Knowing how to reverse a Python string is a common thing you might have to do as a Python developer. It could also help you in coding interviews.
Python does not provide a built-in function to reverse the characters in a string. To reverse a string in Python you can use the slice operator with the syntax [::-1]. You can also use the reversed() function together with the string join() function.
Let’s go through different ways to return a Python string in reverse order.
How Do You Reverse a String in Python?
Let’s immediately learn a simple method to reverse a string.
I want to give you the solution to the problem first, then we will understand how this solution exactly works.
Open the Python shell and define a Python string:
>>> word = "Python"
To reverse a string in Python you can use slicing with the following syntax: [::-1].
>>> print(word)
Python
>>> print(word[::-1])
nohtyP
Fantastic! Nice and concise!
As you can see in the second print statement the reversed string is returned.
If you want to make this code reusable you can create a function that given a string returns the reversed string.
>>> def reverse_word(word):
... return word[::-1]
...
>>> print(reverse_word("Python"))
nohtyP
In the next section, we will learn how the expression [::-1] works.
What is the Meaning of [::-1] in Python?
You might be wondering, what does the syntax [::-1] mean?
This is the Python slice operator that in its basic form allows to select a substring from a string.
For example, let’s say you want to select the first three characters of our word, you can use the following slicing expression.
>>> print(word[0:3])
Pyt
The substring starts at the character with index 0 (the first character) and ends at the character with index 3 – 1 so 2.
If we omit the first zero in the slice expression the output is the same:
>>> print(word[:3])
Pyt
But, the slicing syntax we have used before is slightly different:
[::-1]
This expression uses the extended syntax for the slice operator.
[begin:end:step]
By default, the value of step is 1. Also if you don’t specify any value for begin the Python interpreter will start from the beginning of the string.
And, if you don’t specify a value for end Python will go until the end of the string.
Let’s see what happens if we don’t specify a value for begin and end and we set the step to 1:
>>> print(word)
Python
>>> print(word[::1])
Python
Python goes through every character of the string from the beginning to the end using a step equal to 1.
The only difference in the expression we have seen before to reverse a string is that we have used a step equal to -1.
When you specify a step equal to -1 in a slicing expression the Python interpreter goes through the characters of the string backward. This explains the output returned by the expression [::-1].
>>> print(word[::-1])
nohtyP
How Do You Reverse a String with a Python While Loop?
We can obtain the same output returned by the slice operator but using a while loop.
This is not something you would necessarily use if you had to reverse a string in a real application considering that it requires more lines of code compared to slicing.
At the same time knowing how to reverse a string in Python with a while loop helps you develop the way you think when you look for a solution to a coding problem.
Here is what we want to do…
Start from the end of our input string and go backward one character at a time using a while loop.
Every character is added to the new string reversed_word which is initially an empty string and at the end contains the original string but reversed.
def reversed_word(word):
reversed_word = ''
index = len(word) - 1
while index >= 0:
reversed_word += word[index]
index -= 1
return reversed_word
As you can see we start at the index len(word) -1, the last character of our string. Then we go back, at every iteration of the while loop, as long as the index is greater than or equal to zero.
Remember that len(word) is the length of the string.
Note: writing index -= 1 is the same as index = index – 1. So it’s just a more concise way to decrement the index by 1.
Let’s test this program by calling our function:
print(reversed_word("Python"))
[output]
nohtyP
It works fine!
What Does the Python reversed() Function Do?
Python also provides a built-in function called reversed().
What does it do? Can we use it to reverse the characters in a string?
According to the official Python documentation, the reversed() function returns a reverse iterator.
But, how can it help?

>>> print(reversed("Greece"))
<reversed object at 0x7ff8e0172610>
Here is what we get if we cast the reverse iterator to a list.
>>> print(list(reversed("Greece")))
['e', 'c', 'e', 'e', 'r', 'G']
We get back a Python list in which every element is a character of the initial string but in reversed order. To obtain the reversed string we also have to use the string join() function.
>>> print(''.join(reversed("Greece")))
eceerG
We are using an empty separator character to concatenate the characters returned by the reverse iterator.
We could also cast the iterator to a list before passing it to the join function and the result would be the same.
>>> print(''.join(list(reversed("Greece"))))
eceerG
We have seen how to reverse a string in Python using just one line of code.
In one of the final sections of this tutorial, we will analyze which approach is the most performant.
Reverse String in Python Using Recursion
In this section, we will create a Python function that uses recursion to reverse a word / string.
This is a more complex implementation you will probably never use, but understanding it can add something to your Python developer knowledge.
def reverse_string_recursively(word):
if len(word) == 0:
return word
else:
return reverse_string_recursively(word[1:]) + word[0]
Let’s break down this code to understand how it works.
