If you are starting with Python programming, one of the first things you will learn to do is to create a list of numbers from 1 to N.
To create a list of numbers from 1 to N Python provides the range function. You can pass a “start” argument with value 1 and a “stop” argument with value N+1 to the range function and it generates a list of numbers from 1 to N after passing its output to the list() function. The behavior of the range function changes between Python 2 and Python 3.
Let’s go through some examples!
You can also learn 5 of the 10 ways in the video below:
Generate Numbers From 1 to N Using the Python range() Function
We will start with the simplest way to create a list of numbers from 1 to N in Python.
The first line of code below creates a Python list from the output returned when we call range() and pass the number N to it (10 in this case).
numbers = list(range(10))
print(numbers)
The output is a Python list with numbers from 0 to 9. That’s not exactly what we are looking for considering that we want to generate a list of numbers from 1 to N.
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
The range class allows passing two arguments, “start” and “stop”. Below you can see its syntax.
range(start, stop, step=1)
The “step” argument allows you to define the gap between numbers in the list generated. We won’t use it in this tutorial. We will focus on the “start” and “stop” arguments.
Let’s see what our code returns if the value of start is 1 and the value of stop is 11.
numbers = list(range(1,11))
print(numbers)
This time the output is what we are looking for.
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
To create a list of numbers from 1 to N in Python using the range() function you have to pass two arguments to range(): “start” equal to 1 and “stop” equal to N+1. Use the list() function to convert the output of the range() function into a list and obtain numbers in the specified range.
How Do You Print Numbers From 1 to N in Python Using a While Loop?
You can also use a while loop to print numbers from 1 to N. This is not the best approach considering that the one we have seen in the previous section is pretty simple and concise.
At the same time, as a developer, you should know how to implement programs in different ways because this gives you the knowledge to find the best solution to a given problem.
To print a list of numbers from 1 to N using a Python while loop you can set a numeric variable to 1 outside of the while loop. Then inside the loop, you print the variable and increase its value by 1 at every iteration. The condition of the while loop checks that the value of the numeric variable is less or equal to N.
number = 1
while number <= 10:
print(number)
number += 1
This is the output you get where N is equal to 10.
1
2
3
4
5
6
7
8
9
10
This time in the output you can see one number per line instead of a Python list that contains all the numbers.
If you want to get a Python list you have to add more code.
number = 1
numbers = []
while number <= 10:
numbers.append(number)
number += 1
print(numbers)
Execute the Python code above and confirm that you see the correct list:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
First, we create an empty list before the while loop. Then inside the loop, we call the append method of the list to add each number (element) to the list.
Finally, outside the while loop, we print the content of the list of numbers.
This approach with the while loop requires a few more lines of code compared to the approach using the range() function which only needs one line of code.
Create a List of Numbers from 1 to N in Python Using a For Loop
Are you wondering how to use Python’s for loop to print numbers from 1 to N?
Let’s find out!
Using the Python range function and a for loop you can print the numbers from 1 to N. The arguments to pass to the range class are start=1 and stop=N+1.
n=10
for number in range(1, n+1):
print(number)
Let’s verify the output:
1
2
3
4
5
6
7
8
9
10
That’s correct!
How to Print Numbers from 1 to N in Python Without Newline
In the previous example, you have seen numbers from 1 to 10 printed one per line.
How can you print numbers from 1 to N without a newline?
To print numbers from 1 to N in Python without the newline character on each line you can pass the optional “end” argument to the print() function. You can set the value of “end” to any character you want.
Here is how the output changes when we set end to the comma character.
n=10
for number in range(1, n+1):
print(number, end=',')
[output]
1,2,3,4,5,6,7,8,9,10,%
The percent character at the end of the numbers is there because of the missing newline character at the end of the line.
How can we change the for loop to still keep the comma between the numbers, remove the last comma and add a newline character at the end?
We can modify the for loop by adding an if statement inside it. We use the if statement to implement a different print logic for the last number in the list.
n=10
for number in range(1, n+1):
if number == 10:
print(number)
else:
print(number, end=',')
If the number is the last one in the list we use a standard call to the print() function without passing the “end” argument. This adds a newline character after the last number in the sequence.
Here is what our Python script prints:
1,2,3,4,5,6,7,8,9,10
Looks better!
Create a List of Numbers From 1 to N Using Using List Comprehensions
Another way to create a list of numbers from 1 to N is with Python list comprehensions. A list comprehension is a Python construct that generates a list.
This is a more advanced concept compared to what we covered so far and it’s something you should be aware of as a Python developer. List comprehensions are a very common construct used to write Pythonic code.
numbers = [number for number in range(1,11)]
print(numbers)
[output]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
We are still using range but this time we are using its output as part of a list comprehension.
I have written a separate article if you want to understand more about Python list comprehensions.
How to Generate Numbers from 1 to N with Python 2
If, for some reason, you find yourself using Python 2, here are the differences you will see when using the range() function.
Open the Python shell with Python 3 and then with Python 2. In both cases write the following code:
Python 3
>>> range(10)
range(0, 10)
>>> type(range(10))
<class 'range'>
Python 2
>>> range(10)
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> type(range(10))
<type 'list'>
Can you see the difference?
