Are you seeing the syntax error “unexpected end of file” while executing a Bash script? Let’s find out the cause of this error and how to fix it.
When executing a Bash script you might see the error “syntax error: unexpected end of file” if you have created your script using Windows. That’s because Windows uses a combination of two characters, Carriage Return and Line Feed, as a line break in text files (also known as CRLF) while Unix and Linux only use the Line Feed character as a line break.
I will show you an example of this error and how to fix it!
An Example of the “unexpected end of file” Error on Linux
Here is what happens if you save a Bash script using Windows and then you execute it on Linux.
Using Windows Notepad create a Bash script called end_of_file.sh
that contains the following lines of code:
#/bin/bash
if [ $# -gt 0 ]; then
echo "More than one argument passed"
else
echo "No arguments passed"
fi
Here is the output you will get when executing the script on Unix or Linux:
[ec2-user@localhost scripts]$ ./end_of_file.sh
./end_of_file.sh: line 2: $'\r': command not found
./end_of_file.sh: line 8: syntax error: unexpected end of file
How can you confirm what is causing this error?
Edit the script with the vim editor using the -b flag that runs the editor in binary mode:
[ec2-user@localhost scripts]$ vim -b end_of_file.sh
(Below you can see the content of the script)
#/bin/bash^M
^M
if [ $# -gt 0 ]; then^M
echo "More than one argument passed"^M
else^M
echo "No arguments passed"^M
fi^M
At the end of each line, you will see the ^M character. What is that character?
The ^M character is the Carriage Return character added by Windows at the end of every line. This is used by Windows but not by Unix or Linux for line breaks.
This Carriage Return character is causing the error you are seeing.
Fixing the “unexpected end of file” Error
To fix the syntax error “unexpected end of file” you have to convert the script into a format that Linux understands. The most common tool to do that is the dos2unix
command.
If dos2unix is not present on your system you can use the package manager of your Unix (Linux) distribution to install it. For instance, on the server I’m using for this tutorial, I can use YUM (Yellowdog Updater Modified) to install the package.
To search for the package to install I use the yum search
command:
[root@localhost ~]$ yum search dos2unix
Loaded plugins: extras_suggestions, langpacks, priorities, update-motd
====================== N/S matched: dos2unix =====================================
dos2unix.x86_64 : Text file format converters
Then I run the yum install
command to install the package:
[root@localhost ~]$ yum install dos2unix
Loaded plugins: extras_suggestions, langpacks, priorities, update-motd
amzn2-core | 2.4 kB 00:00:00
amzn2extra-docker | 1.8 kB 00:00:00
Resolving Dependencies
--> Running transaction check
---> Package dos2unix.x86_64 0:6.0.3-7.amzn2.0.2 will be installed
--> Finished Dependency Resolution
Dependencies Resolved
==================================================================================
Package Arch Version Repository Size
==================================================================================
Installing:
dos2unix x86_64 6.0.3-7.amzn2.0.2 amzn2-core 75 k
Transaction Summary
==================================================================================
Install 1 Package
Total download size: 75 k
Installed size: 194 k
Is this ok [y/d/N]: y
Downloading packages:
dos2unix-6.0.3-7.amzn2.0.2.x86_64.rpm | 75 kB 00:00:00
Running transaction check
Running transaction test
Transaction test succeeded
Running transaction
Installing : dos2unix-6.0.3-7.amzn2.0.2.x86_64 1/1
Verifying : dos2unix-6.0.3-7.amzn2.0.2.x86_64 1/1
Installed:
dos2unix.x86_64 0:6.0.3-7.amzn2.0.2
Complete!
We are ready to convert our script using dos2unix!
[ec2-user@localhost scripts]$ dos2unix end_of_file.sh
dos2unix: converting file end_of_file.sh to Unix format ...
The output of the dos2unix command confirms that it’s converting the .sh file to Unix format.
Now let’s try to execute the script again:
[ec2-user@localhost scripts]$ ./end_of_file.sh No arguments passed
It works!
The script is executing correctly the echo command in the else branch of the if/else statement (see the Bash script we created at the beginning of this tutorial).
Conclusion
The error we have fixed in this tutorial is quite a common one with Linux Shell scripting. I have found myself having to fix it with the dos2unix command several times over the past few years.
Now you know what to do if you see the syntax error “unexpected end of file” while running a Bash script 🙂
Related article: The test script we have created prints the output message after verifying the number of arguments we have passed to it (zero in this case). To learn how this check works in Bash, go through the CodeFatherTech tutorial about Bash script arguments.
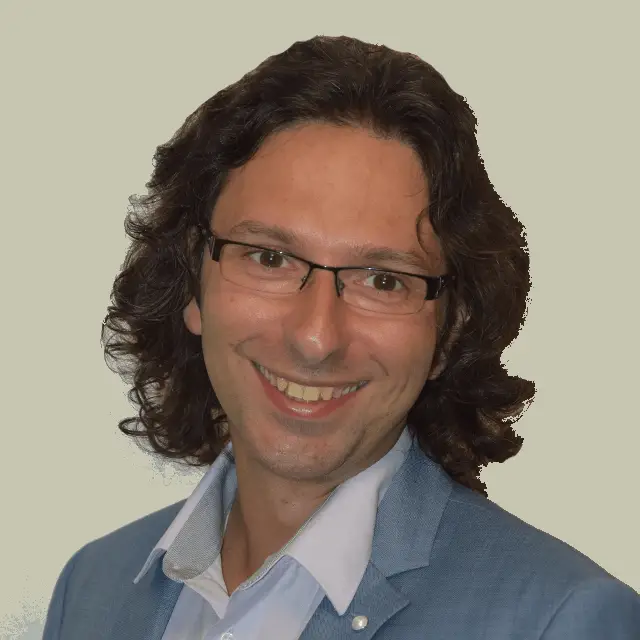
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
Excellent!!! Thanks a lot for the info.
Excellent!!! Thanks a lot for the info.