Are you getting started with Python? Learning how to create a password generator can be the perfect project to learn or review basic Python concepts.
To create a password generator in Python you can use a for loop that randomly selects alphanumeric characters, digits, and punctuation characters to generate the password string. You can set the password length that defines the number of loop iterations. Also, by using a nested for loop you can improve the password generator to generate multiple passwords.
We will start with a simple password generator and then we will refactor the code to make it more flexible.
In the video below, I cover what we will go through in this tutorial (you can copy the code from this tutorial so you can try creating the password generator while going through it).
A Simple Password Generator in Python
We will start by generating a random string of 12 characters. Let’s use the function random.choice() that returns a random character from a sequence.
We will do the following:
- Import the random module.
- Set the password length to 12.
- Define a list of characters that we will use to generate the random password. In this first version of the program, we will use just a few letters and numbers.
- Create an empty string called password.
- Write a for loop that executes 12 iterations and that at every iteration selects a random character from the string characters and appends it to the password string.
- Print the password we have generated.
import random
password_length = 12
characters = "abcde12345"
password = ""
for index in range(password_length):
password = password + random.choice(characters)
print("Password generated: {}".format(password))
Here is an example of a password generated with this code:
Password generated: dcb4a2c4aac5
As you can see the password is not strong considering that we have used a limited number of characters and numbers.
In the next section, we will make it more secure.
How to Generate a Password Using All Alphanumeric Characters
Let’s improve the complexity of the password by using all alphanumeric characters.
To do that we could simply update the value of the characters string to include all letters and numbers but it would be time-consuming and prone to errors.
What can we do instead?
We can import the Python string module that provides a few constants that we can use in the generation of a password.
Here are a few examples:
>>> import string
>>> string.ascii_lowercase
'abcdefghijklmnopqrstuvwxyz'
>>> string.ascii_uppercase
'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
>>> string.ascii_letters
'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
>>> string.digits
'0123456789'
>>> string.punctuation
'!"#$%&\'()*+,-./:;<=>?@[\\]^_`{|}~'
We won’t use string.ascii_lowercase or string.ascii_uppercase considering that they are both included in string.ascii_letters.
In our password generator we will use the following three sets of characters:
- string.ascii_letters
- string.digits
- string.punctuation
We will use the + symbol to concatenate the three sets of characters to create a single string that we will assign to the characters variable.
This is what you get if you concatenate the three sets of characters in the Python shell:
>>> string.ascii_letters + string.digits + string.punctuation
'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!"#$%&\'()*+,-./:;<=>?@[\\]^_`{|}~'
Let’s update our program:
import random, string
password_length = 12
characters = string.ascii_letters + string.digits + string.punctuation
password = ""
for index in range(password_length):
password = password + random.choice(characters)
print("Password generated: {}".format(password))
Here are three examples of passwords generated with the updated program:
Password generated: iE%g.JqurkB0 Password generated: |>J+qbZ<Vl7$ Password generated: c94,JRgshz#g
Update the Password Generator to Receive the Password Length as User Input
Let’s make our password generator a bit more flexible.
We will allow the user to provide the password length instead of hardcoding it in our program. To do that we will use the input function.
import random, string
password_length = int(input("Provide the password length: "))
characters = string.ascii_letters + string.digits + string.punctuation
password = ""
for index in range(password_length):
password = password + random.choice(characters)
print("Password generated: {}".format(password))
Notice that we have converted the value returned by the input function into an integer considering that when using Python 3 the input function returns a string.
The program works fine.
Confirm it works on your machine too before continuing with this tutorial.
Provide the password length: 12 Password generated: ]"c_ga%M^iOd
If you don’t convert the output of the input function into an integer you get the following error when you use the variable password_length in the for loop.
Provide the password length: 12 Traceback (most recent call last): File "password_generator.py", line 9, in <module> for index in range(password_length): TypeError: 'str' object cannot be interpreted as an integer
How to Create a Python Password Generator that Generates Multiple Passwords
In this section, we will enhance our password generator to generate a custom number of passwords.
The approach will be very similar to the one we have used to get the password length from the user with the input function.
