While reading a Python program you might find the expression if __name__ == ‘__main__’.
Let’s have a look at what it means and why you should know about it.
The condition if __name__ == ‘__main__’ is used in a Python program to execute the code inside the if statement only when the program is executed directly by the Python interpreter. When the code in the file is imported as a module the code inside the if statement is not executed.
It might not be very clear why this is done and with this tutorial, the reason will become clear.
Here we go!
What is __main__ in Python?
The word __name__ in Python represents a special variable.
There are many special variables in Python that start and end with double underscores. To make it short they are referred to as dunder (from Double Underscores). So __name__ is pronounced “dunder name”.
Let’s see what the value of __main__ is by using the Python shell:
>>> __name__
'__main__'
As you can see the value is ‘__main__’.
Now, let’s try to import a Python module to see the value assigned to the __name__ variable associated to the module:
>>> import random
>>> random.__name__
'random'
Ok, so after importing the random module we can see that the value of __name__ for the module is ‘random’…basically the name of the module.
We are getting somewhere but maybe if we create our own Python program this can become clearer.
The Values of the Python __name__ Variable
Create a file called understand_name_main.py, this file contains the following code:
print("The value of __name__ is {}".format(__name__))
A single print statement that uses the string format method to print the value of __name__.
Let’s see what happens when:
- We execute this code directly.
- The file is imported as a module (a file that contains Python code can be imported by using the import statement and by taking away the .py at the end of the filename).
When we execute the code directly by referencing the Python file the value of __name__ is __main__:
$ python understand_name_main.py
The value of __name__ is __main__
Instead, if we import the module from the Python shell the value of __name__ is…
>>> import understand_name_main
The value of __name__ is understand_name_main
…understand_name_main.
So the name of the module. In the same way, we have seen before with the Python random module.
So, the value of __name__ changes depending on the way our Python code is executed.
But, why? And how can this help us as developers?
An Example to Understand __name__ in Python
We have seen how the value of the __name__variable changes depending on how we execute our code.
But, how do we use the condition if __name__ == “__main__” in our Python programs?
To explain this concept we will create a Python program that calls three different functions. We will use the same file understand_name_main.py:
def step1():
print("Executing step1...")
def step2():
print("Executing step2...")
def step3():
print("Executing step3...")
step1()
step2()
step3()
This code runs three functions. The implementation of the three functions is a simple print statement because I want you to focus on the concept behind this and not on the implementation of the functions:
$ python understand_name_main.py
Executing step1...
Executing step2...
Executing step3...
Now, let’s say another Python program needs to use the function step1(). To do that we would have to import our file as a module.
Here is what happens when we do that:
>>> import understand_name_main
Executing step1...
Executing step2...
Executing step3...
>>> understand_name_main.step1()
Executing step1...
After importing the module we can execute the step1() function. The problem is that when we import the module the three lines below are automatically executed:
step1()
step2()
step3()
How can we avoid that?
Checking If __name__ is Equal to __main__ When Importing a Module
To prevent the behavior we have seen in the previous section from happening we can check if the value of __name__ is equal to “__main__”.
In this way the code inside the if condition is only executed if the Python file is called directly and not when it is imported as a module.
Our program becomes:
def step1():
print("Executing step1...")
def step2():
print("Executing step2...")
def step3():
print("Executing step3...")
if __name__ == "__main__":
step1()
step2()
step3()
Let’s confirm that the three functions are still executed when we call the Python program directly:
$ python understand_name_main.py
Executing step1...
Executing step2...
Executing step3...
And also that the three functions are not executed when we import this as a module:
>>> import understand_name_main
>>> understand_name_main.step1()
Executing step1...
That’s a lot better this time!
Also, let’s assume the understand_name_main module includes hundreds of functions and you don’t want to import all of them.
How can you just import the step1() function?
You can use the following syntax:
>>> from understand_name_main import step1
>>> step1()
Executing step1...
>>> step2()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'step2' is not defined
As you can see in this case we have only imported the function step1 that gets executed successfully.
When we try to execute step2() it fails with NameError: name ‘step2’ is not defined.
Is There a Main Function in Python?
The concept of main is quite standard in languages like Java or C and it represents the entry point for the execution of a program.
A common approach used in Python is to create a function called main() executed inside the if statement that checks the value of the __name__ variable.
The main() function is the place where multiple functions are called to obtain a specific result.
