The export command is a built-in command of the Bash shell that is very handy to know when you work on Linux systems or create Bash scripts.
What does the export command do in Linux?
The export command is used to make variables or functions in the current shell environment also available to child processes of the current shell (e.g. a subshell). By default, only environment variables set for export are available to a child of the current shell.
In this guide, I will show you how to use the export command in a Bash shell with practical examples to make sure you are not left with any doubts about it.
Let’s get started.
Export Command and Shell Environment
To understand what the export command does, I first want to explain the concept of environment variables in Linux.
Environment variables are variables that your Linux system needs to provide configuration settings required by the shell to execute commands.
In the table below you can see some examples of environment variables:
Environment variable | Description |
HOME | Location of the user home directory |
SHELL | Shell used by your system |
PWD | Your current directory |
HISTSIZE | Number of commands available in the Linux history |
You can use the env command to print all the environment variables for your shell.
The egrep command below prints the value of the four environment variables you have seen in the table:
[ec2-user@ip-172-1-2-3 /]$ env | egrep "HOME|SHELL|PWD|HISTSIZE"
SHELL=/bin/bash
HISTSIZE=1000
PWD=/
HOME=/home/ec2-user
Now, let’s go back to what we said before about using the export command to make variables available to child processes of our shell.
Parent and Child Processes
A child process is created by a parent process using the fork() system call.
Here’s an example, let’s start by printing the PID (Process ID) of the current shell using the echo $$ command:
[ec2-user@ip-172-1-2-3 /]$ echo $$
948
Now I start another Bash shell inside the current shell (a child process) and I use the same echo command to print the PID of the child shell (subshell):
[ec2-user@ip-172-1-2-3 /]$ bash
[ec2-user@ip-172-1-2-3 /]$ echo $$
1050
We can use the ps command with the –ppid flag to show that the process with PID 1050 is child of the process with PID 948.
The –ppid flag selects the processes of a parent process with a specific PID:
[ec2-user@ip-172-1-2-3 /]$ ps --ppid 948
PID TTY TIME CMD
1050 pts/0 00:00:00 bash
Every process can be parent and child, the only exception is the init process (the process with PID 1) that is the first process started by the kernel as part of the boot process.
The init process can only be parent of other processes.
Now that you know how parent and child processes work, let’s have a look at the role of the export command when child processes are created.
Passing a Variable From Parent to Child Process Using Export
The export command sets the export attribute of a variable or function.
Let’s see what this means using the export command without flags (or with the -p flag).
This shows all the variables set for export in the current shell (I use the head command to limit the size of the output):
[ec2-user@ip-172-1-2-3 ~]$ export -p | head -3
declare -x HISTCONTROL="ignoredups"
declare -x HISTSIZE="1000"
declare -x HOME="/home/ec2-user"
Now, let’s define a new variable called TESTVAR:
[ec2-user@ip-172-1-2-3 ~]$ TESTVAR=Hello
[ec2-user@ip-172-1-2-3 ~]$ echo $TESTVAR
Hello
As you can see we can use the echo command to print the value of our custom variable.
I’m wondering what happens to this variable if I create a child shell of the current shell (simply using the bash command):
[ec2-user@ip-172-1-2-3 ~]$ bash
[ec2-user@ip-172-1-2-3 ~]$ echo $TESTVAR
The last line of the output is empty, this means that the TESTVAR variable has no value in the new child shell.
I will go back to the parent shell (using the exit command) and using the “export -p” command I will see if the TESTVAR is set to be exported:
[ec2-user@ip-172-1-2-3 ~]$ export -p | grep TESTVAR
[ec2-user@ip-172-1-2-3 ~]$
It’s not, and this explains why the value of the variable TESTVAR was empty in the child shell.
This is exactly what you can use the export command for…
…let’s do the following:
- export the TESTVAR variable when we are in the parent shell
- use the export -p command to confirm that the variable is set for export
- create a child shell using the bash command
- confirm that the TESTVAR variable has a value in the child shell
[ec2-user@ip-172-1-2-3 ~]$ export TESTVAR
[ec2-user@ip-172-1-2-3 ~]$ export -p | grep TESTVAR
declare -x TESTVAR="Hello"
[ec2-user@ip-172-1-2-3 ~]$ bash
[ec2-user@ip-172-1-2-3 ~]$ echo $TESTVAR
Hello
Everything looks good!
The TESTVAR variable is now visible in the child shell.
Passing a Function From Parent to Child Process Using Export
In the same way we have done it with a variable, we can also pass a function to a child shell.
Let’s define a one-line Bash function, called TESTFUNCTION, that prints a message in the shell:
[ec2-user@ip-172-1-2-3 ~]$ TESTFUNCTION() { echo "This is a function"; }
[ec2-user@ip-172-1-2-3 ~]$ TESTFUNCTION
This is a function
Now export the function and confirm that is available in the subshell:
[ec2-user@ip-172-1-2-3 ~]$ export TESTFUNCTION
[ec2-user@ip-172-1-2-3 ~]$ export -p | grep TESTFUNCTION
declare -x TESTFUNCTION
[ec2-user@ip-172-1-2-3 ~]$ bash
[ec2-user@ip-172-1-2-3 ~]$ TESTFUNCTION
bash: TESTFUNCTION: command not found
Something didn’t work…
…the function TESTFUNCTION does not exit in the child shell.
