Have you seen the Python AttributeError while writing your program and don’t know how to fix it? This tutorial will answer that.
If you are getting started with Python you might have experience seeing lots of different Python errors. The Python AttributeError is a very common type of error.
The Python AttributeError is a type of exception raised when your Python program tries to access an attribute that doesn’t exist on a variable/object. The description of the attribute error tells you the specific line of code, type of the variable, and attribute causing the error. Sometimes the error message also suggests a potential fix.
Right now you might not know what to do with this error if you are new to the Python programming language.
After going through this tutorial you will know how to fix the AttributeError in your Python code.
Let’s learn more about the AttributeError in Python!
What Is a Python AttributeError?
A Python AttributeError occurs when you try to use an attribute on a variable of a type that doesn’t support that attribute. For example, you try to call a method on a string and that method is not supported by the string data type. A method represents a functionality provided by a given Python data type or object (e.g. a string, int, boolean).
And is there a generic approach to troubleshooting and fixing a Python AttributeError?
To fix a Python AttributeError in your program you have to identify the variable causing the error. The traceback of the error helps you identify the root cause of this type of error because it includes the line of code where the error occurs. The traceback also tells you the name of the invalid attribute used in your program.
To make this simple, a Python AttributeError occurs when:
- You call an attribute of a variable (using the dot notation).
- That attribute does not exist on that variable/object.
Let’s go through two examples that will show you why the AttributeError is raised and the steps you can take to fix it.
How to Fix AttributeError in Python: ‘int’ object has no attribute
Let’s see an example of an attribute error when working with an integer in Python.
We will create an integer variable and then try to sum another integer to it using an attribute not supported by the int data type.
number = 5
total = number.add(4)
print("The total is", total)
Try to execute this code, you will see the following AttributeError exception:
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/attribute_error.py", line 2, in <module>
total = number.add(4)
AttributeError: 'int' object has no attribute 'add'
The attribute error says that an “int” object (in other words an integer) has not attribute “add”. This means that the .add() method (a method is a type of function) is not provided by an integer in Python.
The traceback is telling you that:
- The error occurred on line 2 of the code (it even shows you the content of that line).
- This is caused by the attribute “add” called on an “int” variable (object).
With these two pieces of information, you can immediately see that the variable to focus on is number and the code causing the error is number.add(4).
So, how can we fix this error?
Simply remove .add(4) and use the plus sign to calculate the sum of the two numbers. We will be using the plus operator that is supported by a variable of type integer.
Here is the correct code:
number = 5
total = number + 4
print("The total is", total)
[output]
The total is 9
You can see that this time there is no attribute error and the result is correct.
How to Fix AttributeError in Python: ‘str’ object has no attribute
Let’s have a look at another example of AttributeError raised with a Python string.
Imagine you want to convert every single character in a message (string data type) to upper case. You know that there is a way to do it in Python but you don’t remember exactly how.
So you try to use the dot notation followed by to_upper().
message = "Let's cause an attribute error in Python"
print(message.to_upper())
Here is the error you get.
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/tempCodeRunnerFile.py", line 2, in <module>
print(message.to_upper())
AttributeError: 'str' object has no attribute 'to_upper'. Did you mean: 'isupper'?
The Python interpreter raises an AttributeError because a “str” object (in other words, a string) doesn’t have the attribute “to_upper”. Python is also suggesting that maybe we wanted to use “isupper” instead.
The error is also telling you that the line of code causing this error is line 2 and it shows you the code causing the error: “print(message.to_upper())”.
To fix this error you have to replace the method “to_upper()” with the correct method a Python string provides to convert a string to upper case.
How can you find the correct method?
One way is by typing the dot ( . ) after the variable name and by looking for the correct method in the list of methods suggested by your IDE (e.g. Visual Studio Code or PyCharm).
Another option is to look at the official Python documentation for string methods. If you don’t know what to look for you might be searching for “to uppercase” on that page. This will get you to the “str.upper()” section of that page.

Let’s update our code and use the upper() method instead of to_upper().
message = "Let's cause an attribute error in Python"
print(message.upper())
[output]
LET'S CAUSE AN ATTRIBUTE ERROR IN PYTHON
The output is now correct and the initial message has been converted to uppercase.
How Do You Check Which Attributes Exist in a Python Object?
We have seen that you can use your IDE or Python’s official documentation to identify the correct attributes to use in your Python programs when working with a variable (or any Python object).
There is a quick way to identify all the attributes provided by a variable in your Python program. You can use Python’s dir() built-in function. Call this function and pass the variable to it. As a result, you will get the list of attributes available for that variable.
To see how this works, open the Python shell, create a string, and call the dir() function by passing the string variable to it.
>>> message = "test message"
>>> dir(message)
['__add__', '__class__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mod__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmod__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', 'capitalize', 'casefold', 'center', 'count', 'encode', 'endswith', 'expandtabs', 'find', 'format', 'format_map', 'index', 'isalnum', 'isalpha', 'isascii', 'isdecimal', 'isdigit', 'isidentifier', 'islower', 'isnumeric', 'isprintable', 'isspace', 'istitle', 'isupper', 'join', 'ljust', 'lower', 'lstrip', 'maketrans', 'partition', 'removeprefix', 'removesuffix', 'replace', 'rfind', 'rindex', 'rjust', 'rpartition', 'rsplit', 'rstrip', 'split', 'splitlines', 'startswith', 'strip', 'swapcase', 'title', 'translate', 'upper', 'zfill']
From the output of the dir() function you can also see the upper() method we have used previously in this tutorial to fix the AttributeError.
Attribute Error Tutorial Wrap Up
In this Python tutorial, we have seen what to do when the execution of your program fails due to an AttributeError.
Now you know:
- why this error/exception occurs: you are calling an attribute that is not supported by the variable/object you are calling it against.
- where to find more details about the AttributeError: the Python traceback tells you the line of code causing the error, the variable type, and the attribute called.
- how to fix the AttributeError: use your IDE, the dir() built-in function, or the official Python documentation to identify the correct attribute (method) to use.
Well done! Now you can fix the error you are seeing in your program and move forward with the development!
Related article: one of the things we have done in this tutorial is working with a Python string. Do you want to learn more about strings? Here is another CodeFatherTech tutorial that shows how to concatenate strings in Python.
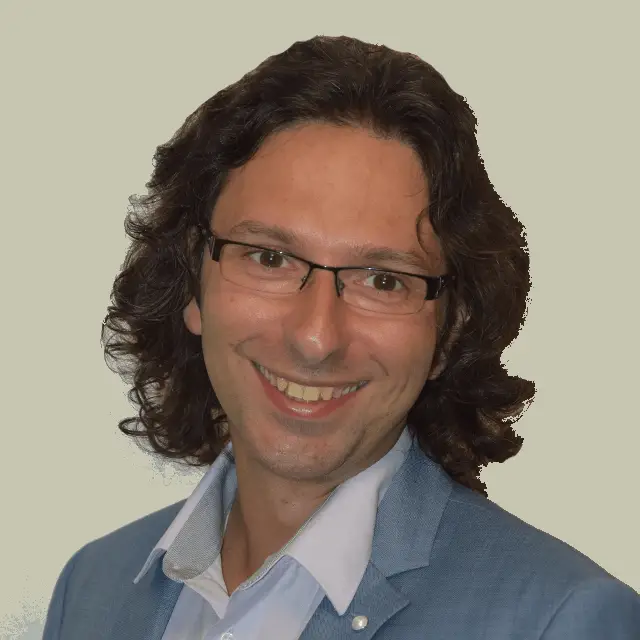
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.