While writing Python code you might have seen the “str object is not callable” error. What does it mean and how can you fix it?
Python raises the error ‘str’ object is not callable when on the line of code specified by the error you use a string variable like a function. You call a function by specifying parentheses after the function name and if you do the same with a string this causes the error ‘str’ object is not callable.
Once you understand why this error occurs it will be easier for you to debug and fix it. You will learn this skill through this tutorial.
Let’s fix this error!
What Does the Error “str object is not callable” Mean?
The error “str object is not callable” indicates that you are trying to use a string as a function in your Python code. In Python, you can define a function and then “call it”, in other words, a function is “callable” in Python. Data types like strings are not callable and that’s why this error occurs.
Let’s go through an example of Python code that shows this error.
message = "This is a string"
message()
In this code we have created the variable message and assigned a string to it.
To show how this error occurs we can specify the variable message followed by parentheses. In Python, you use parentheses to call a function.
In other words, we are trying to make a “call” to our string variable.
As you can see, the error “str object is not callable” is raised by Python:
Traceback (most recent call last):
File "/opt/codefathertech/tutorials/str_object_not_callable.py", line 2, in <module>
message()
TypeError: 'str' object is not callable
This code shows how strings don’t fall in the category of Python data types that can be “called”.
Why Does The Error “str object is not callable” Occur?
In the previous section, we have seen a simple example that explains when the “str object is not callable” occurs.
At the same time, it’s very unlikely for you to create a string and then purposefully call it as a function considering that this wouldn’t make logical sense.
Usually, this error occurs because, with the increase in complexity of your code, it can become harder to see that you are handling a string as a function.
Let’s analyze some other scenarios in which this error could happen.
Overriding a Python Built-In Function
Python provides a number of built-in functions that are widely used by developers.
If a developer is at the beginning of their Python learning, they might not be aware of the existence of a specific built-in function.
If the developer assigns a string to the name of a built-in function, this would cause the “str object is not callable” error later in the program when they use that built-in function.
Let me explain this with some code…
We will take as an example the filter() built-in function. This function applies a filter (represented by a function) to an iterable (e.g. a Python list).
The following line of code uses the filter() function to return numbers in a list that are lower than 3:
>>> list(filter(lambda x: x < 3, [1, 2, 3, 4]))
[1, 2]
Now, imagine that a few lines earlier in your Python code you have the following line:
filter = "This filter will select numbers lower than 3"
Now, if you try to call the filter() function here is what happens:
>>> list(filter(lambda x: x < 3, [1, 2, 3, 4]))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'str' object is not callable
The reason for this is that filter is not a function anymore but its value is now a string (the message we assigned to the variable before).
Shortly we will see what you can do when you encounter this type of error in your Python programs.
Using Parentheses Instead of Square Brackets
Here is another scenario that can cause the error “str object is not callable”…
Parentheses and square brackets can be confusing for a Python beginner developer. If you try to access a character in a string and instead of square brackets you use parentheses around the index of the character, this will cause Python to raise the error “str object is not callable”.
As an example, create the following string variable:
message = "This is a test message"
To get back the first character of the string you can use the syntax below:
>>> print(message[0])
T
This is what happens if by mistake you use parentheses instead of square brackets when retrieving the character:
>>> message(0)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'str' object is not callable
Python thinks that you are calling the string as a function. That’s why it raises the error ‘str’ object is not callable.
How to Fix the Error “str object is not callable”
Now that we have seen two scenarios in which this error can occur, let’s see what can you do to fix the error.
In the second scenario, you can replace the incorrect parentheses with square brackets. This might seem easy but it can get harder when you have hundreds of lines of code and also very long lines of code calling multiple functions in a single line (try to avoid that).
Let’s go through a process you can use to fix the error we have seen in the first scenario (overriding by mistake a built-in function).
Assume you are seeing the error we have previously explained:
>>> list(filter(lambda x: x < 3, [1, 2, 3, 4]))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'str' object is not callable
From the TypeError you receive it’s not straightforward to understand which variable in the code is a string.
So, let’s print the value of each variable from left to right and see what we get back:
>>> print(list)
<class 'list'>
By printing the value of list we can see that it’s an object of type list. This means it’s not a string, so this is not what the error is referring to.
Now let’s print the value of filter:
>>> print(filter)
This filter will select numbers lower than 3
The fact that we see a message in the value of filter already tells us that this might be the string our program is complaining about.
You can also use Python’s type built-in function to confirm that filter is a string:
>>> print(type(filter))
<class 'str'>
Ok, but now that you know that filter is a string and not a function as it should be, what can you do?
Simply rename the variable you used before for the message from filter to something else in your code, for example, filter_info.
filter_info = "This filter will select numbers lower than 3"
Now you have a way to fix this error.
Also, remember that the more you learn about Python programming the less likely is for you to encounter this type of error.
That’s because you will be able to remember quickly if a variable name is already used for a built-in function.
Conclusion
Knowing Python’s basic syntax and built-in functions is important to avoid errors while writing code.
As we have seen in this tutorial, the error ‘str’ object is not callable usually happens because of mistakes in the code due to not fully understanding Python data types and built-in functions.
The good news is that even if this error occurs you can always find the reason why through debugging (e.g. simply by using print statements) and then fix the error.
Related article: In this tutorial, we have seen that Python functions are “callable” because you can call them in your code. Dig deeper into what functions are and how they can help you as a programmer by going through the CodeFatherTech step-by-step tutorial about Python functions.
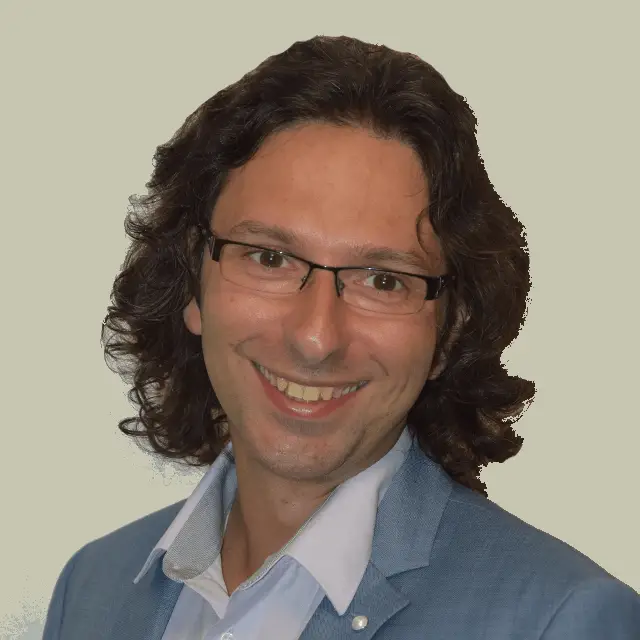
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.