When you start working with functions in Python you will likely ask yourself what is the difference between parameters and arguments.
This is a very common question and it often confuses those who are new to programming.
In Python when you define a function, you list parameters on the same line as the def keyword within parentheses. These are like placeholders for the values (arguments) that the function works with when it’s called. In other words, arguments are passed to a function call and give values to function parameters.
Let’s take the following code as an example of a function:
def calculate_sum(a, b):
result = a + b
return result
number1 = 10
number2 = 15
print("The sum of the two numbers is " + str(calculate_sum(number1, number2)))
Which ones are parameters and which ones are arguments?
- The parameters are
a
andb
. They are present in the definition of the function on the same line as thedef
keyword. Those are the input values that the function expects. - The arguments are
number1
andnumber2
. You pass them to the functioncalculate_sum()
when you call it. - The value of the argument
number1
is assigned to the parametera
and the value of the argumentnumber2
is assigned to the parameterb
.
In other words, arguments provide concrete values to the function parameters. This allows the function to perform its intended purpose with the data provided.
Standard function arguments are also called positional arguments because they have to follow the same order of the parameters specified in the definition of a function.
Number of Function Parameters and Arguments
When using positional arguments the number of arguments passed to a function has to match the number of parameters in the function definition.
Here is what happens if the numbers of arguments and parameters don’t match.
Passing more positional arguments than parameters
def calculate_sum(a, b):
result = a + b
return result
number1 = 10
number2 = 15
number3 = 20
calculate_sum(number1, number2, number3)
When passing more positional arguments than parameters, the TypeError raised by Python tells you that the function takes 2 positional arguments but 3 were provided.
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: calculate_sum() takes 2 positional arguments but 3 were given
Passing less positional arguments than parameters
calculate_sum(number1)
When passing less arguments than parameters, the TypeError
raised tells you that the function is missing one required positional argument with details about which argument is missing.
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: calculate_sum() missing 1 required positional argument: 'b'
Now you know how to troubleshoot this TypeError if you see it when calling a function.
Changing the Order of the Arguments Passed to a Function
The order of arguments in a function call may change the output returned by the function.
For instance, let’s take the function below:
def calculate_sum(a, b):
result = 2*a + b
return result
number1 = 10
number2 = 15
When you call the function in the following way the value number1
is assigned to the parameter a
and the value number2
is assigned to b
.
Call this function using the Python shell to verify the result returned by the function:
>>> calculate_sum(number1, number2)
35
If you switch the order of the arguments, the value number2
is assigned to a
and the value number1
is assigned to b
.
>>> calculate_sum(number2, number1)
40
You can see how switching the order of the arguments results in a different value returned by the function.
However, there is an alternative to positional arguments.
Python offers flexibility with keyword arguments that allow you to specify the names of the parameters when you call the function, enabling you to pass arguments in a different order.
The syntax you use when passing a keyword argument is the following:
parameter_name=argument_value
Let’s see how this works with the previous example of code:
>>> calculate_sum(a=number1, b=number2)
35
>>> calculate_sum(b=number2, a=number1)
35
As you can see, using keyword arguments, we make sure that the arguments are assigned to the correct parameters.
This feature is useful in functions that have many parameters, or when default values are involved. It improves code readability and reduces the chances of passing arguments in the wrong order.
Note: you can mix positional and keyword arguments when calling a function, but all keyword arguments must come after positional arguments.
Related article: If you are getting started with functions go through the CodeFatherTech tutorial that explains how to create a function in Python.
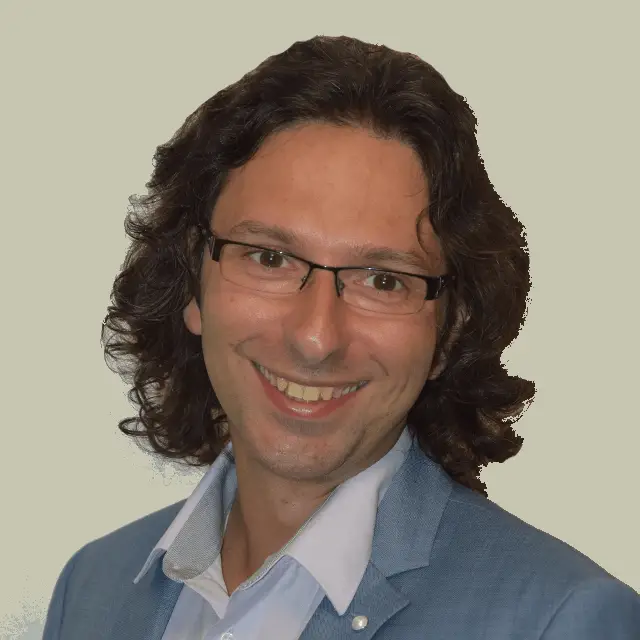
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.