The type of object returned by range() is different between Python 2 and Python 3. Python 3 returns the iterable range(0, N) while Python 2 returns a list.
So, to print numbers from 1 to N (where N is equal to 10) with Python 2 you can simply use the following code:
numbers = range(1,11)
print(numbers)
[output]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Using Python 2, we didn’t have to pass the output of the range() function to the list() function.
Python Code to Print List of Numbers From 1 to 1000
At this point, you are an expert in printing numbers using Python 🙂
How do you think we can print the numbers from 1 to 1000 in Python?
We will use the simplest approach covered so far, we will use the list() and range() functions.
numbers = list(range(1,1001))
print(numbers)
To print the numbers from 1 to 1000 in Python you can use the range() function with the argument “start” set to 1 and the argument “stop” set to 1001. The list() function takes the output of range() and returns the list of numbers.
Given the length of the output, I will leave it to you to verify that you get the correct output.
Example of How to Print List from 0 to N Using Python
Once again, let’s use the range() function to print numbers from 0 to N (assume N is 50).
numbers = list(range(0,51))
print(numbers)
[output]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50]
To print numbers from 0 to N in Python you can return the output of range() to the list() function that then returns the list of numbers. The range() function takes as arguments “start” equal to 0 and “stop” equal to N+1. A simpler option is also to just pass “stop” equal to N+1 to the range() function.
Let’s see the simpler version of the code where we don’t pass the “start” argument to range().
numbers = list(range(51))
print(numbers)
Nice one, even simpler!
Create an Array of Numbers From 1 to N Using NumPy Module
So far, we didn’t have to import any external modules to implement our code.
In this section, we will see how to use the Python NumPy library to print numbers from 1 to N.
To create a sequence of integers using Numpy you can use the numpy.arange() function. To get a list of numbers from 1 to N pass two arguments to numpy.arange(): the “start” argument with value 1 and the “stop” argument with value N+1.
import numpy as np
n = 10
numbers = np.arange(1,n+1)
print(numbers)
On the first line, we have imported the NumPy module. Then we called the arange() function with “start” set to 1 and “stop” set to N+1.
The output is the following:
[ 1 2 3 4 5 6 7 8 9 10]
You can see that the output is different from the output you have seen previously when printing a list.
That’s because the output returned by numpy.arange() is a NumPy array instead of a list. You can confirm this by printing the output of the type() function on the object returned using numpy.arange().
print(type(numbers))
[output]
<class 'numpy.ndarray'>
How to Create a User-Defined Function That Returns Numbers From 1 to N
Once you have the logic of your Python code working, the next step is to create functions that allow you to reuse your code.
Let’s see how to write a function to create a list of numbers from 1 to N. We will use the approach based on the range() and list() functions.
To define a function in Python you have to use the def keyword, define the parameter of the function n, and use the same logic based on the range() and list() functions we have used previously.
Here is the Python function:
def get_numbers_from_1_to_n(n):
numbers = list(range(1,n+1))
return numbers
Let’s call the function to confirm it works. It should print numbers from 1 to 10.
print(get_numbers_from_1_to_n(10))
[output]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
The output is correct!
I have created a separate tutorial that explains Python functions in more depth if you want to get more familiar with them.
Summary of This Tutorial
In this Python tutorial, we have seen different ways to write a program to create a list of numbers from 1 to N.
When developing your Python applications you will have to implement one of the approaches we discussed, ideally the most Pythonic and concise one, for example, based on range().
At the same time, I hope you have found it helpful to see how to solve this problem in multiple ways.
Related article: if you are getting started with Python have a look at this step-by-step article about starting with Python coding.
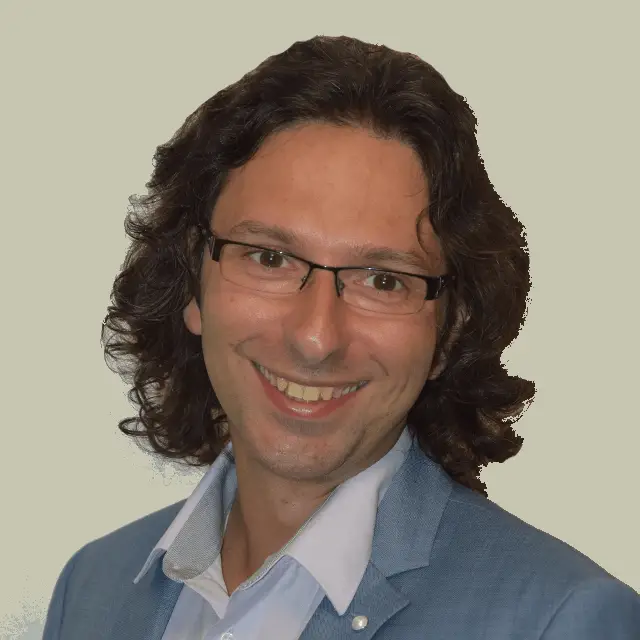
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.