You might have seen a Python recursion error when running your Python code. Why does this happen? Is there a way to fix this error?
A Python RecursionError exception is raised when the execution of your program exceeds the recursion limit of the Python interpreter. Two ways to address this exception are increasing the Python recursion limit or refactoring your code using iteration instead of recursion.
Let’s go through some examples so you can understand how this works.
The recursion begins!
RecursionError: Maximum Recursion Depth Exceeded in Comparison
Let’s create a program to calculate the factorial of a number following the formula below:
n! = n * (n-1) * (n-2) * ... * 1
Write a function called factorial and then use print statements to print the value of the factorial for a few numbers.
def factorial(n):
if n == 0:
return 1
else:
return n*factorial(n-1)
This is a recursive function…
A recursive function is a function that calls itself. Recursion is not specific to Python, it’s a concept common to most programming languages.
You can see that in the else statement of the if else we call the factorial function passing n-1 as parameter.
The execution of the function continues until n is equal to 0.
Let’s see what happens when we calculate the factorial for two small numbers:
if __name__ == '__main__':
print("The factorial of 4 is: {}".format(factorial(4)))
print("The factorial of 5 is: {}".format(factorial(5)))
[output]
The factorial of 4 is: 24
The factorial of 5 is: 120
After checking that __name__ is equal to ‘__main__’ we print the factorial for two numbers.
It’s all good.
But, here is what happens if we calculate the factorial of 1000…
print("The factorial of 1000 is: {}".format(factorial(1000)))
[output]
Traceback (most recent call last):
File "recursion_error.py", line 9, in <module>
print("The factorial of 1000 is: {}".format(factorial(1000)))
File "recursion_error.py", line 5, in factorial
return n*factorial(n-1)
File "recursion_error.py", line 5, in factorial
return n*factorial(n-1)
File "recursion_error.py", line 5, in factorial
return n*factorial(n-1)
[Previous line repeated 995 more times]
File "recursion_error.py", line 2, in factorial
if n <= 1:
RecursionError: maximum recursion depth exceeded in comparison
The RecursionError occurs because the Python interpreter has exceeded the recursion limit allowed.
The reason why the Python interpreter limits the number of times recursion can be performed is to avoid infinite recursion and hence avoid a stack overflow.
Let’s have a look at how to find out what the recursion limit is in Python and how to update it.
What is the Recursion Limit in Python?
Open the Python shell and use the following code to see the value of the recursion limit for the Python interpreter:
>>> import sys
>>> print(sys.getrecursionlimit())
1000
Interesting…the limit is 1000.
To increase the recursion limit to 1500 we can add the following lines at the beginning of our program:
import sys
sys.setrecursionlimit(1500)
If you do that and try to calculate again the factorial of 1000 you get a long number back (no more errors).
The factorial of 1000 is: 4023872600770937735437024339230039857193748642107146325437999104299385123986290205920
.......835777939410970027753472000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000
That’s good! But…
…this solution could work if like in this case we are very near to the recursion limit and we are pretty confident that our program won’t end up using too much memory on our system.
How to Catch a Python Recursion Error
One possible option to handle the RecursionError exception is by using try except.
It allows to provide a clean message when your application is executed instead of showing an unclear and verbose exception.
Modify the “main” of your program as follows:
if __name__ == '__main__':
try:
print("The factorial of 1000 is: {}".format(factorial(1000)))
except RecursionError as re:
print("Unable to calculate factorial. Number is too big.")
Note: before executing the program remember to comment the line we have added in the section before that increases the recursion limit for the Python interpreter.
Now, execute the code…
You will get the following when calculating the factorial for 1000.
$ python recursion_error.py
Unable to calculate factorial. Number is too big.
Definitely a lot cleaner than the long exception traceback.
Interestingly, if we run our program with Python 2.7 the output is different:
$ python2 recursion_error.py
Traceback (most recent call last):
File "recursion_error.py", line 13, in <module>
except RecursionError as re:
NameError: name 'RecursionError' is not defined
We get back a NameError exception because the exception of type RecursionError is not defined.
Looking at the Python documentation I can see that the error is caused by the fact that the RecursionError exception was only introduced in Python 3.5:

So, if you are using a version of Python older than 3.5 replace the RecursionError with a RuntimeError.
if __name__ == '__main__':
try:
print("The factorial of 1000 is: {}".format(factorial(1000)))
except RuntimeError as re:
print("Unable to calculate factorial. Number is too big.")
