Are you looking for a complex data structure for your application? Learn to create a nested dictionary in Python.
Using a nested dictionary in Python is a way to create a hierarchical data structure for your application. In a nested dictionary, the values mapped to keys are also dictionaries. You can build a nested dictionary with as many levels of nesting as you need.
Let’s explore how to create and use this data type!
What is a Nested Dictionary in Python?
A Python nested dictionary is a dictionary that contains one or more dictionaries as values. This is a data structure that allows storing data hierarchically.
How can you create a dictionary with a nested structure in Python?
Below you can see what a nested dictionary looks like:
data = {
'key1': {'inner_key1': 'inner_value1'},
'key2': {'inner_key2': 'inner_value2'}
}
The data dictionary has two keys: key1 and key2. The values associated with key1 and key2 are also dictionaries. That’s why we call this a nested dictionary. We have a dictionary inside a dictionary.
In this example, we have used the names key1 and inner_key1 to distinguish the keys of the outer dictionary from the keys of the inner dictionary.
How to Access Items in a Nested Dictionary with Python
To access a nested dictionary you use the inner and outer dictionary keys sequentially following the order in which they appear in the nested dictionary.
For example, to access the value inner_value2 in the previous dictionary you can use the following syntax:
print(data['key2']['inner_key2'])
[output]
inner_value2
As you can see, we have specified both keys within square brackets in the order in which they appear in the nested dictionary.
How to Access Items in Nested Dictionaries 3 Levels Deep
Let’s see how a nested dictionary works if we go one level deeper.
We will define a nested dictionary 3 levels deep:
data = {
'key1': {'inner_key1': {'inner_inner_key1': 'inner_value1'}},
'key2': {'inner_key2': {'inner_inner_key2': 'inner_value2'}}
}
If we access the value of inner_key1 we get a dictionary:
print(data['key2']['inner_key2'])
print(type(data['key2']['inner_key2']))
[output]
{'inner_inner_key2': 'inner_value2'}
<class 'dict'>
To access inner_value2 we have to add the other inner key to the chain of keys we have specified:
print(data['key2']['inner_key2']['inner_inner_key2'])
[output]
inner_value2
The logic is the same for any level of nesting you need in your data structure.
Adding Elements to a Nested Dictionary in Python
To add elements to a nested dictionary you have to specify the dictionary name followed by the key in square brackets and then assign a dictionary to it.
Let’s add an element to the initial 2-level nested dictionary and set the value of the key to key3.
Below you can see the initial dictionary:
data = {
'key1': {'inner_key1': 'inner_value1'},
'key2': {'inner_key2': 'inner_value2'}
}
Assign a new dictionary to key3:
data['key3'] = {'inner_key3': 'inner_value3'}
And print the updated value of the nested dictionary:
print(data)
[output]
{'key1': {'inner_key1': 'inner_value1'}, 'key2': {'inner_key2': 'inner_value2'}, 'key3': {'inner_key3': 'inner_value3'}}
To print the dictionary in a more readable way you can use Python’s pprint module.
import pprint
pprint.pprint(data)
[output]
{'key1': {'inner_key1': 'inner_value1'},
'key2': {'inner_key2': 'inner_value2'},
'key3': {'inner_key3': 'inner_value3'}}
It definitely looks better!
How To Update an Element in a Nested Dictionary
To update an element in a nested dictionary you have to chain the keys that identify the value in the nested dictionary and then assign the new value to it.
As an example, we will update the value of inner_value3 to the value updated_inner_value3.
We start with the following dictionary:
{'key1': {'inner_key1': 'inner_value1'},
'key2': {'inner_key2': 'inner_value2'},
'key3': {'inner_key3': 'inner_value3'}}
The following line of code updates the value for inner_value3 by accessing the value identified by key3 and inner_key3.
import pprint
data['key3']['inner_key3'] = 'updated_inner_value3'
pprint.pprint(data)
[output]
{'key1': {'inner_key1': 'inner_value1'},
'key2': {'inner_key2': 'inner_value2'},
'key3': {'inner_key3': 'updated_inner_value3'}}
From the output, you can see that we have updated the value inner_value3 to updated_inner_value3.
Deleting an Element From a Python Nested Dictionary
To delete an element from a nested dictionary you can use the del keyword in Python. The sequence of keys you will pass as part of the del statement determines which part of the nested dictionary you delete.
For example, let’s start with the following dictionary:
data = {
'key1': {'inner_key1': 'inner_value1'},
'key2': {'inner_key2': 'inner_value2'}
}
To delete the key-value pair inner_key2 : inner_value2 you can use the following Python statement:
del data['key2']['inner_key2']
Now let’s print the dictionary:
pprint.pprint(data)
[output]
{'key1': {'inner_key1': 'inner_value1'}, 'key2': {}}
You can see that we have deleted the key-value pair that represented the value of the dictionary key key2. That’s why the value of key2 is now an empty dictionary.