We will do the following:
- Get the
number_of_passwords
using the input function. - Add a for loop to generate multiple passwords based on the value of the variable number_of passwords provided by the user.
import random, string
number_of_passwords = int(input("How many passwords do you want to generate? "))
password_length = int(input("Provide the password length: "))
characters = string.ascii_letters + string.digits + string.punctuation
for password_index in range(number_of_passwords):
password = ""
for index in range(password_length):
password = password + random.choice(characters)
print("Password {} generated: {}".format(password_index, password))
Note: make sure you use the correct indentation as shown in the code above.
Let’s test our code:
How many passwords do you want to generate? 3 Provide the password length: 8 Password 0 generated: 2B.1&=~k Password 1 generated: Wt$@1vi' Password 2 generated: ,aOXN_@$
It works, nice!
How to Generate Strong Passwords in Python
Before completing this tutorial let’s find out how we can enforce a set of rules to make sure the passwords we generate are strong enough.
We will make sure passwords contain at least:
- three digits
- two punctuation characters
To do that we will use two integers that define the number of digits and punctuation characters. Then we will use multiple nested for loops.
import random, string
number_of_digits = 3
number_of_punctuation_characters = 2
characters = string.ascii_letters + string.digits + string.punctuation
number_of_passwords = int(input("How many passwords do you want to generate? "))
password_length = int(input("Provide the password length: "))
for password_index in range(number_of_passwords):
password = ""
for digits_index in range(number_of_digits):
password = password + random.choice(string.digits)
for punctuation_index in range(number_of_punctuation_characters):
password = password + random.choice(string.punctuation)
for index in range(password_length - number_of_digits - number_of_punctuation_characters):
password = password + random.choice(string.ascii_letters)
print("Password {} generated: {}".format(password_index, password))
Let’s have a look at a few passwords generated with this program:
How many passwords do you want to generate? 3 Provide the password length: 10 Password 0 generated: 738<>ZKwMA Password 1 generated: 332|(SlZDT Password 2 generated: 756%#NFWHs
They look fine but to make them stronger we have to avoid having digits and punctuation characters always in the same position of the password string.
To shuffle characters in the password string we will use the random.shuffle() function.
We will create a specific function that does the shuffling.
def randomize_password(password):
password_list = list(password)
random.shuffle(password_list)
return "".join(password_list)
This function converts the password string into a list before applying random.shuffle. Then it returns a string using the string join method.
Then update the last print statement to call the randomize_password function.
print("Password {} generated: {}".format(password_index, randomize_password(password)))
And the output is…
How many passwords do you want to generate? 3 Provide the password length: 10 Password 0 generated: X1+dT)4U1q Password 1 generated: 2T7g+OQ-B4 Password 2 generated: g3n0<3O>if
The password is a lot stronger now that digits and punctuation characters are in random positions.
Conclusion
In this tutorial, you have learned how to create a password generator in Python and how to update its logic to increase the strength of the passwords.
We have covered a few Python core concepts:
- importing modules.
- defining functions.
- using the input function to read user input.
- nested for loops.
- selecting random characters from a string and shuffling a string.
- using the string format method.
Well done for completing this tutorial!
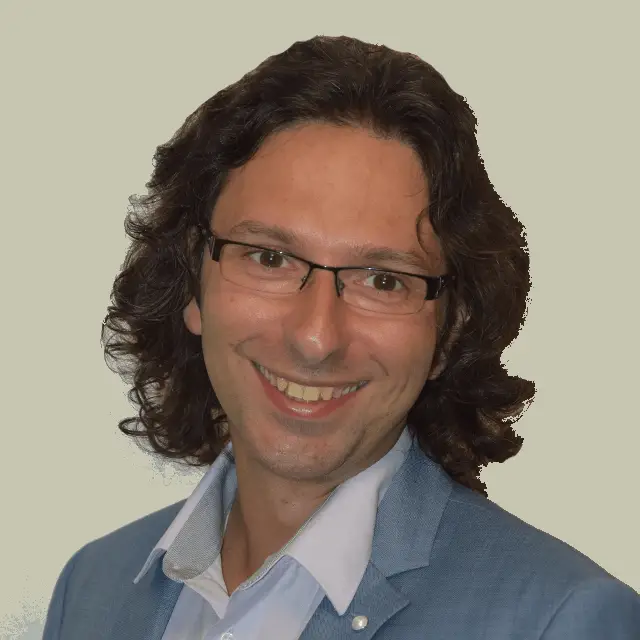
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
I really enjoyed this I am ver new to coding and this hands on step by step explanations is the perfect way to learn where can I find more like this? Thank you again, very good job.