I’m familiar with lists in Python and I’m aware that dictionaries are also available. What is the difference between lists and dictionaries? Which one should I use?
Lists are ordered Python data structures in which every element can be accessed using an index. Dictionaries are data structures in which each element is a key-value pair. Your choice between lists or dictionaries depends on the requirements you have for your Python application.
Let’s start with simple definitions of lists and dictionaries so you can have an idea of what they are.
Lists are collections of ordered elements that can be of multiple types. Lists are also mutable and iterable. You can use an index to access the elements in a list and the index value goes from 0 to n-1 where n is the number of elements in the list.
To create a Python list you use square brackets. List items are enclosed in square brackets and are comma-separated.
Below you can see an example of a list that has three elements of different types (float, string, and integer):
values = [4.5, 'hour', 23]
Here are the main features of lists:
- Ordered: a list maintains the order of its elements. This can be an important requirement in the logic of your application.
- Indexed: you can access the elements in a list by using an integer index that goes from 0 to n-1 (where n is the number of elements in the list).
- Mutable: you can modify the elements in a list after creating the list.
- Iterable: using a Python loop you can go through all the elements of a list. This can be extremely useful when you want to apply a specific logic to all the elements in a list.
Here is the definition of dictionaries:
Dictionaries are collections of key-value pairs. They are unordered, mutable, and iterable. A dictionary is delimited by curly brackets and key-value pairs are separated by commas. Dictionaries are also called hash tables or hashmaps in other programming languages.
Below you can see an example of a Python dictionary:
values = {'user1': 23, 'user2': 456, 'user3': 44}
Here are the main features of dictionaries:
- Unordered: a dictionary doesn’t maintain the order of its elements (key-value pairs). It is an unordered collection of objects.
- Indexed by keys: you can access elements in a dictionary using their unique keys.
- Mutable: you can add, modify, or remove key-value pairs in a dictionary.
- Iterable: you can iterate through Python dictionaries using a for loop.
When should you choose a Python list vs a dictionary?
Sometimes it might not be immediately clear which data type to choose between lists and dictionaries.
Let’s see some scenarios in which it makes sense to use lists:
- When the order of elements is important: if the order of the elements is important in the data structure you need for your application, then use a list. That’s because a list is an ordered sequence of objects.
- If you need numerical indexes: lists allow accessing their elements using a numerical index. So, if you have a requirement to access the elements in your data structure using a numerical index then lists are the right choice.
- When duplicate data is allowed: use lists if allowing duplicate values is a requirement in your application.
In which situation a dictionary is better than a list?
Let’s see a few reasons why you would use a dictionary as the data structure for your program.
- When looking up elements is important: the implementation of dictionaries uses key-value pairs. This provides a lookup of values based on their keys that is faster than a list. This makes dictionaries a lot more efficient to use than lists if performing lookups is one of your main requirements.
- If using key-value pairs makes logical sense: as we have seen, lists store elements as a sequence of values while dictionaries use key-value pairs. You might be developing an application in which using key-value pairs is the most sensible approach (e.g. a data structure that stores countries and their capitals).
- When duplicates are not allowed: keys in a dictionary must be unique. This property of Python dictionaries helps you if you want to make sure to avoid duplicate data in your application.
How do I access elements of a list or a dictionary?
To access an element in a Python list you use an index. To access an element in a dictionary you use a key that returns the value mapped to the key.
For example, let’s open the Python shell and define a list that contains three numbers:
>>> numbers = [3, 56, 789]
To access the second number in the list we will use the index 1 considering that the index of a list starts from zero.
>>> numbers[1]
56
Now, let’s create a dictionary where the keys are string representations of numbers and the values are integers.
>>> numbers = {'three': 3, 'fifty': 50, 'ten': 10}
To access the element identified by the key ‘fifty‘ you use the following syntax:
>>> numbers['fifty']
50
Do you see the different approaches we use to access list items or dictionary items?
Which one is better a Python list or a dictionary?
If we consider lists and dictionaries, one data structure is not necessarily better than the other. Using a list or a dictionary depends on what you need in your Python program.
A list is the right option if you want an ordered list of items that allows duplicates and you need to access it using an index. Remember that a dictionary is unordered.
Dictionaries are a better option than lists if you need data stored in key-value pairs and you are looking for fast lookups.
The decision to use a list or a dictionary depends on the specific requirements of your project.
If you notice that your code is becoming too complex consider the possibility that you might not be using the right data structure for that specific scenario.
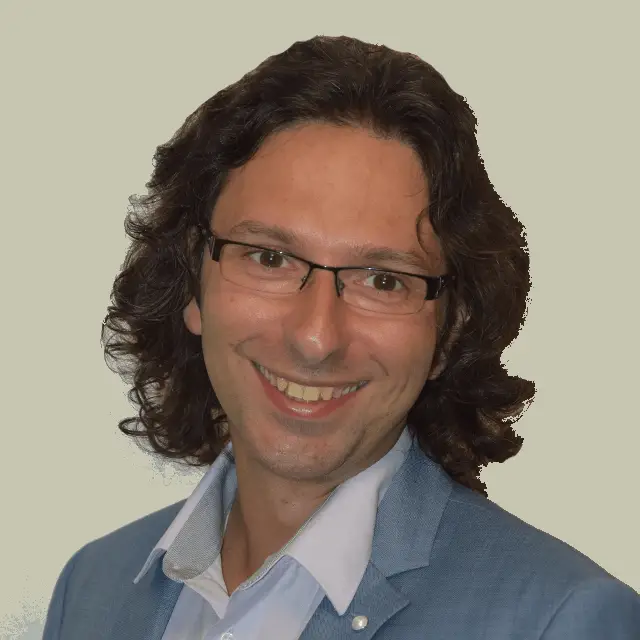
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.