When writing a Python program you might want to access multiple elements in a list. In this scenario, Python list slicing can be very useful.
With Python’s list slicing notation you can select a subset of a list, for example, the beginning of a list up to a specific element or the end of a list starting from a given element. The slicing notation allows specifying the start index, stop index, and interval between elements (step) to select.
Slicing is a topic that can be a bit confusing for Python beginners and in this article, I will help you understand slicing based on my personal experience of using it in several Python applications.
Let’s see some examples of list slicing!
What Is List Slicing in Python?
In Python, you can use the colon character( : ) within square brackets to print part of a list (this is called slicing).
The first step to using slicing with a Python list is to understand the syntax for slicing:
list_slice = original_list[start:stop:step]
The first important concept to know is that when you apply the slicing operator to a list you get back another list.
The syntax of slicing in Python supports the following arguments:
- start: the start index (inclusive)
- stop: the stop index (exclusive)
- step: the interval between elements returned in the slice
Based on my experience, one of the confusing aspects of slicing can be the fact that the start index is inclusive and the stop index is exclusive.
This is something you will have to remember and get used to to make sure you select the correct subset of a list.
6 Examples of List Slicing in Python
Let’s go through a few examples to explain how you can use the start, stop, and step arguments in the slicing notation.
Let’s take a list that contains the first 10 numbers of the Fibonacci sequence:
fibonacci_sequence = [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
In the following sections, you will see the lists returned by the slicing operator when using different combinations of the start, stop, and step arguments.
1. Slice with Start and Stop Arguments
In this example, we specify where the slice starts and stops.
sliced_list = fibonacci_sequence[2:5]
print(sliced_list)
[output]
[1, 2, 3]
Start is equal to 2 and stop is equal to 5. The result is a slice of the original list that goes from index 2 (inclusive) to index 5 (exclusive).
2. Slice with Start Argument Only
When you only specify the start argument, the slice goes from the start index to the end of the list.
sliced_list = fibonacci_sequence[3:]
print(sliced_list)
[output]
[2, 3, 5, 8, 13, 21, 34]
Considering that start is equal to 3 the slice goes from index 3 to the end of the original list.
3. Slice with Stop Argument Only
When you only specify the stop argument the slice goes from the beginning of the list to the stop index (exclusive).
sliced_list = fibonacci_sequence[:4]
print(sliced_list)
[output]
[0, 1, 1, 2]
Given that stop is equal to 4 the slice goes from the beginning of the original list to index 4 exclusive.
4. Slice with Start, Stop, and Step Arguments
You can combine the start, stop, and step arguments to have more control over the slice of the original list.
This is the first time we use the step argument in this tutorial, here is what it does:
sliced_list = fibonacci_sequence[1:8:2]
print(sliced_list)
[output]
[1, 2, 5, 13]
In this example, start is equal to 1, stop is equal to 8 and step is equal to 2. This means that the slice goes from index 1 (inclusive) to index 8 (exclusive).
The fact that step is 2 means that the slice includes every other element of the list within the limits defined by start and stop.
5. Slicing with Positive Step Argument Only (without Start and Stop)
Let’s see what happens if you only use a positive step value with the list slicing operator.
sliced_list = fibonacci_sequence[::2]
print(sliced_list)
[output]
[0, 1, 3, 8, 21]
Notice that the slice notation contains two colons followed by the value 2. That’s because there are no values for start/stop and the step is equal to 2.
The result is a slice that contains every other element in the original list.
6. Slice with Negative Step Argument Only
You can use an empty start, stop, and a negative value for the step argument (-1) to reverse the elements of a list.
sliced_list = fibonacci_sequence[::-1]
print(sliced_list)
[output]
[34, 21, 13, 8, 5, 3, 2, 1, 1, 0]
This is similar to the result you can get using Python’s built-in reversed() function.
Conclusion
In this tutorial, you have learned what list slicing is in Python and how you can use different combinations of start, stop, and step values to obtain different slices.
How are you using slicing in your Python programs? Let me know in the comments below!
Related article: go through the following CodeFatherTech tutorial to learn about methods provided by Python lists.
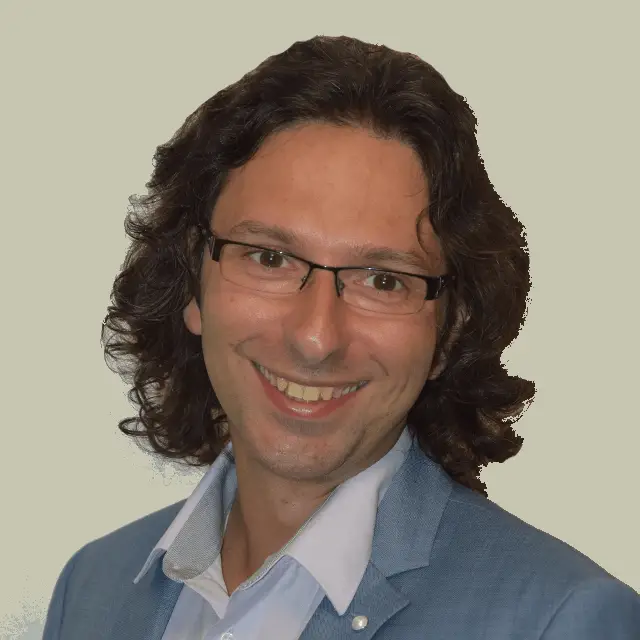
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
Thank you for sharing your knowledge!
Cheers!