Why?
That’s because to export a function with the Bash export command we need to pass the additional flag -f.
Let’s try again:
[ec2-user@ip-172-1-2-3 ~]$ export -f TESTFUNCTION
[ec2-user@ip-172-1-2-3 ~]$ export -p | grep TESTFUNCTION
declare -x TESTFUNCTION
[ec2-user@ip-172-1-2-3 ~]$ bash
[ec2-user@ip-172-1-2-3 ~]$ TESTFUNCTION
This is a function
Nice, it worked this time.
Bash Export Command For Variables In One Line
So far, in our examples we have used separate commands to define the variable or function and then to export it using the export command.
The two things can be done with a single export command in one line if we want to export a variable.
Let’s see how it looks like:
export TESTVAR=Hello
Makes sense?
Help for the Export Command
Usually to learn how to use a command can be very handy to use the man command.
Let’s do it with the export command:
man export
For some reason we don’t just get the manual for the export command.
We also get the manually for other commands (e.g. cd, echo, ulimit, umasks, and a lot more).
That’s because the export command is a built-in command of the Bash shell together with other commands.
For shell built-in commands is easier to use the help command:
help export
That shows the following output:
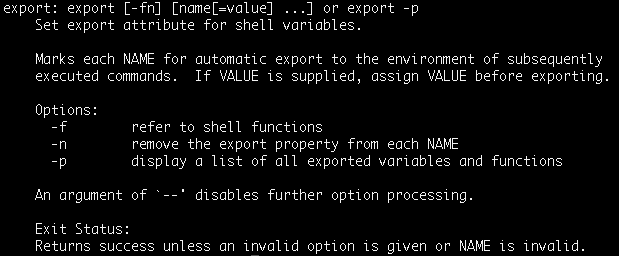
We have already covered the -f and -p flags.
Let’s see how the -n flag works. According to the help you can use this flag to remove the export property from a variable.
[ec2-user@ip-172-1-2-3 ~]$ echo $TESTVAR
Hello
[ec2-user@ip-172-1-2-3 ~]$ export TESTVAR
[ec2-user@ip-172-1-2-3 ~]$ export -p | grep TESTVAR
declare -x TESTVAR="Hello"
[ec2-user@ip-172-1-2-3 ~]$ export -n TESTVAR
[ec2-user@ip-172-1-2-3 ~]$ export -p | grep TESTVAR
This confirms that after using the export -p command the variable TESTVAR doesn’t appear anymore in the list of variables marked for export.
The Export Command and the Path Environment Variable
The PATH environment variable is one of the most important variables in a Linux system. It gives the shell a list of directories where to search for executable files when a command is executed by a user. It allows to execute commands without knowing the full path of their binaries.
When I open my Linux shell the PATH environment variable is already defined:
[ec2-user@ip-172-1-2-3 ~]$ env | grep PATH
PATH=/usr/local/bin:/usr/bin:/usr/local/sbin:/usr/sbin:/home/ec2-user/.local/bin:/home/ec2-user/bin
How come?
That’s because is automatically defined and exported in the .bash_profile file, located in the home directory on my user.
This file is used by default to initialise the user environment.
[ec2-user@ip-172-1-2-3 ~]$ pwd
/home/ec2-user
[ec2-user@ip-172-1-2-3 ~]$ grep PATH .bash_profile
PATH=$PATH:$HOME/.local/bin:$HOME/bin
export PATH
You can update the .bash_profile file if you want to add more directories to the PATH variable.
Note that directories in the PATH variable must be separated by a colon ( : ).
Conclusion
The export command is one of the Linux commands people have often doubts about.
It’s common seeing people use the export command without a full understanding of what’s behind it and how it relates to child processes.
I hope you have found this guide useful and that it has answered all the questions you have about the Bash export command.
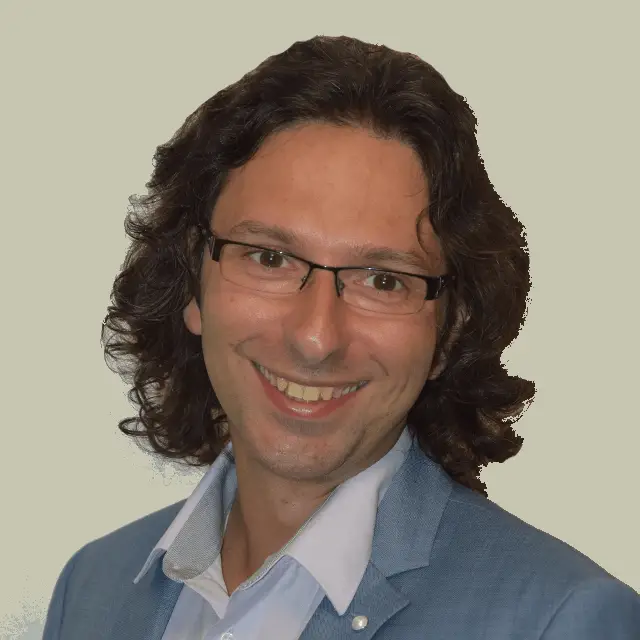
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.