In this tutorial, you will see how to convert a tuple to a string in Python. We will go through a few examples you will find useful for your applications.
Given a tuple of strings, the simplest method to convert that tuple to a single string in Python is with the string join() method. This method generates a string by concatenating the strings in the tuple. This works fine if the elements of the tuple are strings, if that’s not the case make sure to convert them into strings first.
We will start with a simple example and then go through different scenarios you might also encounter in your Python programs.
Converting a Python Tuple to String and Back
Let’s say you have the following tuple of strings:
>>> codefather = ('c','o','d','e','f','a','t','h','e','r')
To convert a tuple of strings into a single string you can use the string join method that concatenates the strings in an iterable to create a single string.
>>> ''.join(codefather)
'codefather'
As you can see, we have applied the join()
method to an empty string that acts as a separator between each character in the final string.
In this case, the iterable is a tuple. It could also be a list or a set.
To convert this string back to a tuple you have to split the string into a tuple of characters. To do that you can use the tuple() sequence type.
>>> codefather = 'codefather'
>>> tuple(codefather)
('c', 'o', 'd', 'e', 'f', 'a', 't', 'h', 'e', 'r')
Give it a try by using the Python shell on your computer.
Convert a Python Tuple to a String with a Comma Separator
In some scenarios, you might want to use the comma as a separator when converting a tuple into a string.
For example, let’s take a tuple that contains names of planets:
>>> planets = ('earth','mars','saturn','jupiter')
If you use the join()
method in the same way we have done in the previous section, you will get the following string:
>>> ''.join(planets)
'earthmarssaturnjupiter'
To make the final string readable add a comma between each string in the tuple. We will use the comma as a separator of the join() method (in the previous example the separator was an empty string).
>>> ','.join(planets)
'earth,mars,saturn,jupiter'
For a cleaner code, you can also store the separator in a variable, this can be handy in case you want to change the separator later on.
>>> separator = ','
>>> separator.join(planets)
'earth,mars,saturn,jupiter'
Note: this method applies to any separator character you want to use.
Convert a Tuple that Contains Numbers to a String
Let’s see what happens if we apply the join() method to a tuple that contains a mix of strings and integers:
>>> values = ('radius', 34)
>>> ''.join(values)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: sequence item 1: expected str instance, int found
The error “sequence item 1: expected str instance, int found” tells us that the element of the tuple identified by index 1 should be a string but it’s an int instead.
The official Python documentation for the join() method confirms that a TypeError
is raised if the iterable contains any non-string values (like integers):

To make this code work we have to convert any numbers in the tuple into strings first, and to do that you can use a list comprehension.
>>> values = ('radius', 34)
>>> separator = ','
>>> separator.join([str(value) for value in values])
'radius,34'
The list comprehension converts the tuple into a list where every item is a string. Then the list is passed to the join method.
To clarify how this works, if you are not familiar with list comprehensions, you can see the output below that shows what the list comprehension returns:
>>> [str(value) for value in values]
['radius', '34']
The same error occurs if the tuple contains floats:
>>> values = (3.14, 5.67, 7.89)
>>> ''.join(values)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: sequence item 0: expected str instance, float found
You get an exception when trying to use the join() method directly. As an alternative, you can use the same list comprehension we have used previously.
>>> separator = ','
>>> separator.join([str(value) for value in values])
'3.14,5.67,7.89'
Using Map() Function to Convert a Tuple of Numbers into a String
Let’s see how you can use the map() function to convert a tuple of numbers into a string instead of using a list comprehension (as seen in the previous section).
Here is the syntax of the map() function:

If you pass the str function as the first argument and the tuple as the second argument you will get the following output:
>>> values = (3.14, 5.67, 7.89)
>>> map(str, values)
<map object at 0x101125970>
You can pass this map object to the tuple() sequence type to get back a tuple:
>>> tuple(map(str, values))
('3.14', '5.67', '7.89')
Notice how this time every element of the tuple is a string and not a float.
Now, let’s use map() together with join() to create a string from our tuple of numbers.
>>> ' '.join(map(str, values))
'3.14 5.67 7.89'
Et voilà, here is the final string! 🙂
Conclusion
We went through a few ways to convert a Python tuple to a string.
Now you know how you can use the string join() method, the map() function, and list comprehensions to create the best implementation you want.
Which approach do you prefer amongst the ones we have seen?
Related article: Learn more about tuples with the step-by-step CodeFatherTech tutorial about tuples in Python.
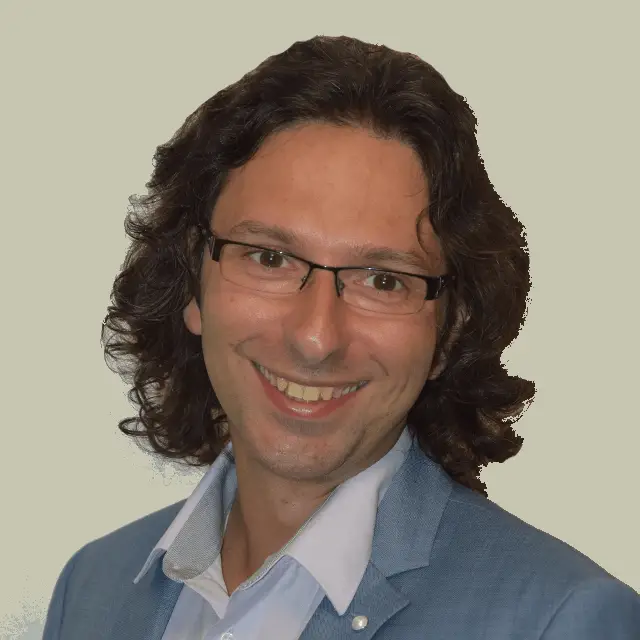
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.