, in <module>
number_of_terms = int(input("How many terms do you want for the sequence? "))
ValueError: invalid literal for int() with base 10: 'not_a_number'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "fibonacci.py", line 5, in <module>
sys.exit(1)
NameError: name 'sys' is not defined
Bad news, at the end you can see another NameError…it says that sys is not defined.
That’s because I have used the exit() function of the sys module without importing the sys module at the beginning of my program. Let’s do that.
Here is the final program:
import sys
try:
number_of_terms = int(input("How many terms do you want for the sequence? "))
except ValueError:
print("Unable to continue. Please provide a valid number.")
sys.exit(1)
count = 0
n1 = 1
n2 = 0
def calculate_nth_term(n1, n2):
n = n1 + n2
return n
while count < number_of_terms:
n = calculate_nth_term(n1, n2)
print(n)
n2 = n1
n1 = n
count += 1
And when I run it I can see that the exception is handled correctly:
How many terms do you want for the sequence? not_a_number
Unable to continue. Please provide a valid number.
Lesson 3: Remember to import any modules that you use in your Python program.
Catch Any Misspellings
The NameError can also happen if you misspell something in your program.
For instance, let’s say when I call the function to calculate the nth term of the Fibonacci sequence I write the following:
n = calculate_nt_term(n1, n2)
As you can see, I missed the ‘h’ in ‘nth’:
How many terms do you want for the sequence? 5
Traceback (most recent call last):
File "fibonacci.py", line 18, in <module>
n = calculate_nt_term(n1, n2)
NameError: name 'calculate_nt_term' is not defined
Python cannot find the name “calculate_nt_term” in the program because of the misspelling.
This can be harder to find if you have written a very long program.
Lesson 4: Verify that there are no misspellings in your program when you define or use a variable or a function. This also applies to Python built-in functions.
Conclusion
You now have a guide to understand why the error “NameError: name … is not defined” is raised by Python during the execution of your programs.
Let’s recap the scenarios I have explained:
- The Python NameError happens if you use a variable without declaring it.
- Make sure a variable or function is declared before being used in your code (and not after).
- Remember to import any modules that you use in your Python program.
- Verify that there are no misspellings in your program when you define or use a variable or a function. This also applies to Python built-in functions.
Does it make sense?
If you have any questions feel free to post them in the comments below 🙂
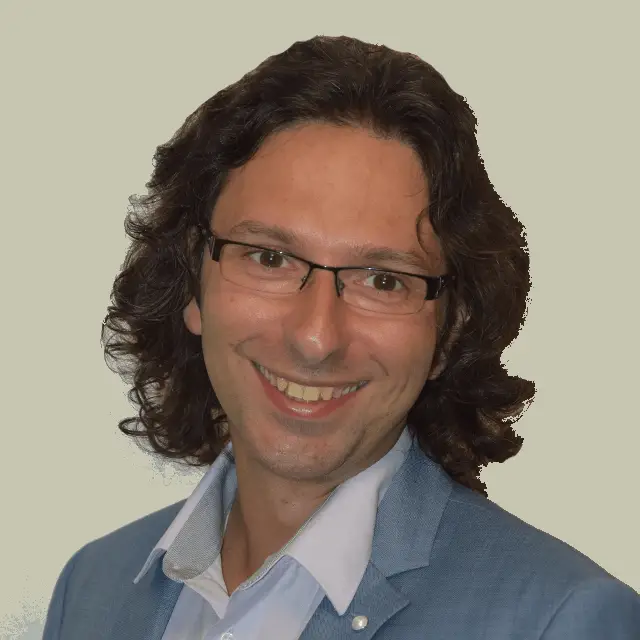
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.