In this way our Python application works fine with Python2:
$ python2 recursion_error.py
Unable to calculate factorial. Number is too big.
How Do You Stop Infinite Recursion in Python?
As we have seen so far, the use of recursion in Python can lead to a recursion error.
How can you prevent infinite recursion from happening? Is that even something we have to worry about in Python?
Firstly, do you think the code we have written to calculate the factorial could cause an infinite recursion?
Let’s look at the function again…
def factorial(n):
if n == 0:
return 1
else:
return n*factorial(n-1)
This function cannot cause infinite recursion because the if branch doesn’t make a recursive call. This means that the execution of our function eventually stops.
We will create a very simple recursive function that doesn’t have an branch breaking the recursion…
def recursive_func():
recursive_func()
recursive_func()
When you run this program you get back “RecursionError: maximum recursion depth exceeded”.
$ python recursion_error2.py
Traceback (most recent call last):
File "recursion_error2.py", line 4, in <module>
recursive_func()
File "recursion_error2.py", line 2, in recursive_func
recursive_func()
File "recursion_error2.py", line 2, in recursive_func
recursive_func()
File "recursion_error2.py", line 2, in recursive_func
recursive_func()
[Previous line repeated 996 more times]
RecursionError: maximum recursion depth exceeded
So, in theory this program could have caused infinite recursion, in practice this didn’t happen because the recursion depth limit set by the Python interpreter prevents infinite recursion from occurring.
How to Convert a Python Recursion to an Iterative Approach
Using recursion is not the only option possible. An alternative to solve the RecursionError is to use a Python while loop.
We are basically going from recursion to iteration.
def factorial(n):
factorial = 1
while n > 0:
factorial = factorial*n
n = n - 1
return factorial
Firstly we set the value of the factorial to 1 and then at each iteration of the while loop we:
- Multiply the latest value of the factorial by n
- Decrease n by 1
The execution of the while loop continues as long as n is greater than 0.
I want to make sure that this implementation of the factorial returns the same results as the implementation that uses recursion.
So, let’s define a Python list that contains a few numbers. Then we will calculate the factorial of each number using both functions and compare the results.
We use a Python for loop to go through each number in the list.
Our program ends as soon as the factorials calculated by the two functions for a given number don’t match.
def factorial(n):
factorial = 1
while n > 0:
factorial = factorial*n
n = n - 1
return factorial
def recursive_factorial(n):
if n == 0:
return 1
else:
return n*factorial(n-1)
numbers = [4, 9, 18, 23, 34, 56, 78, 88, 91, 1000]
for number in numbers:
if factorial(number) != recursive_factorial(number):
print("ERROR: The factorials calculated by the two functions for the number {} do not match.".format(number))
print("SUCCESS: The factorials calculated by the two functions match")
Let’s run our program and see what we get:
$ python factorial.py
SUCCESS: The factorials calculated by the two functions match
Great!
Our implementation of the factorial using an iterative approach works well.
Conclusion
In this tutorial we have seen why the RecursionError occurs in Python and how you can fix it.
Two options you have are:
- Increase the value of the recursion limit for the Python interpreter.
- Use iteration instead of recursion.
Which one are you going to use?
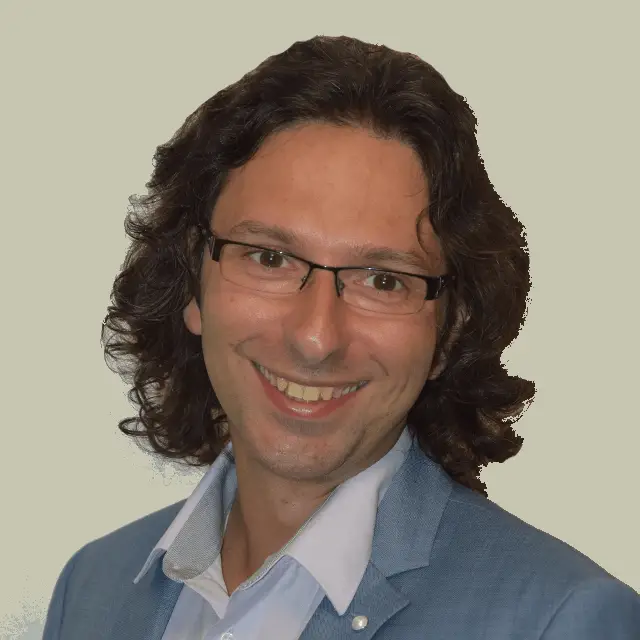
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.