Python is a programming language that is both versatile and powerful. Knowing how to iterate through a dictionary in Python is essential for every Python developer.
The dictionary data type, which is a collection of key-value pairs, can be iterated over to change and extract information. Python dictionaries provide multiple methods to iterate through their items, keys, and values.
In this article, we will look at numerous iteration strategies to access the dictionary data structure in Python.
Let’s get started!
Can You Iterate Through a Dictionary in Python?
In Python, you can iterate through a dictionary using various techniques. Whether you need to access keys, values, or both, you can use a Python for loop to iterate over dictionaries.
The for loop is an easy way to access data in a dictionary using a very simple syntax.
Let’s look at the first way we can use a for loop to iterate through dictionary keys and values.
How to Iterate Dictionary Keys and Values Using a Python For Loop
A Python dictionary is a data structure that stores a collection of key-value pairs. Every key in the dictionary is unique and corresponds to a specific value. A for loop can be used to iterate or cycle over the entries (keys and values) in a dictionary.
Consider the following scenario, in which students and their respective grades are represented using a dictionary:
students = {
'John': 85,
'Emily': 92,
'Michael': 78,
'Sarah': 89
}
for student in students:
print(student, students[student])
Here is the output of this Python code:
John 85
Emily 92
Michael 78
Sarah 89
In the code snippet above, we have a dictionary named students that stores the names of students as keys and their respective grades as values. These are also called key-value pairs.
To loop through the dictionary and print each student’s name along with their grade, we use a for loop.
The loop iterates over the keys of the dictionary, which are the student names. In each iteration, it prints the student’s name followed by their grade.
By using the notation students[student], you can retrieve the grade associated with each student in the loop. This allows you to access and process keys and values of the dictionary within the loop.
Iterating with a Python loop through a dictionary is the simplest approach!
Iterate Over Dictionary Items in Python Using dict.items() to Get Each Key-Value Pair
In Python, the items() method is a built-in functionality for dictionaries. The key-value pairs from the dictionary are included in the object returned by the items() dictionary method. You can use this object to loop through a dictionary and access all its keys and values.
Here’s an example:
students = {
'John': 85,
'Emily': 92,
'Michael': 78,
'Sarah': 89
}
for name, grade in students.items():
print(name, grade)
Output:
John 85
Emily 92
Michael 78
Sarah 89
In the code above, we have the same dictionary named students that stores the names of students as keys and their respective grades as values.
This time, instead of iterating only over the keys of the dictionary, we use the items() method to iterate over keys and values at the same time. The items() method returns an object that contains tuples of key-value pairs from the dictionary.
The loop iterates through each key-value pair in the dictionary, and for each iteration, it prints the student’s name (name variable in the for loop) followed by their grade (grade variable).
Below you can see the object returned by the items() method:
print(students.items())
[output]
dict_items([('John', 85), ('Emily', 92), ('Michael', 78), ('Sarah', 89)])
Let’s use the type() built-in function to confirm that this object contains Python tuples:
for item in students.items():
print(type(item))
[output]
<class 'tuple'>
<class 'tuple'>
<class 'tuple'>
<class 'tuple'>
By using the students.items() notation, we can access the keys and values of the dictionary at the same time within the loop.
Loop Through a Dictionary Using dict.keys() and dict.values()
For scenarios where we only need to iterate through the keys or values individually, we can use the keys() or values() methods respectively.
students = {
'John': 85,
'Emily': 92,
'Michael': 78,
'Sarah': 89
}
# Iterating through keys
for name in students.keys():
print(name)
# Iterating through values
for grade in students.values():
print(grade)
Here is the output in which you can see the dictionary keys followed by its values:
John
Emily
Michael
Sarah
85
92
78
89
In this code snippet, we demonstrate how to iterate only through the keys of the dictionary and how to iterate only through the values.
To iterate through the keys of a dictionary, you can use the keys() method. The keys() method returns an object that contains all the keys in the dictionary.
The loop iterates through each key in the dictionary, and for each iteration, it prints the key, which represents the student’s name.
Here is the object returned by the keys() method:
print(students.keys())
[output]
dict_keys(['John', 'Emily', 'Michael', 'Sarah'])
To iterate through the values of a dictionary, you can use the values() method. The values() method returns an object that contains all the values in the dictionary.