Call the function as shown below:
print(reverse_string_recursively('Hello'))
The first time it’s executed, the return statement in the else branch is equal to:
reverse_string_recursively('ello') + 'H'
This expression is equal to:
reverse_string_recursively('llo') + 'e' + 'H'
And this continues until the string passed to the function is empty:
reverse_string_recursively('lo') + 'l' + 'e' + 'H'
reverse_string_recursively('o') + 'l' + 'l' + 'e' + 'H'
reverse_string_recursively('') + 'o' + 'l' + 'l' + 'e' + 'H'
When the length of the string passed to the function is zero the function returns the string itself (see the logic in the if branch), so the final result is:
'' + 'o' + 'l' + 'l' + 'e' + 'H'
Here is what you get if you execute the expression above in the Python shell:
>>> '' + 'o' + 'l' + 'l' + 'e' + 'H'
'olleH'
The + sign is the Python string concatenation operator.
How to Reverse a Unicode String
Unicode is a standard used to represent characters in any language (and not only, with Unicode you can even represent emojis).
You might want to use Unicode if you have to handle strings in languages other than English.
For example, the following word means ‘Good morning’ in Greek:
Καλημερα
Let’s print each letter by using their Unicode representation:
>>> print('\U0000039A')
Κ
>>> print('\U000003B1')
α
>>> print('\U000003BB')
λ
>>> print('\U000003B7')
η
>>> print('\U000003BC')
μ
>>> print('\U000003B5')
ε
>>> print('\U000003C1')
ρ
>>> print('\U000003B1')
α
And now let’s print the full word still using the Unicode representation for each character:
>>> word = '\U0000039A\U000003B1\U000003BB\U000003B7\U000003BC\U000003B5\U000003C1\U000003B1'
>>> print(word)
Καλημερα
We can reverse the string by using the first approach we have covered in this tutorial (the slice operator).
>>> print(word[::-1])
αρεμηλαΚ
Performance Comparison of Approaches to Reverse a Python String
Let’s confirm which one of the approaches to reverse the characters of a string is the fastest.
To measure the performance of each implementation we will use Python’s timeit module.
Reverse a string using the slice operator
import timeit
testfunction = '''
def reversed_word():
return 'hello'[::-1]
'''
print(timeit.timeit(testfunction))
[output]
0.054680042
Reverse a string using a while loop
import timeit
testfunction = '''
def reversed_word():
word = 'hello'
reversed_word = ''
index = len(word) - 1
while index >= 0:
reversed_word += word[index]
index = index - 1
return reversed_word
'''
print(timeit.timeit(testfunction))
[output]
0.063328583
Reverse a string using the join() and reversed() functions
import timeit
testfunction = '''
def reversed_word():
word = 'hello'
return ''.join(reversed(word))
'''
print(timeit.timeit(testfunction))
[output]
0.062542167
Reverse a string using the join(), list() and reversed() functions
import timeit
testfunction = '''
def reversed_word():
word = 'hello'
return ''.join(list(reversed(word)))
'''
print(timeit.timeit(testfunction))
[output]
0.059792666999999994
The fastest implementation is the one using the slice operator.
Conclusion
In this tutorial, we have seen multiple ways to reverse a Python string.
The most performant way to reverse a string uses slicing but it’s also possible to use the reversed() function together with the join() and list() functions.
Implementing your own function to reverse a string using a loop doesn’t really make sense considering that it wouldn’t be as performant as the slice operator and it would require more lines of code.
Bonus read: Are you getting started with Python? Have a look at this tutorial that will give you a comprehensive overview of what it means to start coding in Python.
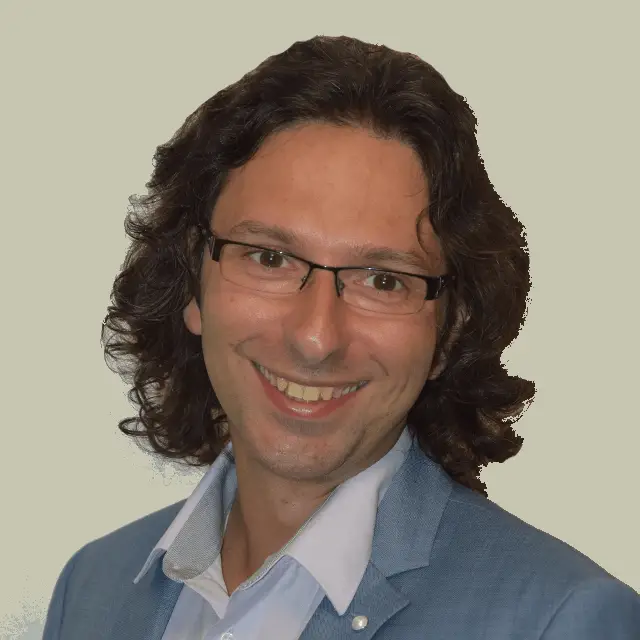
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.