If you are getting familiar with Python classes you might have heard the term instance method.
What are instance methods? How can you use them?
Instance methods are the default methods defined in Python classes. They are called instance methods because they can access instances of a class (objects). Two ways to use instance methods are to read or write instance attributes. In other words, instance methods are used to read or modify the state of an object.
We will go through a few examples to make sure you understand instance methods and you can use them in your Python programs.
Let’s get started!
How to Create an Instance Method in Python
The first step to create an instance method is to create a Python class using the class keyword.
The class keyword allows to define a new class in Python. By defining a new class you create a new data type.
Once you create a class you can define an instance method inside that class using a similar syntax you would use to define a Python function (using the def keyword).
We will create a class called Vehicle and an instance method part of it.
class Vehicle:
def __init__(self, type, color):
self.type = type
self.color = color
def get_description(self):
print("This vehicle is a {} {}".format(self.color, self.type))
Let’s go through the logic in the code above:
- Define a class called Vehicle.
- Add a class constructor (the __init__ method) that allows to create instances of Vehicle that have two attributes: type and color.
- Define an instance method called get_description().
An instance method always has self as first argument. The self argument represents the instance of the class on which the instance method is called.
As you can see from the implementation of the get_description() method, we use self to access the two attributes of the object: type and color.
And to print the message in the instance method we use the string format method.
Every method you define in a class is by default an instance method unless you tell the Python interpreter otherwise using specific decorators.
I will create a separate tutorial that explains how to define other types of methods like static methods and class methods in Python.
How to Call an Instance Method in Python
After defining a class and an instance method inside it we can call the instance method on an instance of that class.
First of all create an instance of the class Vehicle:
bus = Vehicle("bus", "blue")
To create a Vehicle object (or instance) we have passed values for the two attributes we have specified in the definition of the class: type and color.
Then use the instance name and the dot notation to call the instance method.
bus.get_description()
[output]
This vehicle is a blue bus
Notice that…
When you call an instance method you don’t pass the self parameter. Self is implicitly passed when you call the instance method.
So, the correct syntax to call an instance method is:
instance.instance_method(arg1, arg2, ..., argN)
Where arg1, arg2, …, argN are the arguments passed to the instance method. Self is excluded considering that as we said before doesn’t have to be passed.
What is the Value of self in Instance Methods?
I want to say more about the self argument of instance methods considering that it can be confusing if you are just getting started with object oriented Python.
Self represents an instance of a class (also called object) and we use it in two methods of the Vehicle class.
- __init__() method: this is the constructor that creates instances of type Vehicle. In the constructor self is used to set the values of the instance attributes type and color based on the values passed when the object is created. This means that in this case self is used to update the state of an object.
- get_description() method: this is an instance method and it uses self to read the values of the instance attributes type and color so it can print a message. In this case self is used to read the state of an object.
Before when creating the Vehicle object we have used the following code:
bus = Vehicle("bus", "blue")
In the same way we could create a second object:
car = Vehicle("car", "red")
car.get_description()
[output]
This vehicle is a red car
This shows that every object you create has its own independent state that is set when the object is created via the constructor.
To understand even more about self let’s add a print statement in the first line of our instance method.
def get_description(self):
print(self)
print("This vehicle is a {} {}".format(self.color, self.type))
When you execute the instance method against the car instance you get the following output:
<__main__.Vehicle object at 0x10430b3a0>
This vehicle is a red car
The print statement shows that self is an object of type Vehicle as we expected.
Can You Call an Instance Method From Another Instance Method?
Let’s find out if an instance method can call another instance method of the same class.
The instance method we have defined before just prints a message. Generally the purpose of instance methods is to allow objects to perform specific actions.
For example…
We could create an instance method that turns on the engine of our car (imagine if this object is part of a video game).
Let’s update our class to do the following:
- Track the status of the engine of our vehicle using a new boolean attribute called engine_on that by default is False.
- Define a new instance method called turn_engine_on() that prints the description of the vehicle, sets the value of the engine_on attribute to True and then prints a message.
class Vehicle:
def __init__(self, type, color):
self.type = type
self.color = color
self.engine_on = False
def turn_engine_on(self):
self.get_description()
self.engine_on = True
print("{} engine turned on".format(self.type.title()))
def get_description(self):
print("This vehicle is a {} {}".format(self.color, self.type))
Call the new instance method and confirm that the output is correct…
car = Vehicle("car", "red")
car.turn_engine_on()
[output]
This vehicle is a red car
Car engine turned on
As you can see the turn_engine_on() method calls the other instance method of the class using the following syntax:
self.get_description()
To call an instance method from another instance method in the same class use the following generic syntax:
self.instance_method(arg1, arg2, ..., argN)
Note: as you can see you don’t have to pass self when calling the other instance method.
Also, another thing to notice…
We have used the new instance method turn_engine_on() to update the value of the boolean instance attribute engine_on.
As explained before we have updated the state of our instance (or object).
Conclusion
You should now have enough knowledge to start using instance methods in your classes. And you should also have an understanding of how to use self in your code.
In this tutorial we have seen how to:
- Define instance methods.
- Call instance methods on a class instance to read or update its state.
- Call an instance method from another instance method in the same class.
Watch the video below to make sure you remember all the concepts we have covered in this tutorial:
And now it’s your time to use instance methods.
Happy object-oriented programming!
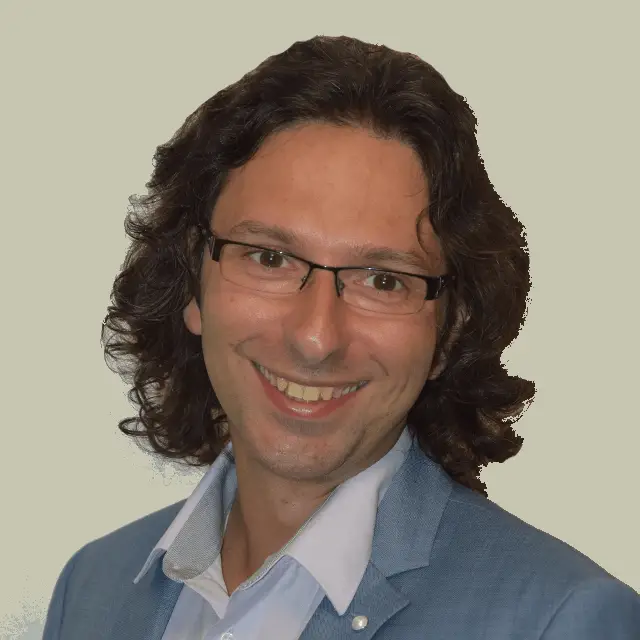
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
thks,
very clear the mode you exposed this topic.