The loop iterates through each value in the dictionary, and for each iteration, it prints the value, which represents the student’s grade.
Let’s have a look at the object returned by the values() method:
print(students.values())
[output]
dict_values([85, 92, 78, 89])
By using the keys() method, you can iterate through the keys of the dictionary, and by using the values() method, you can iterate through the values. This allows accessing and processing the keys or values separately, depending on your specific needs.
What is the Most Efficient Method to Iterate Over a Dictionary in Python?
When it comes to performance, it is crucial to measure and compare the execution time of different methods for iterating over a dictionary.
Let’s demonstrate the timing measurements for each approach using a dictionary that contains 500,000 items.
import time
numbers = {str(i): i for i in range(500000)}
# Using a for loop to iterate through keys
start_time = time.time()
for key in numbers:
pass
end_time = time.time()
for_loop_time = end_time - start_time
# Using the items() method to iterate through keys and values
start_time = time.time()
for key, value in numbers.items():
pass
end_time = time.time()
items_method_time = end_time - start_time
# Using the keys() method to iterate through keys
start_time = time.time()
for key in numbers.keys():
pass
end_time = time.time()
keys_method_time = end_time - start_time
# Using the values() method to iterate through values
start_time = time.time()
for value in numbers.values():
pass
end_time = time.time()
values_method_time = end_time - start_time
print("Execution time for each method:")
print("for loop:", for_loop_time)
print("items() method:", items_method_time)
print("keys() method:", keys_method_time)
print("values() method:", values_method_time)
Let’s compare the execution time of each approach:
Execution time for each method:
for loop: 0.007589817047119141
items() method: 0.013831138610839844
keys() method: 0.007203817367553711
values() method: 0.006747245788574219
The code above measures the execution time for each iteration technique separately.
We first create a dictionary called numbers with 500,000 items, where the keys are strings representing numbers and the values are the corresponding numbers (integers).
To create this dictionary we use a dictionary comprehension (see the second line of the code immediately after the import statement).
Based on these measurements, we can conclude that the values() method tends to be the fastest approach for iterating through a dictionary.
After running this test multiple times you will notice that sometimes the keys() method is faster than the values() one.
However, it’s important to note that the performance difference between these methods is generally negligible for dictionaries of this size. The choice of iteration technique should be based on your specific requirements and the readability of your code.
Conclusion
To summarise, knowing how to iterate a dictionary is critical in Python. We investigated three approaches that use different dictionary methods:
- a for loop
- the items() method
- the keys() and values() methods
The ideal solution depends on the use case and data structure, but in general, the items() method strikes a balance between speed and simplicity of use.
Understanding these strategies allows for more efficient data extraction and processing.
Now it’s time to apply these approaches to your project and see which one you prefer!
Related article: now that you know how to iterate through Python dictionaries, have a look at how to work with Python nested dictionaries.
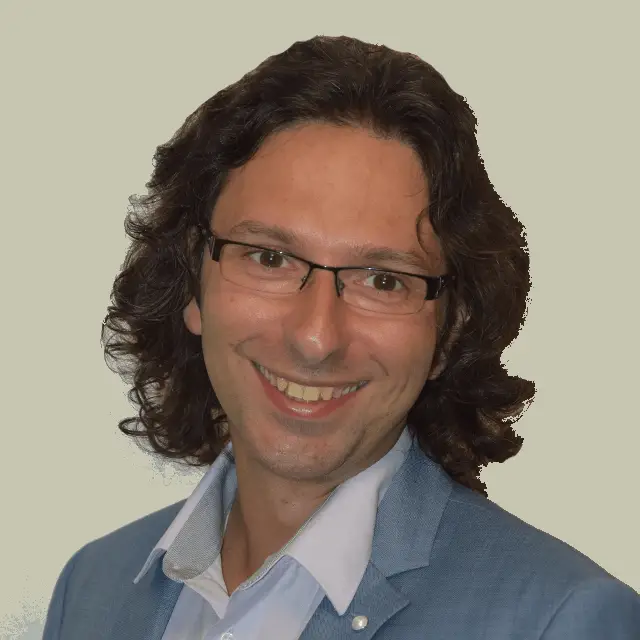
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.