Let’s delete key2 and the associated value.
del data['key2']
pprint.pprint(data)
[output]
{'key1': {'inner_key1': 'inner_value1'}}
Now the only key left is key1 and its associated dictionary.
How Do You Iterate Through a Nested Dictionary?
Given a nested dictionary, how can you loop through it using a Python for loop?
First of all, open a Python shell and define a nested dictionary.
>>> data = {
... 'key1': {'inner_key1': 'inner_value1'},
... 'key2': {'inner_key2': 'inner_value2'}
... }
Now use the dir() function to see the methods provided by this dictionary.
>>> dir(data)
['__class__', '__class_getitem__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getstate__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__ior__', '__iter__', '__le__', '__len__', '__lt__', '__ne__', '__new__', '__or__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__ror__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'clear', 'copy', 'fromkeys', 'get', 'items', 'keys', 'pop', 'popitem', 'setdefault', 'update', 'values']
One of the methods provided by dictionaries is items().
Let’s see what this method returns in the Python shell.
>>> data.items()
dict_items([('key1', {'inner_key1': 'inner_value1'}), ('key2', {'inner_key2': 'inner_value2'})])
Here is what happens if we use a for loop to go through the elements in the object returned by the items() method.
>>> for x, y in data.items():
... print("This is x: ", x)
... print("This is y: ", y)
...
This is x: key1
This is y: {'inner_key1': 'inner_value1'}
This is x: key2
This is y: {'inner_key2': 'inner_value2'}
As you can see, at each iteration of the for loop we get back the outer key of the nested dictionary and the inner dictionary.
Using the same logic, we can use a nested for loop to get the key-value pairs of the inner dictionaries.
Let’s write the full Python code to go through all the keys and values of the nested dictionary.
for outer_key, inner_dict in data.items():
print(outer_key)
for inner_key, inner_value in inner_dict.items():
print(f"{inner_key} : {inner_value}")
And the output is:
key1
inner_key1 : inner_value1
key2
inner_key2 : inner_value2
This code allows you to go through the elements of a nested dictionary.
Should You Use a Nested Dictionary in Python?
There is nothing wrong with using a nested dictionary as a data structure for your Python programs.
At the same time, using a nested dictionary is not the only option available. For example, you could avoid a nested dictionary by using a dictionary in which the keys are tuples.
Here is what I mean. Take the following nested dictionary:
data = {
'key1': {'inner_key1': 'inner_value1'},
'key2': {'inner_key2': 'inner_value2'}
}
You could avoid the nested structure by using tuples as keys.
new_data = {
('key1', 'inner_key1'): 'inner_value1',
('key2', 'inner_key2'): 'inner_value2'
}
Let’s see how you would retrieve inner_value1 in both scenarios.
# Nested dictionary
print(data['key1']['inner_key1'])
# Dictionary with tuples as keys
print(new_data['key1', 'inner_key1'])
Verify that the output is inner_value1 in both cases.
Conclusion
In this Python tutorial, you learned how to work with Python nested dictionaries. From creating them to accessing their elements, from updating to deleting elements.
You have also seen how to iterate through a nested dictionary using a nested for loop.
Related article: in the last section of this article we have seen how you can use tuples in combination with a dictionary. Go through the CodeFatherTech tutorial about Python tuples to learn how to use them in your programs.
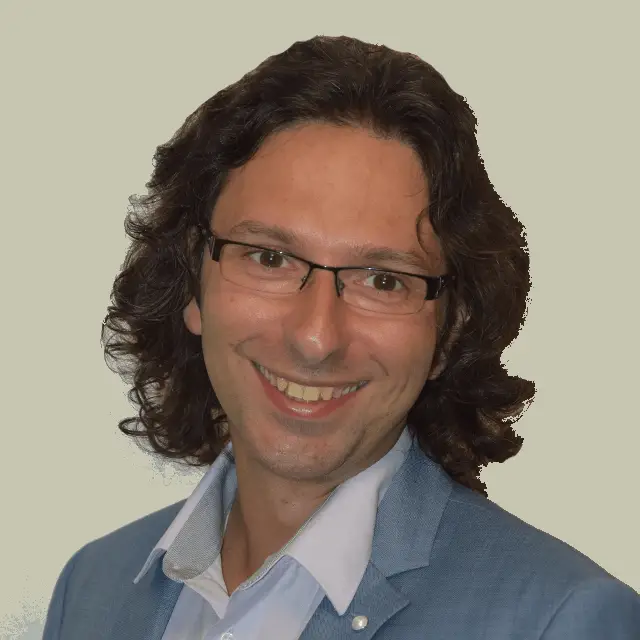
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.