In our case the three functions would be called from the main function:
def step1():
print("Executing step1...")
def step2():
print("Executing step2...")
def step3():
print("Executing step3...")
def main():
step1()
step2()
step3()
if __name__ == "__main__":
main()
Naming the function main() is just a common naming convention that increases the readability of the program considering that the concept of main is well-known to other developers too.
In reality, nothing prevents us from giving another arbitrary name to the main function.
Before continuing with this tutorial confirm that our updated code works fine in both scenarios:
- Code executed directly
- Module imported
Python __name__, __main__ and Arguments
Inside the if condition that checks if the variable __name__ is equal to “__main__” we can also handle any parameters passed to our Python application when called directly.
To handle arguments passed to the application we can use the sys module.
import sys
def main(args):
print(args)
if __name__ == "__main__":
main(sys.argv)
In this code we do the following:
- Import the sys module.
- Define a main() function that takes one argument as input. This argument will be a list of strings that contains the arguments passed to the application when executed.
- Pass sys.argv to the main() function inside the if condition that verifies the value of the __name__ variable.
Here is what happens when we run the program in the terminal:
$ python test_args.py
['test_args.py']
$ python test_args.py arg1 arg2
['test_args.py', 'arg1', 'arg2']
As you can see the first argument received by the Python program is the name of the file .py itself.
Let’s update the main function to print the type of the args variable.
def main(args):
print(type(args))
print(args)
It confirms that args is a list:
$ python test_args.py arg1 arg2
<class 'list'>
['test_args.py', 'arg1', 'arg2']
When passing arguments we can also unpack the arguments we need in our program excluding the name of the program itself:
import sys
def main(a, b):
return int(a) * int(b)
if __name__ == "__main__":
arg1, arg2 = sys.argv[1:3]
print(main(arg1, arg2))
And here is how we run this program and the result we get back:
$ python product.py 4 5
20
This code uses the slicing operator to take the two numbers passed via the command line. Then it passes the two numbers (at this point still in string format) to the main() function.
The main function then returns the product of the two numbers converted to integers.
Conclusion
Now you know how to use the __name__ variable in your Python programs.
It allows you to include code that doesn’t get executed if your module is imported by another program.
Imagine if someone else wants to use the functions you have created but doesn’t want to automatically execute a bunch of logic you have implemented when your application is executed directly.
And now I have a small question for you…
Do you remember the short name you can use for the variable __name__ instead of calling it “double underscore name double underscore“? 🙂
Bonus read: now that you know how to use ‘if __name__ == “__main__”’ in your Python programs, I want to show you how you can use this concept as part of the implementation of the binary search algorithm.
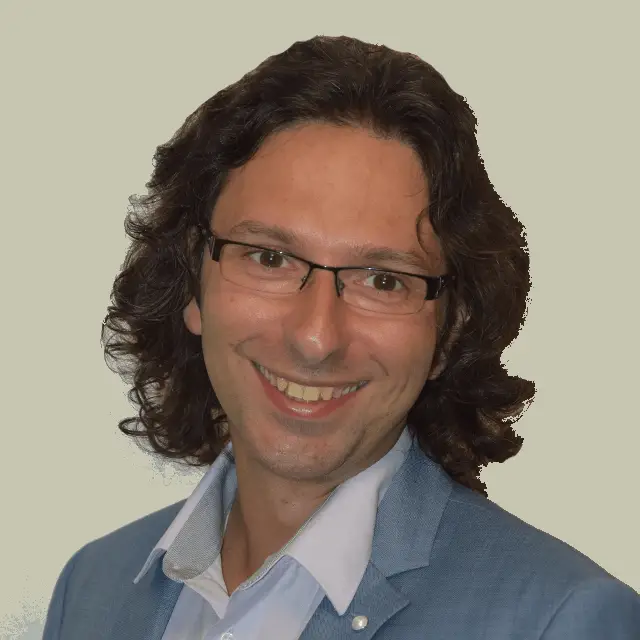
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
You are a great teacher
Finally, an explanation of if __name__ == “__main” that actually makes sense!!! Thank you codefather 🙂
Oh man! I have no words to say thanks for this wonderful explanation. AWESOME.
Ah, I see! I’ve always wondered why that line of code appears in so many Python scripts. It’s nice to finally understand its purpose and how it works. Thank you for explaining it in a clear and concise manner!